


How do you register lifecycle hooks (e.g., onMounted, onUpdated, onUnmounted) in the Composition API?
In Vue 3's Composition API, lifecycle hooks are registered using specific functions provided by the Vue framework. These functions are imported from 'vue' and are called directly inside the <script></script>
section of a Vue component. Here's how you can register some of the common lifecycle hooks:
-
onMounted: This hook is called after the component has been mounted, meaning the component's template has been rendered in the DOM. To register it, you simply call
onMounted
and pass a callback function as an argument.import { onMounted } from 'vue'; export default { setup() { onMounted(() => { console.log('Component is mounted!'); }); } }
-
onUpdated: This hook is triggered after a component has updated, which means after a reactive data change has caused the re-rendering of the component. It's registered similarly to
onMounted
.import { onUpdated } from 'vue'; export default { setup() { onUpdated(() => { console.log('Component has updated!'); }); } }
-
onUnmounted: This hook is called after a component has been unmounted, meaning it has been removed from the DOM. You register it by calling
onUnmounted
with a callback function.import { onUnmounted } from 'vue'; export default { setup() { onUnmounted(() => { console.log('Component is unmounted!'); }); } }
These hooks allow you to execute code at specific points in a component's lifecycle, which is crucial for managing side effects, such as setting up and tearing down event listeners, timers, or fetching data.
What are the benefits of using lifecycle hooks in the Composition API compared to the Options API?
Using lifecycle hooks in the Composition API offers several benefits over the Options API:
- Better Code Organization: In the Composition API, you can group related logic together, including lifecycle hooks. This makes the code more readable and maintainable, as opposed to the Options API where lifecycle hooks are scattered throughout the component.
- Reusability: The Composition API allows you to extract and reuse lifecycle logic across different components more easily. You can create composable functions that include lifecycle hooks, which can then be used in multiple components.
- Clearer Dependency Tracking: With the Composition API, it's easier to see which reactive data or computed properties a lifecycle hook depends on, as they can be defined in close proximity. This is not as straightforward in the Options API, where dependencies might be spread across different sections.
- TypeScript Support: The Composition API integrates better with TypeScript, providing more type safety and better tooling support. Lifecycle hooks in the Composition API can be typed more effectively, reducing the chance of runtime errors.
- Flexibility: The Composition API allows for more flexible use of lifecycle hooks. For example, you can conditionally register a lifecycle hook or register it multiple times within the same component, which is not possible with the Options API.
How can lifecycle hooks in the Composition API help manage component state more effectively?
Lifecycle hooks in the Composition API can significantly enhance the management of component state by allowing developers to execute code at precise moments in a component's lifecycle. Here's how they contribute to effective state management:
-
Initialization and Cleanup:
onMounted
can be used to initialize state or fetch data when the component is first rendered. Conversely,onUnmounted
can be used to clean up resources, such as timers or event listeners, ensuring that the component's state is properly managed even after it's removed from the DOM. -
Reactive Updates:
onUpdated
can be used to react to changes in the component's state. For example, you might want to perform some action or update another part of the state whenever a specific piece of data changes. - Conditional Logic: Lifecycle hooks can be used to implement conditional logic based on the component's lifecycle state. For instance, you might only want to perform certain operations if the component is mounted or updated.
-
Error Handling:
onErrorCaptured
can be used to manage errors that occur during the component's lifecycle, allowing you to handle or log errors and update the component's state accordingly. -
Asynchronous Operations: Lifecycle hooks like
onMounted
are ideal for managing asynchronous operations, such as API calls. You can set up loading states and handle the data once it's received, ensuring that the component's state reflects the current status of the operation.
By leveraging these lifecycle hooks, developers can create more robust and efficient state management strategies within their Vue 3 components.
Can you provide an example of how to use onMounted and onUnmounted hooks together in a Vue 3 component?
Here's an example of how you can use onMounted
and onUnmounted
hooks together in a Vue 3 component to manage a timer that updates the component's state:
<template> <div> <p>Seconds elapsed: {{ seconds }}</p> </div> </template> <script> import { ref, onMounted, onUnmounted } from 'vue'; export default { setup() { const seconds = ref(0); let timer = null; onMounted(() => { // Start the timer when the component is mounted timer = setInterval(() => { seconds.value ; }, 1000); }); onUnmounted(() => { // Clean up the timer when the component is unmounted if (timer) { clearInterval(timer); } }); return { seconds }; } }; </script>
In this example, onMounted
is used to start a timer that increments the seconds
reactive reference every second. The onUnmounted
hook is used to clear the timer when the component is removed from the DOM, ensuring that the timer does not continue to run unnecessarily. This demonstrates how lifecycle hooks can be used to manage component state effectively by initializing and cleaning up resources at the appropriate times.
The above is the detailed content of How do you register lifecycle hooks (e.g., onMounted, onUpdated, onUnmounted) in the Composition API?. For more information, please follow other related articles on the PHP Chinese website!
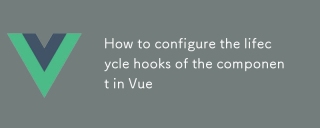
This article clarifies the role of export default in Vue.js components, emphasizing that it's solely for exporting, not configuring lifecycle hooks. Lifecycle hooks are defined as methods within the component's options object, their functionality un
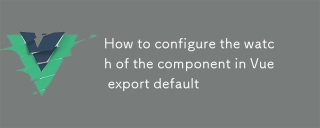
This article clarifies Vue.js component watch functionality when using export default. It emphasizes efficient watch usage through property-specific watching, judicious deep and immediate option use, and optimized handler functions. Best practices
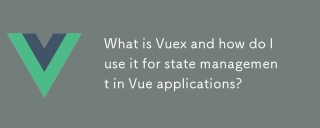
This article explains Vuex, a state management library for Vue.js. It details core concepts (state, getters, mutations, actions) and demonstrates usage, emphasizing its benefits for larger projects over simpler alternatives. Debugging and structuri
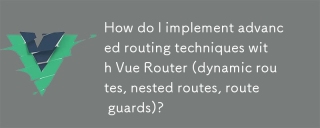
This article explores advanced Vue Router techniques. It covers dynamic routing (using parameters), nested routes for hierarchical navigation, and route guards for controlling access and data fetching. Best practices for managing complex route conf

Article discusses creating and using custom Vue.js plugins, including development, integration, and maintenance best practices.

The article explains how to configure Vue CLI for different build targets, switch environments, optimize production builds, and ensure source maps in development for debugging.

Vue.js enhances web development with its Component-Based Architecture, Virtual DOM for performance, and Reactive Data Binding for real-time UI updates.
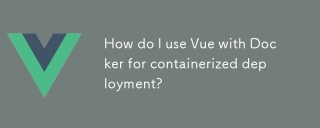
The article discusses using Vue with Docker for deployment, focusing on setup, optimization, management, and performance monitoring of Vue applications in containers.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
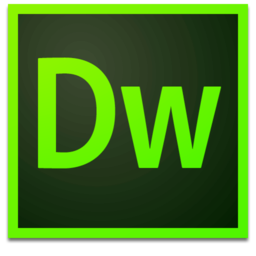
Dreamweaver Mac version
Visual web development tools
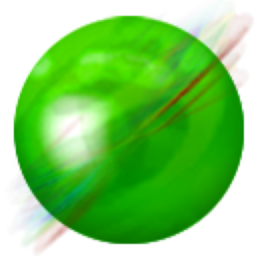
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
