How do you handle errors and loading states when making API requests?
Handling errors and loading states during API requests involves several steps that ensure a smooth user experience and robust application behavior. Here's a detailed approach:
-
Loading State:
- When an API request is initiated, immediately set a loading state flag to
true
. This flag can be used to show loading indicators like spinners or skeleton screens to the user, indicating that a process is ongoing. - Use this flag to disable form submissions or buttons that triggered the API call to prevent multiple requests.
- When an API request is initiated, immediately set a loading state flag to
-
Error Handling:
- Use try-catch blocks or promise
.catch()
methods to capture errors during the API call. - Once an error is caught, set an error state to
true
and store the error message or error object for display. - Ensure the loading state is set back to
false
so that the user knows the process has concluded, albeit with an error.
- Use try-catch blocks or promise
-
Success Handling:
- Upon receiving a successful response, set the loading state to
false
. - Update your application state with the data received from the API.
- Optionally, set a success state to
true
if you need to show a success message or animation to the user.
- Upon receiving a successful response, set the loading state to
-
State Management:
- Use state management solutions like React Context, Redux, or Vuex to handle loading and error states across your application effectively.
- These tools help in managing global states, making it easier to toggle states like loading and error throughout your app.
By following these steps, you can effectively manage errors and loading states, providing a responsive and user-friendly experience during API interactions.
What are the best practices for displaying error messages to users during API requests?
Displaying error messages effectively is crucial for maintaining user trust and aiding in troubleshooting. Here are some best practices:
-
Be Clear and Concise:
- Error messages should be straightforward and easy to understand. Avoid technical jargon unless your audience is technical.
-
Provide Actionable Information:
- Include suggestions on how the user can resolve the issue, such as retrying the request, checking their internet connection, or contacting support.
-
Use Appropriate Visual Cues:
- Use colors like red or orange to signify errors, but ensure they are accessible to color-blind users.
- Consider using icons (e.g., an exclamation mark) to visually indicate an error.
-
Positioning and Timing:
- Display error messages close to the action that caused the error, such as near a form field or button.
- Show the error message immediately after the error occurs, but allow users to dismiss it easily.
-
Logging and Reporting:
- Log errors on the server-side for debugging and improvement purposes.
- Consider providing users with an error ID or code that they can reference when seeking help.
-
User-Friendly Language:
- Use friendly and empathetic language. Instead of saying "Error 404," you might say, "We couldn't find the page you're looking for. Let's try again?"
By adhering to these practices, you can ensure that error messages are not only informative but also enhance the overall user experience.
How can you implement a smooth loading state experience for users while waiting for API responses?
Implementing a smooth loading state experience involves several techniques to keep users engaged and informed during API requests:
-
Skeleton Screens:
- Use skeleton screens to show a preview of the content structure before the actual data loads. This gives users a sense of what to expect and reduces perceived load times.
-
Progress Indicators:
- Implement progress bars or spinners to visually indicate that the application is working on a task. Ensure these indicators are placed in a prominent position on the screen.
-
Optimistic UI Updates:
- For actions like form submissions, update the UI optimistically to reflect the expected outcome before the API response is received. If the API call fails, revert the UI gracefully.
-
Lazy Loading:
- Load content in chunks or as the user scrolls, reducing the initial load time and providing a smoother experience.
-
Feedback on Long Operations:
- For operations that might take longer, provide feedback on the progress, such as "50% complete" or "Estimated time remaining: 2 minutes."
-
Avoid Blocking the UI:
- Ensure that the UI remains responsive during loading states. Users should be able to navigate away or perform other actions if needed.
-
Use of Animations:
- Subtle animations can make the loading process feel more dynamic and less static, enhancing the user experience.
By incorporating these techniques, you can create a loading state that feels smooth and keeps users informed and engaged.
What strategies can be used to effectively retry failed API requests due to transient errors?
Retrying failed API requests due to transient errors can significantly improve the reliability of your application. Here are some effective strategies:
-
Exponential Backoff:
- Implement an exponential backoff strategy where the time between retries increases exponentially (e.g., 1 second, 2 seconds, 4 seconds, etc.). This helps in managing server load and increasing the chances of a successful retry.
