What is the purpose of .sync modifier in Vue 2? How is it replaced in Vue 3?
What is the purpose of .sync modifier in Vue 2? How is it replaced in Vue 3?
The .sync
modifier in Vue 2 is a shorthand syntax that facilitates two-way data binding between a parent component and a child component. It was originally designed to simplify the process of updating a prop from a child component, which would reflect the changes in the parent component as well. For example, if a parent component passes a value
prop to a child component, and that child component needs to update value
, the parent would typically listen to an event and update value
itself. The .sync
modifier simplifies this process by automatically creating the event listener and updating the prop.
In Vue 2, the .sync
modifier can be used like this:
<my-component v-bind:value.sync="message"></my-component>
This is equivalent to:
<my-component :value="message" @update:value="val => message = val"> </my-component>
When the child component emits an update:value
event, the parent's message
will be updated automatically.
In Vue 3, the .sync
modifier has been removed as part of the effort to streamline and simplify the API. The recommended approach in Vue 3 is to use the v-model
directive, which is extended to support custom events for more flexible two-way binding. Instead of using .sync
, developers can use v-model
like this:
<my-component v-model="message"></my-component>
This is now a shorthand for:
<my-component :modelValue="message" @update:modelValue="val => message = val"> </my-component>
This change aligns more closely with the component design principles of Vue and encourages more explicit and clear communication between components.
What are the benefits of using the .sync modifier in Vue 2 for component communication?
Using the .sync
modifier in Vue 2 offers several benefits for component communication:
-
Simplified Two-Way Binding: It simplifies the process of two-way data binding. Without
.sync
, developers would need to manually emit an event from the child component and listen for it in the parent to update the prop, which can be cumbersome and error-prone. -
Reduced Boilerplate Code: By using
.sync
, developers can write less code. Instead of setting up an event listener and manually updating the parent data, the.sync
modifier handles this automatically, reducing the amount of boilerplate code needed. -
Improved Readability: The
.sync
modifier makes it clear at a glance that a prop is being used for two-way binding. This improves the readability of the component's template and makes the component's behavior more predictable. -
Consistency with Form Inputs: The
.sync
modifier brings the behavior of custom components closer to native form inputs, where two-way data binding is common. This can make custom components feel more intuitive to use. -
Flexibility in Event Naming: The
.sync
modifier allows for more flexibility in naming custom events. Developers can useupdate:myProp
to indicate that a prop namedmyProp
is being updated, which is more explicit than a generic event name.
How does the removal of the .sync modifier in Vue 3 affect the way developers handle props?
The removal of the .sync
modifier in Vue 3 shifts the approach to handling props towards more explicit and declarative methods. Here are the key impacts on how developers handle props:
-
Explicit Event Handling: Without
.sync
, developers must explicitly define event listeners in the parent component to handle updates from child components. This can lead to clearer code as the flow of data is more transparent. -
Use of
v-model
: Vue 3 encourages the use ofv-model
for two-way data binding, not just for form inputs but also for custom components. This means developers need to adapt their components to work withv-model
, usingmodelValue
andupdate:modelValue
instead of custom prop and event names. -
More Predictable Component Behavior: By removing
.sync
, Vue 3 promotes a more predictable and standardized approach to component communication. This can reduce confusion and errors that might arise from the less explicit nature of.sync
. -
Encouragement of Best Practices: The removal of
.sync
pushes developers to follow best practices in component design, such as using events and props in a more standardized way. This can lead to better-maintained and more scalable codebases. -
Backward Compatibility: For developers migrating from Vue 2 to Vue 3, the removal of
.sync
requires updates to existing code. However, Vue 3 provides a configuration optioncompilerOptions.sync
that can be used to enable.sync
syntax temporarily during migration.
What alternative methods can developers use in Vue 3 to achieve the same functionality as the .sync modifier in Vue 2?
To achieve the same functionality as the .sync
modifier in Vue 2, developers can use several alternative methods in Vue 3:
-
Using
v-model
:
As mentioned earlier,v-model
is the primary replacement for.sync
in Vue 3. It is not limited to form inputs and can be used with custom components for two-way data binding. For a custom component to work withv-model
, it should usemodelValue
as the prop name and emit anupdate:modelValue
event when the value changes.Example:
<my-component v-model="message"></my-component>
-
Explicit Event Listeners and Prop Updates:
Developers can manually set up event listeners in the parent component to handle updates from child components. This involves passing a prop to the child and listening for an event that indicates the prop should be updated.Example:
<template> <child-component :value="message" @update:value="newValue => message = newValue" ></child-component> </template>
-
Computed Properties:
In cases where simple prop passing and event handling are not sufficient, developers can use computed properties to manage complex two-way bindings. A computed property can be used to derive the value of a prop and update it when necessary.Example:
<template> <child-component :value="computedValue" @update:value="updateComputedValue"></child-component> </template> <script> export default { data() { return { message: 'Hello' } }, computed: { computedValue: { get() { return this.message; }, set(newValue) { this.message = newValue; } } }, methods: { updateComputedValue(newValue) { this.computedValue = newValue; } } } </script>
-
Using the
compilerOptions.sync
Configuration:
For projects migrating from Vue 2 to Vue 3, developers can temporarily enable the.sync
syntax using thecompilerOptions.sync
configuration in the Vue build process. This allows for a smoother transition but should be used as a temporary measure.Example (in
vue.config.js
):module.exports = { chainWebpack: config => { config.module .rule('vue') .use('vue-loader') .tap(options => { options.compilerOptions = { ...options.compilerOptions, sync: true } return options }) } }
By adopting these alternative methods, developers can achieve the same level of two-way data binding and component communication in Vue 3 that was possible with the .sync
modifier in Vue 2.
The above is the detailed content of What is the purpose of .sync modifier in Vue 2? How is it replaced in Vue 3?. For more information, please follow other related articles on the PHP Chinese website!

