


What are the different memory ordering constraints available for atomic operations?
Atomic operations are crucial in concurrent programming as they allow operations to be performed in a thread-safe manner. Memory ordering constraints, also known as memory models or memory ordering semantics, dictate how memory operations from multiple threads are observed by each other. The specific constraints available can vary depending on the programming language or hardware architecture, but common memory ordering constraints for atomic operations include:
- Sequential Consistency (SC): This is the strongest memory ordering constraint where all operations appear to happen in a single, total order that is consistent across all threads. This means that any operation performed by any thread must be visible in the same order to all other threads.
- Acquire-Release (AR): This model is commonly used in C and other languages. "Acquire" operations ensure that no memory accesses that appear after the acquire in program order may be reordered before it. Conversely, "Release" operations ensure that no memory accesses that appear before the release in program order may be reordered after it. This model is weaker than sequential consistency but still provides strong guarantees necessary for many concurrent algorithms.
- Relaxed Ordering: This is the weakest form of memory ordering where atomic operations do not provide any ordering guarantees relative to other memory operations except that the atomic operation itself is executed atomically. This can be useful for counters and other operations where the exact order of updates is not important.
- Consume Ordering: Similar to acquire ordering, but only orders dependent reads. It is weaker than acquire ordering and is less commonly used because its semantics can be complex and hardware support may vary.
These memory ordering constraints allow developers to balance the need for correct concurrent behavior with the need for performance optimization, as stronger ordering constraints typically result in more overhead.
What are the performance implications of using different memory ordering constraints in atomic operations?
The choice of memory ordering constraint can significantly impact the performance of concurrent programs. Here's how each constraint typically affects performance:
- Sequential Consistency (SC): As the strongest model, it offers the most intuitive behavior but can incur the highest overhead. Processors need to ensure that all operations are globally visible in a consistent order, which often requires flushing caches or other synchronization mechanisms that can slow down execution.
- Acquire-Release (AR): This model allows for some optimizations compared to SC. The use of "acquire" and "release" semantics enables processors to reorder independent memory operations as long as the dependency order is maintained. This can reduce the number of synchronization operations required, leading to improved performance over SC.
- Relaxed Ordering: Offering the least overhead, relaxed ordering can provide significant performance benefits in scenarios where ordering is not critical. By allowing more aggressive reordering of operations, processors can optimize memory access patterns more effectively. However, it requires careful use to ensure correctness.
- Consume Ordering: This constraint can offer performance similar to or slightly better than acquire-release, depending on the hardware and the specific use case. However, its effectiveness can be limited by its complexity and inconsistent hardware support.
In summary, weaker memory ordering constraints generally result in better performance because they allow more freedom for processors to optimize memory operations, but they also require more careful programming to ensure correct behavior.
How do memory ordering constraints affect the correctness of concurrent programs using atomic operations?
Memory ordering constraints play a pivotal role in ensuring the correctness of concurrent programs that use atomic operations. The choice of constraint directly impacts how operations performed by different threads are observed by each other, which can either prevent or introduce race conditions and other concurrency issues. Here’s how each constraint influences correctness:
- Sequential Consistency (SC): With SC, all threads see all operations in the same order, making it easier to reason about the program's behavior and avoid race conditions. However, it may lead to unnecessary synchronization if not all operations require such strong ordering.
- Acquire-Release (AR): This model can ensure correctness for many common synchronization patterns, such as locks and semaphores. It helps prevent race conditions by ensuring that operations before a release are visible to operations after an acquire. However, misusing acquire-release semantics can still lead to subtle bugs if the programmer assumes stronger ordering than is actually provided.
- Relaxed Ordering: Using relaxed ordering can lead to correctness issues if not handled carefully. Without ordering guarantees, operations might appear to happen out of order to different threads, leading to race conditions or unexpected behaviors. Relaxed ordering should only be used when the exact order of operations is not critical to the program's correctness.
- Consume Ordering: This constraint can be tricky to use correctly due to its dependency on the compiler and hardware. If used incorrectly, it might not provide the necessary ordering guarantees, leading to race conditions. It is generally recommended to use acquire-release instead unless there is a specific performance benefit and the semantics are well understood.
