How does Pinia simplify state management compared to Vuex?
Pinia simplifies state management in several key ways compared to Vuex, primarily by offering a more straightforward and intuitive API. Here’s a detailed breakdown:
- No Mutations: One of the most significant simplifications in Pinia is the elimination of mutations. In Vuex, mutations are the only way to change the state, which adds an extra layer of complexity and boilerplate code. In Pinia, you can directly modify the state within actions, similar to how you would modify a reactive object in Vue 3. This change reduces the cognitive load on developers and simplifies the codebase.
- Simplified Store Setup: Setting up a store in Pinia is more straightforward than in Vuex. In Vuex, you need to create a new store instance with separate sections for state, mutations, actions, and getters. In Pinia, you define a store using a single function, which encapsulates all these aspects in a more cohesive manner.
- TypeScript Support: Pinia has been designed with TypeScript in mind from the ground up, making it easier to work with typed state, actions, and getters. This is a significant advantage over Vuex, where TypeScript integration can be more cumbersome.
- Devtools Integration: Pinia integrates seamlessly with Vue Devtools, providing a more intuitive and user-friendly experience for debugging and inspecting state changes. This integration is more streamlined compared to Vuex, where additional setup might be required.
- Modular and Scalable: Pinia allows for easy creation of multiple stores, which can be combined or used independently. This modularity makes it easier to manage state in large applications, whereas Vuex can become more complex to manage as the application grows.
What specific features does Pinia offer that make it easier to manage state in Vue applications?
Pinia offers several specific features that enhance state management in Vue applications:
- Stores as Functions: Stores in Pinia are defined as functions, which makes them more intuitive to set up and use. This approach allows for better organization and encapsulation of state logic.
- Reactive State: Pinia leverages Vue 3's reactivity system, allowing you to directly modify the state without the need for mutations. This makes state management more aligned with Vue 3's reactive programming model.
- Getters as Computed Properties: In Pinia, getters are defined similarly to computed properties in Vue, making them easier to understand and use. This is a more natural fit for Vue developers compared to Vuex's separate getter syntax.
- Actions as Methods: Actions in Pinia are defined as methods within the store function, which can directly modify the state. This simplifies the process of handling asynchronous operations and state changes.
- Hot Module Replacement (HMR): Pinia supports HMR out of the box, allowing for seamless updates to the store during development without needing to refresh the page. This feature enhances the development experience and productivity.
- SSR Support: Pinia has built-in support for Server-Side Rendering (SSR), making it easier to use in SSR environments compared to Vuex, where additional configuration might be required.
How does the setup and configuration of Pinia differ from Vuex, and what benefits does this bring?
The setup and configuration of Pinia differ significantly from Vuex, offering several benefits:
-
Simplified Store Creation: In Pinia, you create a store using a single function, which encapsulates state, getters, and actions. In Vuex, you need to create a store instance with separate sections for state, mutations, actions, and getters. This simplification in Pinia reduces boilerplate and makes the code more readable.
// Pinia import { defineStore } from 'pinia' export const useCounterStore = defineStore('counter', { state: () => ({ count: 0 }), getters: { doubleCount: (state) => state.count * 2, }, actions: { increment() { this.count }, }, }) // Vuex import { createStore } from 'vuex' export default createStore({ state: { count: 0, }, mutations: { increment(state) { state.count }, }, actions: { increment({ commit }) { commit('increment') }, }, getters: { doubleCount: (state) => state.count * 2, }, })
- No Need for Mutations: Pinia eliminates the need for mutations, allowing direct state modifications within actions. This reduces complexity and aligns better with Vue 3's reactivity system.
- Easier TypeScript Integration: Pinia is designed with TypeScript in mind, making it easier to type your stores, state, actions, and getters. This is a significant advantage over Vuex, where TypeScript integration can be more challenging.
- Automatic Store Registration: In Pinia, stores are automatically registered when imported, eliminating the need for manual store registration. This simplifies the setup process and reduces the chance of errors.
- Benefits: These differences bring several benefits, including reduced boilerplate code, improved readability, easier maintenance, and better alignment with Vue 3's reactivity system. The simplified setup and configuration also make it easier for new developers to get started with state management in Vue applications.
Can Pinia's simpler API improve developer productivity compared to using Vuex?
Yes, Pinia's simpler API can significantly improve developer productivity compared to using Vuex. Here’s how:
- Reduced Boilerplate: Pinia's approach to state management eliminates the need for mutations and simplifies the store setup, reducing the amount of boilerplate code. This allows developers to focus more on application logic rather than on managing the state management framework.
- Easier Learning Curve: The simpler and more intuitive API of Pinia makes it easier for new developers to learn and understand state management in Vue applications. This can accelerate onboarding and reduce the time needed to become productive.
- Improved Code Readability: With Pinia, the state, getters, and actions are defined in a more cohesive and readable manner. This improved readability can make it easier for developers to understand and maintain the codebase, leading to faster development and fewer bugs.
- Better TypeScript Integration: Pinia's native support for TypeScript can enhance developer productivity by providing better type safety and autocompletion. This can reduce the time spent on debugging type-related issues and improve overall code quality.
- Enhanced Development Experience: Features like automatic store registration, seamless Devtools integration, and HMR support in Pinia contribute to a smoother development experience. These features can save time and reduce frustration during development, leading to higher productivity.
In summary, Pinia's simpler API, reduced boilerplate, and better alignment with Vue 3's reactivity system can significantly improve developer productivity by making state management more intuitive, easier to learn, and more efficient to maintain.
The above is the detailed content of How does Pinia simplify state management compared to Vuex?. For more information, please follow other related articles on the PHP Chinese website!

