How can you customize v-model for your own components?
Customizing v-model
for your own components in Vue.js allows you to create reusable and interactive components that can be easily integrated into your application. To customize v-model
, you need to understand how it works under the hood. v-model
is essentially syntactic sugar for a combination of v-bind
and v-on
directives. By default, v-model
on a component uses value
as the prop and input
as the event to update the parent's data.
To customize v-model
, you can specify different prop and event names using the model
option in your component's options object. Here's how you can do it:
-
Define the
model
option: In your component's options, you can specify themodel
object to define the prop and event names thatv-model
should use. For example:export default { model: { prop: 'myValue', event: 'change' }, props: { myValue: String }, methods: { updateValue(newValue) { this.$emit('change', newValue); } } }
-
Use the custom prop and emit the custom event: In your component's template, you can then use the custom prop and emit the custom event when the value changes. For example:
<template> <input :value="myValue" @input="updateValue($event.target.value)" /> </template>
By following these steps, you can customize v-model
to use different prop and event names that suit your component's needs.
What are the steps to implement a custom v-model in a Vue.js component?
Implementing a custom v-model
in a Vue.js component involves several steps to ensure that it works seamlessly with the parent component. Here are the detailed steps:
-
Define the
model
option: In your component's options, specify themodel
object to define the prop and event names thatv-model
should use. For example:export default { model: { prop: 'customValue', event: 'update' }, props: { customValue: { type: String, default: '' } } }
-
Create a method to update the value: Define a method in your component that will emit the custom event with the new value. For example:
methods: { updateCustomValue(newValue) { this.$emit('update', newValue); } }
-
Use the custom prop and emit the custom event in the template: In your component's template, bind the custom prop to an input element and call the method to emit the custom event when the value changes. For example:
<template> <input :value="customValue" @input="updateCustomValue($event.target.value)" /> </template>
-
Use the custom v-model in the parent component: In the parent component, you can now use the custom
v-model
with your component. For example:<template> <my-component v-model="parentValue"></my-component> </template>
By following these steps, you can implement a custom v-model
that works seamlessly with your component and the parent component.
How can you ensure two-way data binding with a custom v-model?
Ensuring two-way data binding with a custom v-model
involves making sure that changes in the parent component's data are reflected in the child component, and vice versa. Here's how you can achieve this:
-
Use the
model
option correctly: Define themodel
option in your component's options to specify the prop and event names thatv-model
should use. For example:export default { model: { prop: 'customValue', event: 'update' }, props: { customValue: { type: String, default: '' } } }
-
Emit the custom event with the new value: When the value in the child component changes, emit the custom event with the new value. For example:
methods: { updateCustomValue(newValue) { this.$emit('update', newValue); } }
-
Bind the custom prop and emit the custom event in the template: In your component's template, bind the custom prop to an input element and call the method to emit the custom event when the value changes. For example:
<template> <input :value="customValue" @input="updateCustomValue($event.target.value)" /> </template>
-
Use the custom v-model in the parent component: In the parent component, use the custom
v-model
with your component. This ensures that changes in the parent's data are reflected in the child component, and changes in the child component are reflected in the parent's data. For example:<template> <my-component v-model="parentValue"></my-component> </template>
By following these steps, you can ensure two-way data binding with a custom v-model
, allowing seamless communication between the parent and child components.
What are common pitfalls to avoid when customizing v-model for components?
When customizing v-model
for components, there are several common pitfalls to avoid to ensure that your components work correctly and efficiently. Here are some of them:
-
Incorrect
model
option: One of the most common pitfalls is incorrectly defining themodel
option. Make sure that theprop
andevent
names in themodel
option match the ones you use in your component. For example:export default { model: { prop: 'customValue', event: 'update' }, props: { customValue: String } }
If the
prop
andevent
names do not match, the customv-model
will not work as expected. -
Not emitting the correct event: Another common pitfall is not emitting the correct event when the value changes. Make sure that you emit the event specified in the
model
option with the new value. For example:methods: { updateCustomValue(newValue) { this.$emit('update', newValue); } }
If you emit the wrong event or do not emit the event at all, the parent component will not be updated.
-
Not using the correct prop: Make sure that you use the correct prop in your component's template. For example:
<template> <input :value="customValue" @input="updateCustomValue($event.target.value)" /> </template>
If you use the wrong prop, the initial value from the parent component will not be reflected in the child component.
-
Not handling edge cases: Consider edge cases such as null or undefined values. Make sure that your component can handle these cases gracefully. For example:
props: { customValue: { type: String, default: '' } }
By handling edge cases, you can ensure that your component works correctly in all scenarios.
-
Overcomplicating the implementation: Keep the implementation of your custom
v-model
as simple as possible. Overcomplicating the implementation can lead to bugs and make your component harder to maintain. Stick to the basic principles of using themodel
option, emitting the correct event, and using the correct prop.
By avoiding these common pitfalls, you can ensure that your custom v-model
works correctly and efficiently, providing a seamless user experience.
The above is the detailed content of How can you customize v-model for your own components?. For more information, please follow other related articles on the PHP Chinese website!
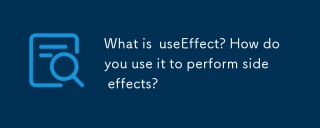
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
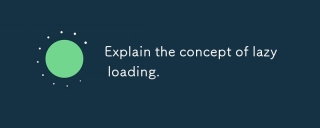
Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
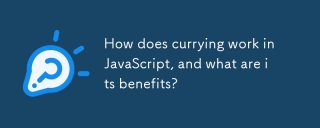
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
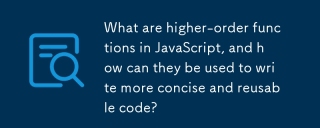
Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
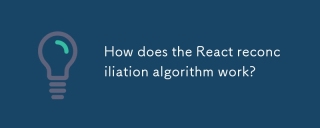
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
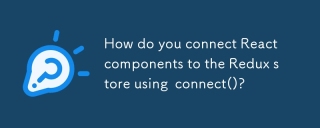
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.
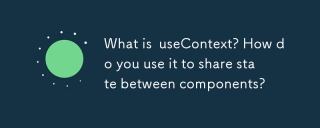
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
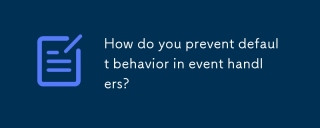
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
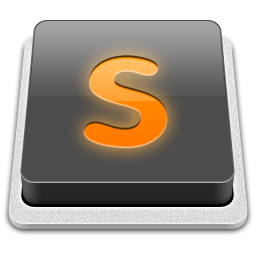
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
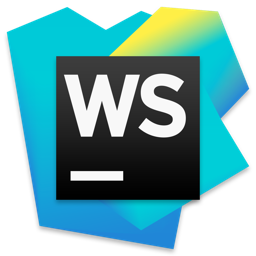
WebStorm Mac version
Useful JavaScript development tools
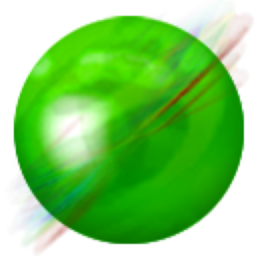
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.