How can you force an update of a component in Vue.js?
To force an update of a component in Vue.js, you can use the $forceUpdate
method. This method is available on every Vue instance and triggers a re-render of the component without changing its data or props. Here's how you can use it:
// Inside a Vue component this.$forceUpdate();
This method is useful when you need to force the Vue instance to re-render the component, especially in cases where the rendering logic depends on data that Vue cannot track, such as local state within the component's methods or computed properties that are not reactive.
What are the potential drawbacks of forcing a component update in Vue.js?
While $forceUpdate
can be useful, it comes with several potential drawbacks:
- Performance Impact: Forcing an update can lead to unnecessary re-renders, which can impact the performance of your application, especially if used frequently.
- Bypassing Reactivity: Vue.js is designed to use a reactive system to manage the state and update the DOM efficiently. By forcing an update, you bypass this system, which can lead to less predictable and more complex code.
-
Debugging Challenges: Since
$forceUpdate
skips the usual reactive updates, it can make debugging more challenging, as the usual tools and techniques might not catch changes caused by forced updates. -
Potential for Overuse: There's a temptation to use
$forceUpdate
as a quick fix for issues that could be solved by better state management or more correct use of Vue's reactivity system.
Are there alternative methods to achieve the same result without forcing an update in Vue.js?
Yes, there are alternative methods to achieve similar results without resorting to forcing an update:
-
Use of
Vue.set
: If you need to add a new property to a reactive object, useVue.set
to make it reactive.Vue.set(object, key, value);
- Reactive Data: Ensure that all data that affects the rendering of your component is reactive. Use Vue's data properties, computed properties, and watchers to keep your component's state reactive.
-
Key Attribute: If you're dealing with lists, use the
key
attribute to help Vue track the identity of elements and re-render them correctly.<div v-for="item in items" :key="item.id"> {{ item.name }} </div>
-
Computed Properties: Use computed properties to derive values from your state, which will automatically update when the state changes.
computed: { fullName() { return this.firstName ' ' this.lastName; } }
-
Watchers: Use watchers to react to changes in data and perform actions that might affect the rendering.
watch: { someData(newVal, oldVal) { // Perform actions based on the change } }
What specific scenarios justify the use of forced updates in Vue.js applications?
While it's generally recommended to avoid using $forceUpdate
, there are specific scenarios where it might be justified:
- Third-Party Libraries: When integrating third-party libraries that modify the DOM directly, you might need to force an update to ensure Vue's virtual DOM is in sync with the actual DOM.
- Non-Reactive Data: If you have data that Vue cannot track (e.g., data stored in a closure or a third-party state management system), you might need to force an update to reflect changes in the UI.
- Complex Computed Properties: In rare cases, if you have complex computed properties that depend on non-reactive data, you might need to force an update to ensure the UI reflects the latest state.
-
Debugging and Testing: During development, you might use
$forceUpdate
to test how your component behaves under forced re-renders, helping you understand and debug the component's rendering logic.
In summary, while $forceUpdate
can be a useful tool in specific situations, it should be used sparingly and with caution, as it can lead to performance issues and make your application harder to maintain. Always consider alternative, more reactive approaches first.
The above is the detailed content of How can you force an update of a component in Vue.js?. For more information, please follow other related articles on the PHP Chinese website!
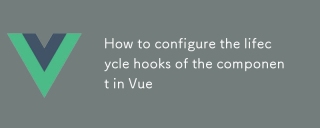
This article clarifies the role of export default in Vue.js components, emphasizing that it's solely for exporting, not configuring lifecycle hooks. Lifecycle hooks are defined as methods within the component's options object, their functionality un
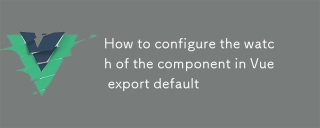
This article clarifies Vue.js component watch functionality when using export default. It emphasizes efficient watch usage through property-specific watching, judicious deep and immediate option use, and optimized handler functions. Best practices

Article discusses creating and using custom Vue.js plugins, including development, integration, and maintenance best practices.
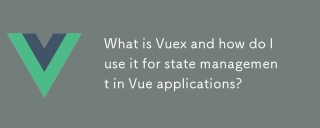
This article explains Vuex, a state management library for Vue.js. It details core concepts (state, getters, mutations, actions) and demonstrates usage, emphasizing its benefits for larger projects over simpler alternatives. Debugging and structuri

Vue.js enhances web development with its Component-Based Architecture, Virtual DOM for performance, and Reactive Data Binding for real-time UI updates.
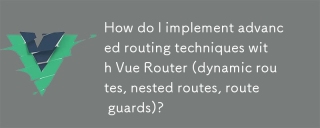
This article explores advanced Vue Router techniques. It covers dynamic routing (using parameters), nested routes for hierarchical navigation, and route guards for controlling access and data fetching. Best practices for managing complex route conf

The article explains how to configure Vue CLI for different build targets, switch environments, optimize production builds, and ensure source maps in development for debugging.
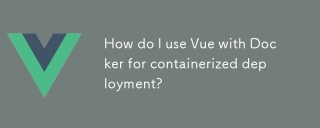
The article discusses using Vue with Docker for deployment, focusing on setup, optimization, management, and performance monitoring of Vue applications in containers.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
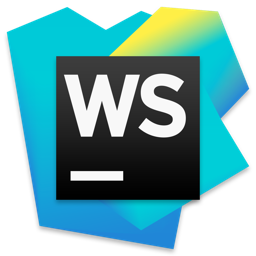
WebStorm Mac version
Useful JavaScript development tools
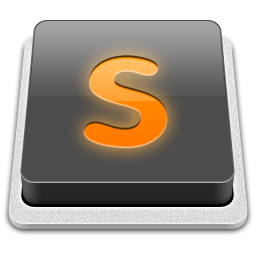
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
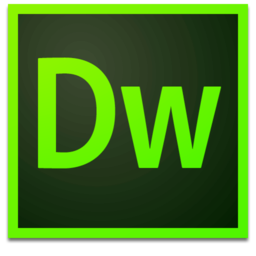
Dreamweaver Mac version
Visual web development tools
