


How can you achieve true parallelism in Python, given the GIL?
The Global Interpreter Lock (GIL) in Python poses a significant challenge to achieving true parallelism, as it allows only one thread to execute Python bytecode at a time, effectively preventing multi-threading from utilizing multiple CPU cores for CPU-bound tasks. However, there are several strategies to achieve true parallelism despite the GIL:
-
Multiprocessing: By using the
multiprocessing
module, you can create separate Python processes, which are not constrained by the GIL. Each process has its own Python interpreter and memory space, allowing them to run in parallel and utilize multiple CPU cores. - Third-party implementations: Some Python implementations like Jython and IronPython do not have a GIL, allowing true multi-threading. These can be used as alternatives to CPython, the standard implementation, to achieve parallelism.
-
External libraries and tools: Libraries such as
numba
andCython
allow you to compile Python code to native machine code, bypassing the GIL for certain sections of code. Additionally, usingasyncio
withasyncio.run_in_executor
can manage I/O-bound tasks efficiently. -
GPU acceleration: Libraries such as
PyCUDA
orPyOpenCL
can leverage GPUs for parallel processing, effectively sidestepping the GIL for certain types of computations.
By leveraging these strategies, developers can overcome the limitations imposed by the GIL and achieve true parallelism in Python.
What alternatives to the GIL can be used to achieve true parallelism in Python?
While the GIL is a central component of CPython, there are several alternatives and strategies that can be employed to achieve true parallelism in Python:
-
Alternative Python Implementations:
- Jython: Runs on the Java Virtual Machine (JVM) and does not have a GIL, allowing true multi-threading.
- IronPython: Runs on the .NET Common Language Runtime and also does not have a GIL.
- PyPy: While it has a GIL, it includes a Just-In-Time (JIT) compiler that can optimize certain types of operations, and its STM (Software Transactional Memory) branch offers experimental GIL-free execution.
-
Using Native Extensions:
- Cython: By compiling Python-like code to C, you can create extensions that run without the GIL and can achieve true parallelism.
- Numba: This library compiles Python and NumPy code to native machine instructions, which can bypass the GIL and use multiple cores effectively.
-
Multiprocessing:
- The
multiprocessing
module in Python provides an API similar tothreading
but spawns new Python processes, which are not subject to the GIL.
- The
-
Asynchronous Programming:
- Libraries like
asyncio
and frameworks likeTwisted
orTornado
use event loops and cooperative multitasking, which can handle high concurrency for I/O-bound tasks.
- Libraries like
-
GPU Computing:
- Libraries like
PyCUDA
andPyOpenCL
allow Python to offload computations to GPUs, achieving parallelism through GPU acceleration.
- Libraries like
These alternatives and strategies offer various paths to achieve true parallelism in Python without being hindered by the GIL.
How does using multiprocessing help bypass the GIL for true parallelism in Python?
Using the multiprocessing
module in Python is a powerful way to bypass the GIL and achieve true parallelism. Here’s how it works:
-
Separate Processes:
multiprocessing
creates separate Python processes, each of which runs its own Python interpreter. Since the GIL is per-interpreter, each process can execute Python code independently without being constrained by the GIL. - Parallel Execution: Each process can utilize a different CPU core, allowing for true parallelism. This means CPU-bound tasks can be distributed across multiple cores, resulting in significant performance improvements.
-
Communication and Synchronization:
multiprocessing
provides mechanisms like queues, pipes, and shared memory to facilitate communication and synchronization between processes. These features allow you to manage data exchange and task coordination effectively. -
API Similar to Threading: The
multiprocessing
module offers an API that is similar to thethreading
module, making it relatively easy for developers familiar with threading to transition to multiprocessing. This similarity includes features likeProcess
,Pool
, andManager
objects. -
Handling CPU-Bound Tasks: By splitting CPU-bound tasks across multiple processes, you can effectively utilize all available CPU cores. For instance, you can use
Pool
to create a pool of worker processes that can execute tasks in parallel.
Here's a simple example of using multiprocessing
to perform parallel computation:
from multiprocessing import Pool def square(x): return x * x if __name__ == '__main__': with Pool(4) as p: print(p.map(square, [1, 2, 3, 4]))
This example uses four processes to square numbers in parallel, bypassing the GIL and utilizing multiple CPU cores.
What are the best practices for managing memory when using multiprocessing to achieve parallelism in Python?
Effective memory management is crucial when using multiprocessing
for parallelism in Python. Here are some best practices:
-
Minimize Data Sharing:
- Avoid sharing large data structures between processes. Instead, pass data through inter-process communication (IPC) mechanisms like queues or pipes only when necessary.
- Use
multiprocessing.Array
ormultiprocessing.Value
for small, simple data that needs to be shared.
-
Use Pickling Wisely:
- Be mindful of pickling large objects, as it can be memory-intensive. If possible, use
multiprocessing.Pool
to limit the number of processes and control the size of the data being passed. - Consider using
dill
orcloudpickle
if standard pickling is insufficient for your use case.
- Be mindful of pickling large objects, as it can be memory-intensive. If possible, use
-
Control Process Creation:
- Limit the number of processes created to manage memory usage. Use
multiprocessing.Pool
with an appropriate number of worker processes based on available memory and CPU cores.
- Limit the number of processes created to manage memory usage. Use
-
Monitor Memory Usage:
- Use tools like
psutil
to monitor memory usage during execution and adjust your process pool size or data handling strategies accordingly.
