Explain the core concepts of Pinia: stores, state, getters, actions.
Pinia is a store library for Vue.js, designed to provide a more intuitive and typed-friendly way of managing global state in Vue applications. Here's an overview of its core concepts:
Stores: In Pinia, a store is a container for your application's global state. It acts similarly to a Vue component but for state management. Stores are defined as composables and can be created using the defineStore
function. There are three types of stores in Pinia: setup, option, and auto-imported stores. Each store can hold state, getters, and actions.
State: The state in Pinia represents the data your application manages. It's reactive, meaning that any changes to the state will automatically trigger updates in components that use this state. You define state within the defineStore
function, and it can be accessed and modified throughout your application.
Getters: Getters in Pinia are similar to computed properties in Vue components. They allow you to derive data from the store's state. Getters are functions that can take the state or other getters as arguments and return computed values based on that state. They are cached based on their dependencies, which makes them efficient.
Actions: Actions are functions defined within a store that can contain both synchronous and asynchronous logic. They are used to perform operations that lead to state changes. Actions have access to the entire store's context, which means they can call other actions or commit changes directly to the state. They are typically used for more complex state mutations or for side effects like API calls.
What are the best practices for managing state with Pinia in a Vue.js application?
When using Pinia for state management in a Vue.js application, following best practices can enhance the maintainability and performance of your application:
- Organize Stores Logically: Group your stores based on the features or domains of your application. This keeps related state and logic together, making it easier to manage and maintain.
- Use Modules for Scalability: As your application grows, consider breaking down your store into smaller, modular stores. This approach helps in keeping the global state manageable and easier to reason about.
- Immutable Updates: Always return new objects instead of mutating existing state directly. This approach ensures better reactivity and helps in avoiding unexpected bugs.
- Use Getters for Computed State: Rely on getters to compute derived state. This helps in keeping your components clean and focused on rendering, while keeping the logic for state transformation in the store.
- Isolate Side Effects in Actions: Use actions for handling asynchronous operations and side effects. This keeps your state mutations predictable and easier to track.
- Testing: Write tests for your stores, especially for actions and getters. This helps ensure the reliability of your state management logic.
- Use TypeScript: If possible, use TypeScript with Pinia. It provides strong typing, which can prevent many state-related bugs and makes the code more maintainable.
How do getters in Pinia differ from computed properties in Vue.js?
Getters in Pinia and computed properties in Vue.js serve a similar purpose—they are used to compute derived state. However, there are key differences in their application and implementation:
- Scope and Usage: Getters are part of a Pinia store and are used to derive data from the global state managed by that store. On the other hand, computed properties are part of Vue components and derive data from the local state of the component or props it receives.
- Reactivity: Both getters and computed properties are reactive and cached based on their dependencies. However, getters in Pinia can access the entire state of the store, whereas computed properties are limited to the scope of the component they are defined in.
- Performance: Getters in Pinia can potentially be more performant in large applications because they are part of a centralized state management system, allowing for better optimization and caching mechanisms.
- Code Organization: Using getters in Pinia promotes a cleaner separation of concerns. State transformation logic is kept out of the components and inside the store, leading to more maintainable code.
Can actions in Pinia be used for asynchronous operations, and if so, how?
Yes, actions in Pinia can indeed be used for asynchronous operations. Actions are ideal for handling such operations due to their ability to include asynchronous code while managing the application's state. Here's how you can use actions for asynchronous operations:
-
Defining Asynchronous Actions: Actions are defined inside the
defineStore
function using theactions
option. You can useasync/await
or return a Promise to handle asynchronous operations within these actions.
const useUserStore = defineStore('user', { state: () => ({ userInfo: null, }), actions: { async fetchUserInfo(userId) { try { const response = await fetch(`/api/user/${userId}`); const data = await response.json(); this.userInfo = data; } catch (error) { console.error('Failed to fetch user info:', error); } }, }, });
- Calling Actions: Actions can be called from within components or other actions. They can be invoked using the store instance.
const userStore = useUserStore(); userStore.fetchUserInfo(123);
- Handling State Changes: After the asynchronous operation completes, you can update the store's state within the action. This ensures that your components stay in sync with the latest data.
- Error Handling: It's good practice to handle errors within actions to prevent unhandled rejections and to manage the state appropriately in case of failures.
By using actions for asynchronous operations, you keep your state management logic centralized and ensure that state changes remain predictable and manageable.
The above is the detailed content of Explain the core concepts of Pinia: stores, state, getters, actions.. For more information, please follow other related articles on the PHP Chinese website!
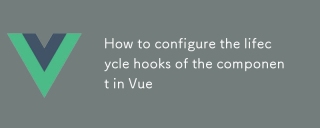
This article clarifies the role of export default in Vue.js components, emphasizing that it's solely for exporting, not configuring lifecycle hooks. Lifecycle hooks are defined as methods within the component's options object, their functionality un
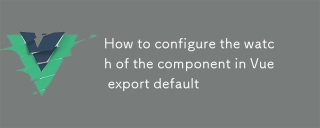
This article clarifies Vue.js component watch functionality when using export default. It emphasizes efficient watch usage through property-specific watching, judicious deep and immediate option use, and optimized handler functions. Best practices

Article discusses creating and using custom Vue.js plugins, including development, integration, and maintenance best practices.
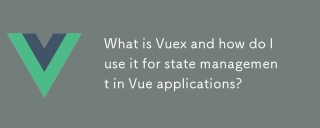
This article explains Vuex, a state management library for Vue.js. It details core concepts (state, getters, mutations, actions) and demonstrates usage, emphasizing its benefits for larger projects over simpler alternatives. Debugging and structuri

Vue.js enhances web development with its Component-Based Architecture, Virtual DOM for performance, and Reactive Data Binding for real-time UI updates.
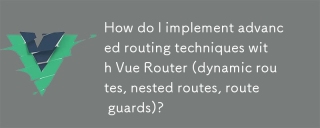
This article explores advanced Vue Router techniques. It covers dynamic routing (using parameters), nested routes for hierarchical navigation, and route guards for controlling access and data fetching. Best practices for managing complex route conf

The article explains how to configure Vue CLI for different build targets, switch environments, optimize production builds, and ensure source maps in development for debugging.
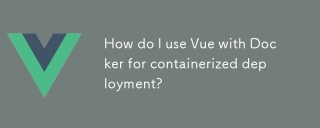
The article discusses using Vue with Docker for deployment, focusing on setup, optimization, management, and performance monitoring of Vue applications in containers.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
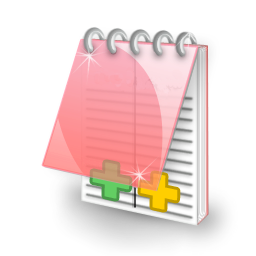
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor
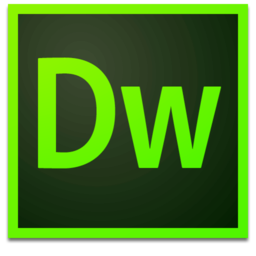
Dreamweaver Mac version
Visual web development tools
