How to Use uni.request API for Making HTTP Requests
The uni.request
API in uni-app is a versatile tool for making HTTP requests to various servers. It's a wrapper around the native XMLHttpRequest object, providing a more convenient and cross-platform approach. Here's a detailed breakdown of how to use it:
Basic Usage:
The core function is uni.request()
, which takes an options object as its argument. This object specifies the request details. A simple GET request might look like this:
uni.request({ url: 'https://api.example.com/data', method: 'GET', success: (res) => { console.log('Request successful:', res.data); }, fail: (err) => { console.error('Request failed:', err); }, complete: (res) => { console.log('Request completed:', res); } });
This code sends a GET request to https://api.example.com/data
. The success
callback handles successful responses, fail
handles errors, and complete
executes regardless of success or failure. res.data
contains the response data.
Advanced Options:
uni.request
supports various options for customizing your requests:
-
method
: Specifies the HTTP method (GET, POST, PUT, DELETE, etc.). Defaults to GET. -
data
: The data to send with the request (usually for POST, PUT, etc.). This can be an object or a string. -
header
: An object containing HTTP headers (e.g.,Content-Type
,Authorization
). -
dataType
: Specifies the expected data type of the response ('json' is common). -
responseType
: Specifies the expected response type ('text', 'arraybuffer', etc.). -
timeout
: Sets a timeout for the request in milliseconds.
Example POST request:
uni.request({ url: 'https://api.example.com/submit', method: 'POST', header: { 'Content-Type': 'application/json' }, data: { name: 'John Doe', email: 'john.doe@example.com' }, success: (res) => { // ... }, fail: (err) => { // ... } });
What are the Common Error Handling Techniques When Using uni.request in uni-app?
Robust error handling is crucial for a smooth user experience. Here are common techniques for handling errors with uni.request
:
-
fail
Callback: Thefail
callback is the primary mechanism. It receives an error object containing information about the failure (e.g., status code, error message). Use this to provide informative error messages to the user or log the error for debugging. -
Status Code Checking: Check the HTTP status code in the
fail
callback (or even incomplete
for more comprehensive handling). Different status codes indicate different issues (404 Not Found, 500 Internal Server Error, etc.). Handle these cases differently, providing tailored user feedback. -
Network Error Handling: Detect network connectivity issues.
uni.request
might fail due to a lack of internet connection. You can useuni.getSystemInfoSync().networkType
to check the network status before making the request or handle network errors specifically within thefail
callback. -
Try...Catch Blocks: While less common with
uni.request
which already provides callbacks, you could wrap theuni.request
call in atry...catch
block to catch unexpected errors that might occur outside the request itself (e.g., JSON parsing errors). - Generic Error Handling: Provide a generic error message to the user if the specific error is unclear or too technical. Log the full error details for debugging purposes.
Example with status code checking:
uni.request({ // ... request options ... fail: (err) => { if (err.statusCode === 404) { uni.showToast({ title: 'Resource not found', icon: 'error' }); } else if (err.statusCode === 500) { uni.showToast({ title: 'Server error', icon: 'error' }); } else { uni.showToast({ title: 'An error occurred', icon: 'error' }); console.error('Request failed:', err); } } });
How Can I Integrate uni.request with My uni-app Project's Authentication System?
Integrating uni.request
with an authentication system typically involves adding an Authorization header to each request. This header usually contains a token (JWT, session ID, etc.) that identifies the authenticated user.
Implementation:
-
Token Storage: Store the authentication token securely (e.g., in uni-app's storage using
uni.setStorageSync
anduni.getStorageSync
). -
Header Injection: Before making each request, retrieve the token and add it to the
header
object:
const token = uni.getStorageSync('token'); uni.request({ url: 'https://api.example.com/protected-data', header: { 'Authorization': `Bearer ${token}` // Adjust as needed for your auth scheme }, success: (res) => { // ... }, fail: (err) => { // Handle authentication errors (e.g., 401 Unauthorized) if (err.statusCode === 401) { // Redirect to login or refresh token } } });
- Token Refreshing: Implement token refreshing if your authentication system uses short-lived tokens. Check the token's expiration and automatically refresh it before it expires. This usually involves making a separate request to a token refresh endpoint.