- Use tools like
-
Optimize Data Transfers:
- Minimize the frequency and size of data transfers between processes. If possible, process data in smaller chunks.
- Use
multiprocessing.Manager
for shared objects, but be cautious as it can lead to higher memory usage due to the overhead of the manager process.
-
Clean Up Properly:
- Ensure proper cleanup of resources by using context managers or explicitly calling
terminate()
andjoin()
methods on processes to free up memory.
- Ensure proper cleanup of resources by using context managers or explicitly calling
-
Avoid Excessive Forking:
- In Unix-based systems, consider the memory overhead associated with forking. Forking a large memory space can lead to significant memory usage spikes.
-
Use Memory-Efficient Data Structures:
- Choose memory-efficient data structures and algorithms. For example, use
numpy
arrays instead of Python lists for large numerical data.
- Choose memory-efficient data structures and algorithms. For example, use
By following these best practices, you can efficiently manage memory when using multiprocessing
for parallel computing in Python, thus maximizing performance and minimizing resource consumption.
The above is the detailed content of How can you achieve true parallelism in Python, given the GIL?. For more information, please follow other related articles on the PHP Chinese website!
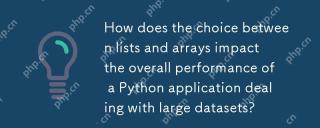
ForhandlinglargedatasetsinPython,useNumPyarraysforbetterperformance.1)NumPyarraysarememory-efficientandfasterfornumericaloperations.2)Avoidunnecessarytypeconversions.3)Leveragevectorizationforreducedtimecomplexity.4)Managememoryusagewithefficientdata
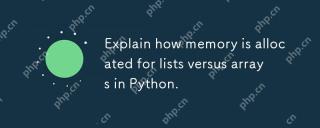
InPython,listsusedynamicmemoryallocationwithover-allocation,whileNumPyarraysallocatefixedmemory.1)Listsallocatemorememorythanneededinitially,resizingwhennecessary.2)NumPyarraysallocateexactmemoryforelements,offeringpredictableusagebutlessflexibility.
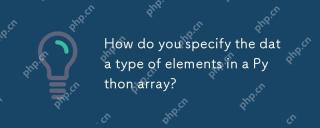
InPython, YouCansSpectHedatatYPeyFeLeMeReModelerErnSpAnT.1) UsenPyNeRnRump.1) UsenPyNeRp.DLOATP.PLOATM64, Formor PrecisconTrolatatypes.
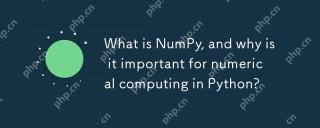
NumPyisessentialfornumericalcomputinginPythonduetoitsspeed,memoryefficiency,andcomprehensivemathematicalfunctions.1)It'sfastbecauseitperformsoperationsinC.2)NumPyarraysaremorememory-efficientthanPythonlists.3)Itoffersawiderangeofmathematicaloperation
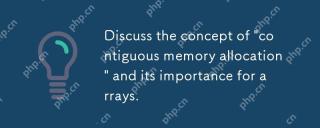
Contiguousmemoryallocationiscrucialforarraysbecauseitallowsforefficientandfastelementaccess.1)Itenablesconstanttimeaccess,O(1),duetodirectaddresscalculation.2)Itimprovescacheefficiencybyallowingmultipleelementfetchespercacheline.3)Itsimplifiesmemorym
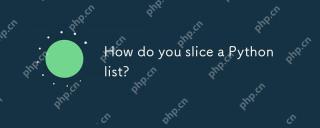
SlicingaPythonlistisdoneusingthesyntaxlist[start:stop:step].Here'showitworks:1)Startistheindexofthefirstelementtoinclude.2)Stopistheindexofthefirstelementtoexclude.3)Stepistheincrementbetweenelements.It'susefulforextractingportionsoflistsandcanuseneg

NumPyallowsforvariousoperationsonarrays:1)Basicarithmeticlikeaddition,subtraction,multiplication,anddivision;2)Advancedoperationssuchasmatrixmultiplication;3)Element-wiseoperationswithoutexplicitloops;4)Arrayindexingandslicingfordatamanipulation;5)Ag
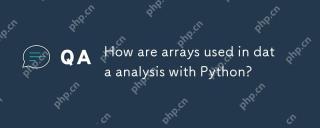
ArraysinPython,particularlythroughNumPyandPandas,areessentialfordataanalysis,offeringspeedandefficiency.1)NumPyarraysenableefficienthandlingoflargedatasetsandcomplexoperationslikemovingaverages.2)PandasextendsNumPy'scapabilitieswithDataFramesforstruc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
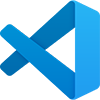
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
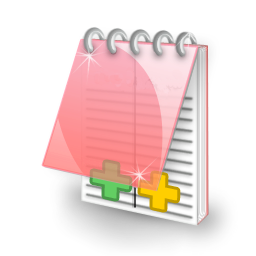
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
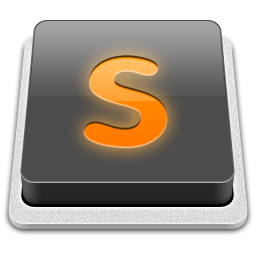
SublimeText3 Mac version
God-level code editing software (SublimeText3)