The main disadvantages of Vue.js include: 1. The ecosystem is relatively new, and third-party libraries and tools are not as rich as other frameworks; 2. The learning curve becomes steep in complex functions; 3. Community support and resources are not as extensive as React and Angular; 4. Performance problems may be encountered in large applications; 5. Version upgrades and compatibility challenges are greater.

Netflix uses React as its front-end framework. 1.React's component development and virtual DOM mechanism improve performance and development efficiency. 2. Use Webpack and Babel to optimize code construction and deployment. 3. Use code segmentation, server-side rendering and caching strategies for performance optimization.

Reasons for Vue.js' popularity include simplicity and easy learning, flexibility and high performance. 1) Its progressive framework design is suitable for beginners to learn step by step. 2) Component-based development improves code maintainability and team collaboration efficiency. 3) Responsive systems and virtual DOM improve rendering performance.

Vue.js is easier to use and has a smooth learning curve, which is suitable for beginners; React has a steeper learning curve, but has strong flexibility, which is suitable for experienced developers. 1.Vue.js is easy to get started with through simple data binding and progressive design. 2.React requires understanding of virtual DOM and JSX, but provides higher flexibility and performance advantages.

Vue.js is suitable for fast development and small projects, while React is more suitable for large and complex projects. 1.Vue.js is simple and easy to learn, suitable for rapid development and small projects. 2.React is powerful and suitable for large and complex projects. 3. The progressive features of Vue.js are suitable for gradually introducing functions. 4. React's componentized and virtual DOM performs well when dealing with complex UI and data-intensive applications.

Vue.js and React each have their own advantages and disadvantages. When choosing, you need to comprehensively consider team skills, project size and performance requirements. 1) Vue.js is suitable for fast development and small projects, with a low learning curve, but deep nested objects can cause performance problems. 2) React is suitable for large and complex applications, with a rich ecosystem, but frequent updates may lead to performance bottlenecks.

Vue.js is suitable for small to medium-sized projects, while React is suitable for large projects and complex application scenarios. 1) Vue.js is easy to use and is suitable for rapid prototyping and small applications. 2) React has more advantages in handling complex state management and performance optimization, and is suitable for large projects.

Vue.js and React each have their own advantages: Vue.js is suitable for small applications and rapid development, while React is suitable for large applications and complex state management. 1.Vue.js realizes automatic update through a responsive system, suitable for small applications. 2.React uses virtual DOM and diff algorithms, which are suitable for large and complex applications. When selecting a framework, you need to consider project requirements and team technology stack.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
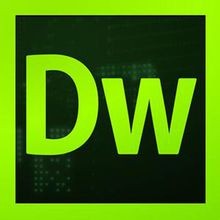
Dreamweaver CS6
Visual web development tools
