


What are computed properties in Vue.js? How are they different from methods?
Computed properties in Vue.js are a feature that allows you to create and use properties in your Vue instance that are derived from other data in the component. They are defined in the computed
option of a Vue component and are essentially functions that Vue.js runs and caches based on their reactive dependencies. When the data these properties depend on changes, the computed property is re-evaluated and updated.
The key difference between computed properties and methods lies in their reactivity and caching behavior. Computed properties are cached based on their dependencies, meaning if the dependency hasn't changed, Vue.js will return the cached result rather than recalculating it. This makes computed properties very efficient for operations that depend on other reactive data.
In contrast, methods in Vue.js are functions defined within the methods
option of a component. They are called every time they are used, and they do not cache their results. This means that a method will be re-executed every time it's called, regardless of whether the underlying data has changed. This can be less efficient for operations that depend on data that doesn't change frequently.
What benefits do computed properties offer in terms of performance in Vue.js applications?
Computed properties offer several performance benefits in Vue.js applications:
- Caching: As mentioned, computed properties are cached based on their reactive dependencies. This means that Vue.js will not recompute the property if its dependencies haven't changed, reducing unnecessary computations and improving performance, especially in complex applications with frequent updates.
- Reactivity: Computed properties automatically update when their dependencies change, without needing manual intervention. This reactivity ensures that your application stays up-to-date and responsive, while still being efficient.
- Optimization of Render Functions: Because computed properties are reactive and cached, they can help optimize the rendering process. When a component re-renders, Vue.js can use the cached value of a computed property instead of recalculating it, which can speed up the rendering of components.
- Simplified Code: Using computed properties can often result in cleaner and more maintainable code. By encapsulating complex calculations within a computed property, you can simplify your template logic and make your components easier to understand and maintain, which indirectly improves performance by reducing the chance of errors and making the code easier to optimize.
How can you use computed properties to manage complex data dependencies in Vue.js?
Computed properties are particularly useful for managing complex data dependencies in Vue.js. Here’s how you can use them effectively:
-
Deriving Data: You can use computed properties to derive new data from existing data. For example, if you have a list of items and need to display the total price of all items, you can create a computed property that calculates this total based on the individual item prices and quantities.
computed: { totalPrice() { return this.items.reduce((total, item) => total item.price * item.quantity, 0); } }
-
Filtering and Sorting: Computed properties can be used to filter or sort data dynamically. For instance, if you have a list of products and want to show only the ones that are in stock, you can create a computed property that filters the list.
computed: { inStockProducts() { return this.products.filter(product => product.stock > 0); } }
-
Combining Multiple Data Sources: If your component depends on multiple data sources, computed properties can combine these sources into a single, usable format. For example, if you have user data and product data, you could create a computed property that merges these into a single data structure for easier manipulation in your template.
computed: { userProducts() { return this.products.map(product => ({ ...product, isPurchased: this.userPurchases.includes(product.id) })); } }
-
Conditional Logic: Computed properties can be used to handle conditional logic based on reactive data. For example, you might want to show different content based on the user's role.
computed: { userContent() { if (this.userRole === 'admin') { return this.adminContent; } else { return this.userContent; } } }
Can you explain a practical example where using a computed property would be more suitable than a method in Vue.js?
Consider a scenario where you're building an e-commerce application with Vue.js, and you need to display a list of products sorted by price. Additionally, you want to ensure that the sorting is only recalculated when the list of products or their prices change.
In this case, using a computed property would be more suitable than a method for the following reasons:
- Efficiency: If you use a method to sort the products, it will be called every time the component re-renders, even if the product list or their prices haven't changed. This could result in unnecessary sorting operations, especially if the sorting is computationally expensive.
- Reactivity: A computed property will automatically recalculate only when its dependencies (the products or their prices) change. This ensures that the sorting operation is only performed when necessary, improving performance.
Here's how you could implement this using a computed property:
new Vue({ el: '#app', data: { products: [ { id: 1, name: 'Product A', price: 100 }, { id: 2, name: 'Product B', price: 200 }, { id: 3, name: 'Product C', price: 50 }, // more products... ] }, computed: { sortedProducts() { return this.products.slice().sort((a, b) => a.price - b.price); } } })
In the template, you can then use sortedProducts
to display the sorted list of products:
<div id="app"> <ul> <li v-for="product in sortedProducts" :key="product.id"> {{ product.name }} - ${{ product.price }} </li> </ul> </div>
In this example, sortedProducts
is a computed property that depends on the products
array. Vue.js will automatically re-calculate sortedProducts
whenever products
or the prices within it change, but will otherwise use the cached result, making it more efficient than using a method for the same purpose.
The above is the detailed content of What are computed properties in Vue.js? How are they different from methods?. For more information, please follow other related articles on the PHP Chinese website!
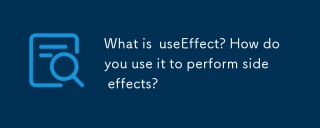
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
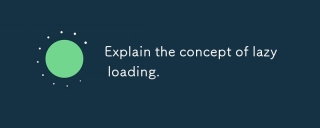
Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
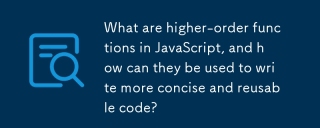
Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
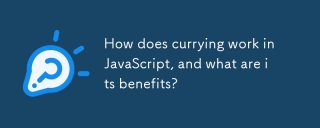
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
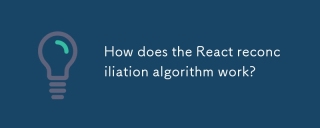
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
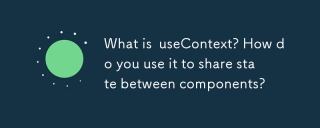
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
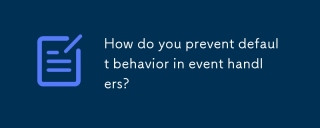
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
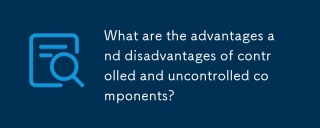
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
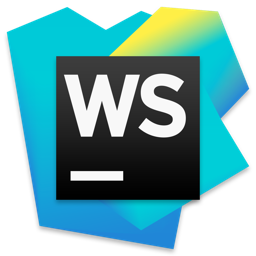
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