In conclusion, choosing the appropriate memory ordering constraint is crucial for ensuring the correctness of concurrent programs. Stronger constraints provide more guarantees but may introduce unnecessary overhead, while weaker constraints offer better performance but require more careful programming to avoid correctness issues.
Which memory ordering constraint should be used for atomic operations in a specific use case?
The choice of memory ordering constraint for atomic operations depends on the specific requirements of the use case, balancing correctness and performance. Here are some guidelines for selecting the appropriate constraint:
- Sequential Consistency (SC): Use SC when the program requires the strongest possible guarantees about the order of operations across all threads. This is suitable for scenarios where the exact order of operations is critical, such as in certain distributed systems or when debugging concurrent code. However, be aware that SC can introduce significant performance overhead.
- Acquire-Release (AR): This is often the best choice for many common synchronization patterns, such as locks, semaphores, and condition variables. Use AR when you need to ensure that operations before a release are visible to operations after an acquire. This model provides a good balance between correctness and performance for most concurrent algorithms.
- Relaxed Ordering: Use relaxed ordering for operations where the exact order is not important, such as counters or other accumulative operations. This can significantly improve performance but should only be used when the lack of ordering guarantees will not affect the correctness of the program.
- Consume Ordering: This should be used cautiously and only when there is a specific performance benefit and the semantics are well understood. It is generally recommended to use acquire-release instead, as consume ordering can be complex and may not be supported consistently across different hardware.
Example Use Case:
Consider a scenario where you are implementing a simple counter that is incremented by multiple threads. If the exact order of increments is not important, and you only need the final value, you could use relaxed ordering for the atomic increment operation. This would provide the best performance.
However, if you are implementing a lock mechanism where one thread needs to ensure that all its previous operations are visible to another thread before releasing the lock, you should use acquire-release semantics. The thread acquiring the lock would use an acquire operation, and the thread releasing the lock would use a release operation.
In summary, the choice of memory ordering constraint should be based on the specific requirements of the use case, considering both the correctness of the concurrent behavior and the performance implications.
The above is the detailed content of What are the different memory ordering constraints available for atomic operations?. For more information, please follow other related articles on the PHP Chinese website!
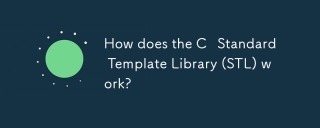
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
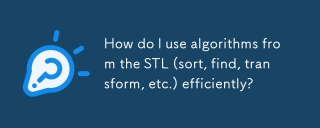
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
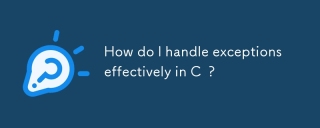
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
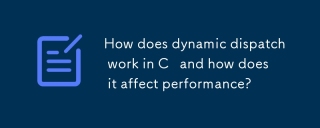
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
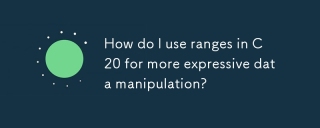
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
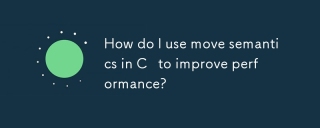
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
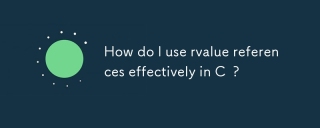
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
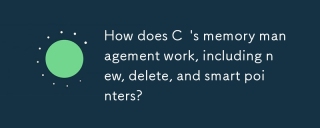
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
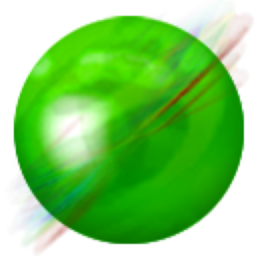
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
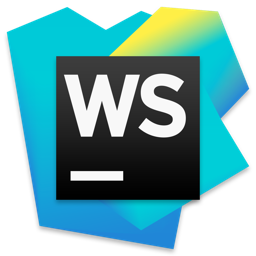
WebStorm Mac version
Useful JavaScript development tools

Notepad++7.3.1
Easy-to-use and free code editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.