How can you minimize the use of setData to improve performance?
Minimizing the use of setData
in applications, particularly in frameworks like Flutter, can significantly enhance performance. setData
is often used to update the state of widgets, which can lead to unnecessary rebuilds if not managed properly. Here are several strategies to minimize its use:
-
Batching Updates: Instead of calling
setData
multiple times in quick succession, batch the updates into a single call. This reduces the number of rebuilds, as the widget tree is only updated once after all changes are made. -
Using StatefulWidget Wisely: Ensure that
setData
is called only when necessary. Sometimes, developers might callsetData
even when the state hasn't changed, leading to unnecessary rebuilds. Always check if the new state is different from the current state before callingsetData
. -
Lifting State Up: If multiple widgets need to share the same state, consider lifting the state to a common ancestor. This way, you can minimize the number of
setData
calls by updating the state in one place, which then propagates down to all dependent widgets. -
Using InheritedWidget or Provider: These state management solutions can help manage state more efficiently. They allow widgets to listen to changes in state without the need for direct
setData
calls, reducing the frequency of rebuilds. -
Immutable Data Structures: Use immutable data structures to prevent unnecessary updates. When the data is immutable, you can more easily determine if a change has occurred, which helps in deciding whether to call
setData
.
By implementing these strategies, you can significantly reduce the number of setData
calls, thereby improving the performance of your application.
What alternative methods can be used instead of setData for better performance?
There are several alternative methods to setData
that can be used to improve performance in applications, especially in Flutter:
-
Provider: The Provider package is a state management solution that allows widgets to listen to changes in state without the need for direct
setData
calls. It uses theChangeNotifier
class to notify listeners when the state changes, which can be more efficient than callingsetData
directly. -
Bloc (Business Logic Component): The BLoC pattern separates the business logic from the UI, allowing for more controlled state updates. Instead of calling
setData
, you can useBlocProvider
andBlocBuilder
to manage and update the state. -
Riverpod: Riverpod is an evolution of Provider that offers more flexibility and control over state management. It allows you to create providers that can be used to manage state and notify widgets of changes without the need for
setData
. -
ValueNotifier:
ValueNotifier
is a simple way to notify listeners of changes to a value. It can be used in conjunction withValueListenableBuilder
to update widgets when the value changes, which can be more efficient than usingsetData
. -
InheritedWidget:
InheritedWidget
allows you to share data between widgets without having to pass it down through the widget tree. It can be used to manage state and notify widgets of changes, reducing the need forsetData
.
By using these alternative methods, you can achieve better performance by reducing the number of unnecessary rebuilds and improving the efficiency of state management.
How does reducing setData calls impact the overall efficiency of an application?
Reducing setData
calls can have a significant positive impact on the overall efficiency of an application, particularly in frameworks like Flutter. Here's how:
-
Reduced Rebuilds: Each call to
setData
triggers a rebuild of the widget tree. By reducing these calls, you minimize the number of rebuilds, which directly translates to improved performance, especially in complex UIs with many widgets. - Lower CPU Usage: Fewer rebuilds mean less work for the CPU, resulting in lower CPU usage. This is particularly important for mobile applications, where battery life and device performance are critical.
- Improved Responsiveness: With fewer rebuilds, the application becomes more responsive. Users will notice smoother interactions and quicker responses to their actions, enhancing the overall user experience.
- Memory Efficiency: Reducing unnecessary rebuilds can also lead to better memory management. When widgets are rebuilt less frequently, the application consumes less memory, which is crucial for devices with limited resources.
-
Better State Management: By reducing
setData
calls, you are often forced to implement more efficient state management techniques, such as using Provider or BLoC. These techniques not only reducesetData
calls but also improve the overall architecture of the application.
In summary, reducing setData
calls leads to a more efficient, responsive, and resource-friendly application, which is essential for delivering a high-quality user experience.
What specific techniques can be implemented to optimize data updates and enhance performance?