- Error Handling: Handle authentication errors (like 401 Unauthorized) appropriately. This might involve redirecting the user to the login page or prompting them to re-authenticate.
Can I Use uni.request to Upload Files in My uni-app Project?
Yes, uni.request
can upload files, but it requires using the formData
API. Here's how:
Implementation:
-
Create FormData: Create a
FormData
object and append the file to it. You'll need to access the file using the appropriate uni-app file selection API (e.g.,uni.chooseImage
oruni.chooseVideo
). -
Set Content-Type: Set the
Content-Type
header tomultipart/form-data
. -
Send the Request: Send a POST request with the
FormData
object as thedata
.
Example:
uni.chooseImage({ count: 1, success: (res) => { const filePath = res.tempFiles[0].path; const formData = new FormData(); formData.append('file', { uri: filePath, name: 'file.jpg', // Adjust filename as needed type: 'image/jpeg' // Adjust file type as needed }); uni.request({ url: 'https://api.example.com/upload', method: 'POST', header: { 'Content-Type': 'multipart/form-data' }, data: formData, success: (res) => { // ... }, fail: (err) => { // ... } }); } });
Remember to adjust the name
and type
properties according to your uploaded file. The server-side needs to be configured to handle multipart/form-data
uploads. Also, consider using a progress indicator to show upload progress to the user for a better user experience, which usually requires a different approach beyond the basic uni.request
.
The above is the detailed content of How do I use uni-app's uni.request API for making HTTP requests?. For more information, please follow other related articles on the PHP Chinese website!
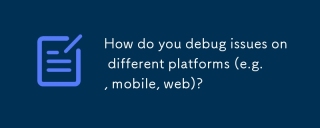
The article discusses debugging strategies for mobile and web platforms, highlighting tools like Android Studio, Xcode, and Chrome DevTools, and techniques for consistent results across OS and performance optimization.

The article discusses debugging tools and best practices for UniApp development, focusing on tools like HBuilderX, WeChat Developer Tools, and Chrome DevTools.

The article discusses end-to-end testing for UniApp applications across multiple platforms. It covers defining test scenarios, choosing tools like Appium and Cypress, setting up environments, writing and running tests, analyzing results, and integrat
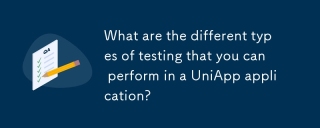
The article discusses various testing types for UniApp applications, including unit, integration, functional, UI/UX, performance, cross-platform, and security testing. It also covers ensuring cross-platform compatibility and recommends tools like Jes
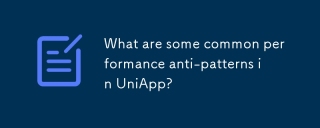
The article discusses common performance anti-patterns in UniApp development, such as excessive global data use and inefficient data binding, and offers strategies to identify and mitigate these issues for better app performance.
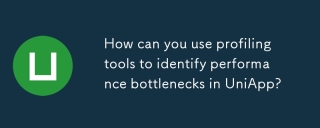
The article discusses using profiling tools to identify and resolve performance bottlenecks in UniApp, focusing on setup, data analysis, and optimization.
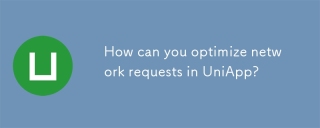
The article discusses strategies for optimizing network requests in UniApp, focusing on reducing latency, implementing caching, and using monitoring tools to enhance application performance.
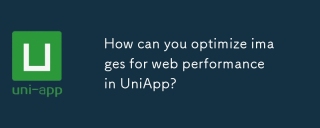
The article discusses optimizing images in UniApp for better web performance through compression, responsive design, lazy loading, caching, and using WebP format.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
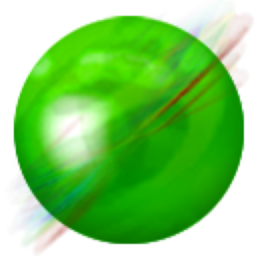
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
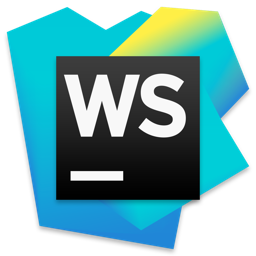
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.