-
Limited Number of Retries:
- Set a maximum number of retry attempts to avoid infinite loops. A common practice is to allow 3 to 5 retries.
-
Jitter:
- Add a random delay (jitter) to the retry intervals to prevent synchronized retries from multiple clients, which could otherwise overwhelm the server.
-
Circuit Breaker Pattern:
- Use a circuit breaker to stop retrying after a certain number of failures. Once the circuit is "open," it prevents further requests until a timeout period has passed, allowing the system to recover.
-
Retry on Specific Errors:
- Only retry on errors that are likely to be transient, such as network timeouts or 5xx HTTP status codes. Do not retry on errors like 400 Bad Request, which indicate a client-side issue.
-
User Notification and Control:
- Inform users about the retry attempts and allow them to cancel or manually trigger retries if needed.
-
Logging and Monitoring:
- Log retry attempts and outcomes for debugging and monitoring purposes. This can help in identifying patterns and improving the retry logic.
By implementing these strategies, you can enhance the resilience of your application and provide a better experience for users facing transient errors during API requests.
The above is the detailed content of How do you handle errors and loading states when making API requests?. For more information, please follow other related articles on the PHP Chinese website!
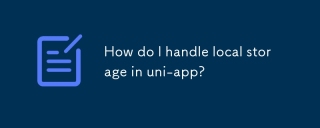
This article details uni-app's local storage APIs (uni.setStorageSync(), uni.getStorageSync(), and their async counterparts), emphasizing best practices like using descriptive keys, limiting data size, and handling JSON parsing. It stresses that lo
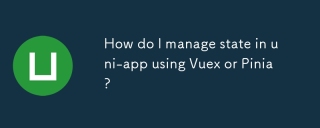
This article compares Vuex and Pinia for state management in uni-app. It details their features, implementation, and best practices, highlighting Pinia's simplicity versus Vuex's structure. The choice depends on project complexity, with Pinia suita
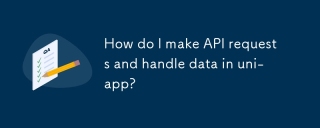
This article details making and securing API requests within uni-app using uni.request or Axios. It covers handling JSON responses, best security practices (HTTPS, authentication, input validation), troubleshooting failures (network issues, CORS, s

This article details uni-app's geolocation APIs, focusing on uni.getLocation(). It addresses common pitfalls like incorrect coordinate systems (gcj02 vs. wgs84) and permission issues. Improving location accuracy via averaging readings and handling
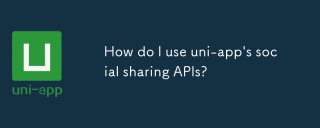
The article details how to integrate social sharing into uni-app projects using uni.share API, covering setup, configuration, and testing across platforms like WeChat and Weibo.
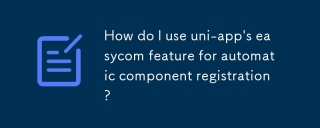
This article explains uni-app's easycom feature, automating component registration. It details configuration, including autoscan and custom component mapping, highlighting benefits like reduced boilerplate, improved speed, and enhanced readability.
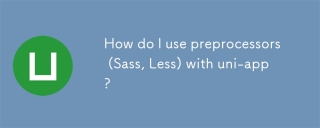
Article discusses using Sass and Less preprocessors in uni-app, detailing setup, benefits, and dual usage. Main focus is on configuration and advantages.[159 characters]
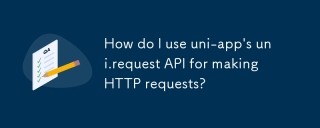
This article details uni.request API in uni-app for making HTTP requests. It covers basic usage, advanced options (methods, headers, data types), robust error handling techniques (fail callbacks, status code checks), and integration with authenticat


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
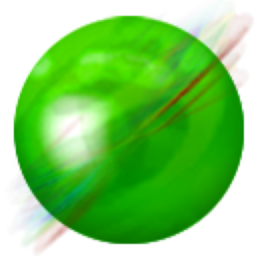
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
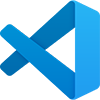
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),