To optimize data updates and enhance performance, several specific techniques can be implemented:
-
Debouncing and Throttling: These techniques can be used to limit the frequency of
setData
calls. Debouncing ensures thatsetData
is only called after a certain period of inactivity, while throttling limits the number of calls within a given time frame. This is particularly useful for handling user input or network requests. -
Selective Rebuilding: Use
Selector
widgets from the Provider package to rebuild only the parts of the UI that depend on the changed data. This prevents unnecessary rebuilds of the entire widget tree. -
Immutable Data: Use immutable data structures to ensure that changes to data are more predictable and easier to manage. This can help in deciding when to call
setData
and reduce unnecessary updates. -
State Management Libraries: Implement state management libraries like Provider, Riverpod, or BLoC to manage state more efficiently. These libraries help in reducing the number of
setData
calls by providing more controlled and efficient ways to update the state. -
Caching: Implement caching mechanisms to store frequently accessed data. This can reduce the need for frequent data updates and
setData
calls, especially when dealing with network requests or expensive computations. -
Optimized List Building: When dealing with lists, use techniques like
ListView.builder
instead ofListView
to build list items on demand. This can significantly reduce the number of widgets that need to be rebuilt when the data changes. -
Asynchronous Updates: Use asynchronous programming to handle data updates without blocking the main thread. This can improve the responsiveness of the application and reduce the impact of
setData
calls on performance.
By implementing these techniques, you can optimize data updates, reduce the number of setData
calls, and enhance the overall performance of your application.
The above is the detailed content of How can you minimize the use of setData to improve performance?. For more information, please follow other related articles on the PHP Chinese website!
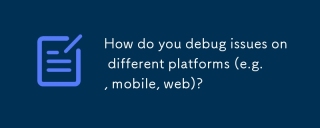
The article discusses debugging strategies for mobile and web platforms, highlighting tools like Android Studio, Xcode, and Chrome DevTools, and techniques for consistent results across OS and performance optimization.

The article discusses debugging tools and best practices for UniApp development, focusing on tools like HBuilderX, WeChat Developer Tools, and Chrome DevTools.

The article discusses end-to-end testing for UniApp applications across multiple platforms. It covers defining test scenarios, choosing tools like Appium and Cypress, setting up environments, writing and running tests, analyzing results, and integrat
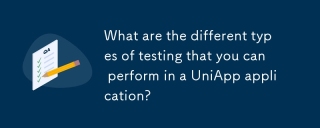
The article discusses various testing types for UniApp applications, including unit, integration, functional, UI/UX, performance, cross-platform, and security testing. It also covers ensuring cross-platform compatibility and recommends tools like Jes
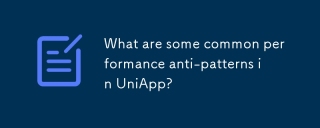
The article discusses common performance anti-patterns in UniApp development, such as excessive global data use and inefficient data binding, and offers strategies to identify and mitigate these issues for better app performance.
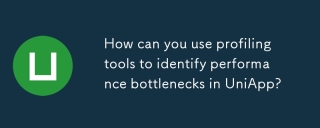
The article discusses using profiling tools to identify and resolve performance bottlenecks in UniApp, focusing on setup, data analysis, and optimization.
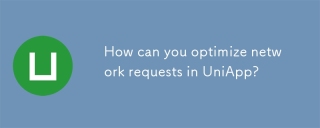
The article discusses strategies for optimizing network requests in UniApp, focusing on reducing latency, implementing caching, and using monitoring tools to enhance application performance.
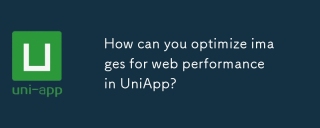
The article discusses optimizing images in UniApp for better web performance through compression, responsive design, lazy loading, caching, and using WebP format.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 Chinese version
Chinese version, very easy to use