Vue.js and React each have their own advantages in scalability and maintainability. 1) Vue.js is easy to use and is suitable for small projects. The Composition API improves the maintainability of large projects. 2) React is suitable for large and complex projects, with Hooks and virtual DOM improving performance and maintainability, but the learning curve is steeper.

The future trends and forecasts of Vue.js and React are: 1) Vue.js will be widely used in enterprise-level applications and have made breakthroughs in server-side rendering and static site generation; 2) React will innovate in server components and data acquisition, and further optimize the concurrency model.

Netflix's front-end technology stack is mainly based on React and Redux. 1.React is used to build high-performance single-page applications, and improves code reusability and maintenance through component development. 2. Redux is used for state management to ensure that state changes are predictable and traceable. 3. The toolchain includes Webpack, Babel, Jest and Enzyme to ensure code quality and performance. 4. Performance optimization is achieved through code segmentation, lazy loading and server-side rendering to improve user experience.

Vue.js is a progressive framework suitable for building highly interactive user interfaces. Its core functions include responsive systems, component development and routing management. 1) The responsive system realizes data monitoring through Object.defineProperty or Proxy, and automatically updates the interface. 2) Component development allows the interface to be split into reusable modules. 3) VueRouter supports single-page applications to improve user experience.

The main disadvantages of Vue.js include: 1. The ecosystem is relatively new, and third-party libraries and tools are not as rich as other frameworks; 2. The learning curve becomes steep in complex functions; 3. Community support and resources are not as extensive as React and Angular; 4. Performance problems may be encountered in large applications; 5. Version upgrades and compatibility challenges are greater.

Netflix uses React as its front-end framework. 1.React's component development and virtual DOM mechanism improve performance and development efficiency. 2. Use Webpack and Babel to optimize code construction and deployment. 3. Use code segmentation, server-side rendering and caching strategies for performance optimization.

Reasons for Vue.js' popularity include simplicity and easy learning, flexibility and high performance. 1) Its progressive framework design is suitable for beginners to learn step by step. 2) Component-based development improves code maintainability and team collaboration efficiency. 3) Responsive systems and virtual DOM improve rendering performance.

Vue.js is easier to use and has a smooth learning curve, which is suitable for beginners; React has a steeper learning curve, but has strong flexibility, which is suitable for experienced developers. 1.Vue.js is easy to get started with through simple data binding and progressive design. 2.React requires understanding of virtual DOM and JSX, but provides higher flexibility and performance advantages.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

Zend Studio 13.0.1
Powerful PHP integrated development environment
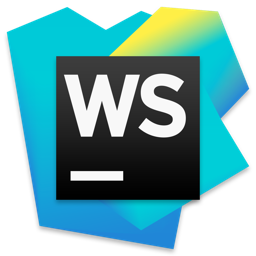
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
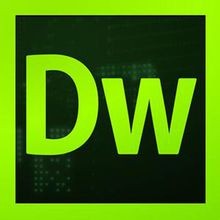
Dreamweaver CS6
Visual web development tools
