How do you handle asynchronous actions in Vuex?
In Vuex, asynchronous actions are handled using the actions
property within a Vuex store. Actions in Vuex are functions that can contain asynchronous operations and are typically used to commit mutations, which are synchronous transactions that mutate the state. Here's how you can handle asynchronous actions:
-
Define an Action: In your Vuex store, actions are defined in the
actions
object. An action is a function that receives acontext
object, which allows you to call other actions or commit mutations. -
Use Promises or Async/Await: To handle asynchronous operations, you can return a Promise from your action or use
async/await
syntax. This allows you to handle the asynchronous nature of operations like API calls.const store = new Vuex.Store({ state: { count: 0 }, mutations: { increment (state) { state.count } }, actions: { incrementAsync ({ commit }) { return new Promise((resolve, reject) => { setTimeout(() => { commit('increment') resolve() }, 1000) }) } } })
Or using
async/await
:actions: { async incrementAsync ({ commit }) { await new Promise(resolve => setTimeout(resolve, 1000)) commit('increment') } }
-
Dispatch Actions from Components: In your Vue components, you can dispatch these actions using the
this.$store.dispatch
method ormapActions
helper.this.$store.dispatch('incrementAsync').then(() => { console.log('Count incremented after 1 second') })
By following these steps, you can effectively handle asynchronous actions in Vuex, ensuring that your application's state is updated correctly after asynchronous operations complete.
What are the best practices for managing asynchronous operations in Vuex?
Managing asynchronous operations in Vuex effectively requires adherence to several best practices:
- Use Actions for Asynchronous Logic: Always use actions to handle asynchronous operations. Actions are the right place for side effects and asynchronous operations, keeping mutations purely synchronous.
- Return Promises from Actions: When possible, return Promises from actions. This allows components to wait for the action to complete before proceeding, which is useful for handling the UI state based on the result of the asynchronous operation.
-
Use Async/Await for Readability: Using
async/await
can make your code more readable and easier to understand, especially when dealing with multiple asynchronous operations. -
Handle Errors Appropriately: Always handle errors within your actions. You can use
try/catch
blocks to catch errors and commit mutations to update the state accordingly.actions: { async fetchData({ commit }) { try { const response = await fetch('/api/data') const data = await response.json() commit('setData', data) } catch (error) { commit('setError', error.message) } } }
- Avoid Direct State Mutations: Never mutate the state directly within an action. Always use mutations to change the state, ensuring that all state changes are trackable and predictable.
- Use Modules for Organized Code: If your store grows large, use modules to organize your actions, mutations, and state. This helps in managing complex asynchronous operations across different parts of your application.
By following these best practices, you can ensure that your Vuex store handles asynchronous operations efficiently and maintainably.
How can you ensure that asynchronous actions in Vuex do not lead to race conditions?
Race conditions in Vuex can occur when multiple asynchronous actions are dispatched in quick succession, potentially leading to unexpected state changes. Here are some strategies to prevent race conditions:
-
Use Unique Identifiers: When fetching data, use unique identifiers to track requests. This allows you to cancel or ignore outdated requests.
actions: { async fetchData({ commit, state }, id) { if (state.currentFetchId !== id) return try { const response = await fetch(`/api/data/${id}`) const data = await response.json() if (state.currentFetchId === id) { commit('setData', data) } } catch (error) { if (state.currentFetchId === id) { commit('setError', error.message) } } } }
-
Cancel Ongoing Requests: Implement a mechanism to cancel ongoing requests when a new request is made. This can be achieved using
AbortController
in modern browsers.actions: { async fetchData({ commit, state }) { if (state.abortController) { state.abortController.abort() } const controller = new AbortController() commit('setAbortController', controller) try { const response = await fetch('/api/data', { signal: controller.signal }) const data = await response.json() commit('setData', data) } catch (error) { if (error.name !== 'AbortError') { commit('setError', error.message) } } } }
- Debounce or Throttle Actions: Use debounce or throttle techniques to limit the frequency of action dispatches, especially for user-initiated actions like search queries.
- Use Optimistic UI Updates: Implement optimistic UI updates where you update the UI immediately and then revert if the asynchronous operation fails. This can help in reducing the impact of race conditions on the user experience.
By implementing these strategies, you can mitigate the risk of race conditions in your Vuex store, ensuring a more predictable and stable application state.
What tools or libraries can enhance the handling of asynchronous actions in Vuex?
Several tools and libraries can enhance the handling of asynchronous actions in Vuex:
-
Vuex ORM: Vuex ORM is a library that provides an Object-Relational Mapping (ORM) system for Vuex. It simplifies the management of complex data models and asynchronous operations by providing a more structured approach to state management.
import { Model } from '@vuex-orm/core' class User extends Model { static entity = 'users' static fields () { return { id: this.attr(null), name: this.attr(''), email: this.attr('') } } static async apiFetch (context, id) { const response = await fetch(`/api/users/${id}`) return await response.json() } }
-
Axios: While not specific to Vuex, Axios is a popular HTTP client that can be used within Vuex actions to handle API requests. It supports Promises and can be easily integrated with Vuex.
import axios from 'axios' actions: { async fetchData({ commit }) { try { const response = await axios.get('/api/data') commit('setData', response.data) } catch (error) { commit('setError', error.message) } } }
-
Vuex-Persist: Vuex-Persist is a library that helps in persisting and rehydrating your Vuex store. It can be useful for managing asynchronous operations that involve local storage or session storage.
import VuexPersist from 'vuex-persist' const vuexPersist = new VuexPersist({ storage: window.localStorage }) const store = new Vuex.Store({ plugins: [vuexPersist.plugin], // ... other store configurations })
-
Vuex Module Decorators: This library provides TypeScript decorators for Vuex modules, making it easier to manage asynchronous actions and state in a more type-safe manner.
import { VuexModule, Module, Mutation, Action } from 'vuex-module-decorators' @Module class UserModule extends VuexModule { public users: User[] = [] @Mutation public setUsers(users: User[]) { this.users = users } @Action public async fetchUsers() { const response = await fetch('/api/users') const users = await response.json() this.context.commit('setUsers', users) } }
By leveraging these tools and libraries, you can enhance the handling of asynchronous actions in Vuex, making your application more robust and easier to maintain.
The above is the detailed content of How do you handle asynchronous actions in Vuex?. For more information, please follow other related articles on the PHP Chinese website!

Vue.js is a progressive framework suitable for building highly interactive user interfaces. Its core functions include responsive systems, component development and routing management. 1) The responsive system realizes data monitoring through Object.defineProperty or Proxy, and automatically updates the interface. 2) Component development allows the interface to be split into reusable modules. 3) VueRouter supports single-page applications to improve user experience.

The main disadvantages of Vue.js include: 1. The ecosystem is relatively new, and third-party libraries and tools are not as rich as other frameworks; 2. The learning curve becomes steep in complex functions; 3. Community support and resources are not as extensive as React and Angular; 4. Performance problems may be encountered in large applications; 5. Version upgrades and compatibility challenges are greater.

Netflix uses React as its front-end framework. 1.React's component development and virtual DOM mechanism improve performance and development efficiency. 2. Use Webpack and Babel to optimize code construction and deployment. 3. Use code segmentation, server-side rendering and caching strategies for performance optimization.

Reasons for Vue.js' popularity include simplicity and easy learning, flexibility and high performance. 1) Its progressive framework design is suitable for beginners to learn step by step. 2) Component-based development improves code maintainability and team collaboration efficiency. 3) Responsive systems and virtual DOM improve rendering performance.

Vue.js is easier to use and has a smooth learning curve, which is suitable for beginners; React has a steeper learning curve, but has strong flexibility, which is suitable for experienced developers. 1.Vue.js is easy to get started with through simple data binding and progressive design. 2.React requires understanding of virtual DOM and JSX, but provides higher flexibility and performance advantages.

Vue.js is suitable for fast development and small projects, while React is more suitable for large and complex projects. 1.Vue.js is simple and easy to learn, suitable for rapid development and small projects. 2.React is powerful and suitable for large and complex projects. 3. The progressive features of Vue.js are suitable for gradually introducing functions. 4. React's componentized and virtual DOM performs well when dealing with complex UI and data-intensive applications.

Vue.js and React each have their own advantages and disadvantages. When choosing, you need to comprehensively consider team skills, project size and performance requirements. 1) Vue.js is suitable for fast development and small projects, with a low learning curve, but deep nested objects can cause performance problems. 2) React is suitable for large and complex applications, with a rich ecosystem, but frequent updates may lead to performance bottlenecks.

Vue.js is suitable for small to medium-sized projects, while React is suitable for large projects and complex application scenarios. 1) Vue.js is easy to use and is suitable for rapid prototyping and small applications. 2) React has more advantages in handling complex state management and performance optimization, and is suitable for large projects.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
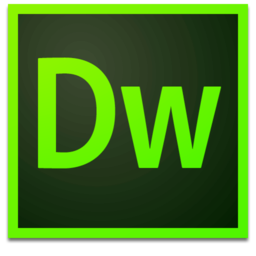
Dreamweaver Mac version
Visual web development tools
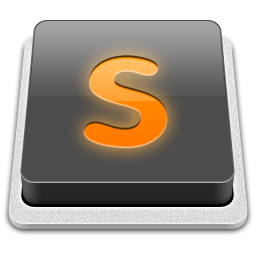
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
