


Explain the difference between make and new in Go. When would you use each?
In Go, make
and new
are both used for memory allocation, but they serve different purposes and are used with different types.
-
new: The
new
function allocates memory for a given type and returns a pointer to that memory. The memory is initialized to zero values for that type.new
is used with any type, including built-in types, structs, and custom types. It returns a pointer of type*T
to newly allocated zeroed storage for a new item of typeT
. For example:p := new(int) // p, of type *int, points to an unnamed int variable fmt.Println(*p) // prints 0
You would use
new
when you need a pointer to a zeroed value of a type, particularly when you're working with structs or other composite types where you want to ensure all fields are initialized to their zero values. -
make: The
make
function is used only with the built-in typesslice
,map
, andchannel
. Unlikenew
,make
initializes these types to non-zero values. It returns an initialized (not zeroed) value of typeT
(not*T
), which is ready to use. For example:s := make([]int, 5) // s is a slice of ints, length 5, capacity 5 m := make(map[string]int) // m is a map of strings to ints c := make(chan int) // c is a channel of ints
You would use
make
when you're initializing slices, maps, or channels. These types need special initialization and are not merely allocated with zero values.
What types of data structures are best initialized with 'make' in Go?
In Go, the make
function is specifically designed for initializing the following three data structures:
-
Slices: Slices are dynamic arrays that can grow or shrink in size. When you use
make
with a slice, you specify the length and optionally the capacity. This initializes the slice with zero values for its elements.mySlice := make([]int, 5, 10) // length 5, capacity 10
-
Maps: Maps are key-value pairs where keys are unique. Using <code>make with a map initializes an empty map ready to store key-value pairs.
myMap := make(map[string]int)
-
Channels: Channels are the conduits through which you can send and receive values with the channel operator
. Using <code>make
with a channel initializes an empty channel that can be used for communication between goroutines.myChannel := make(chan int)
How does memory allocation differ between 'make' and 'new' in Go?
Memory allocation in Go differs between make
and new
in the following ways:
-
new: When you use
new
, Go allocates memory for the type you specify and returns a pointer to that memory. The memory is initialized to the zero value for the specified type.new
essentially performs a simple memory allocation without any additional initialization beyond setting zero values. It is generic and works with any type.p := new(int) // allocates memory and returns a pointer to zeroed int
-
make: When you use
make
, Go not only allocates memory but also initializes the data structure. For slices,make
allocates an underlying array of the specified length and capacity, and the slice is initialized with zero values. For maps and channels,make
performs necessary internal initialization to make them ready to use.make
only works with slices, maps, and channels.s := make([]int, 5) // allocates memory for a slice and initializes it
In summary, new
allocates memory and returns a pointer to zeroed storage, whereas make
allocates memory and initializes the specified data structure (slice, map, or channel) to a ready-to-use state.
In what scenarios might 'new' be more appropriate than 'make' in Go?
new
might be more appropriate than make
in the following scenarios:
-
When working with custom types or structs: If you're defining a custom type or struct and need a pointer to a zeroed instance of that type,
new
is the appropriate choice. This is often useful for initializing objects before you fill in their fields.type Person struct { Name string Age int } p := new(Person) // p is of type *Person, points to zeroed Person struct p.Name = "Alice" p.Age = 30
-
When you need a pointer to any type: If you need a pointer to a basic type like
int
,float64
, etc., or to a custom type where you want the zero value,new
is suitable. This can be useful in scenarios where you're working with pointers directly.pi := new(int) // pi is of type *int, points to zeroed int *pi = 42
-
For efficient zero-value initialization: When you want to efficiently allocate and zero-initialize memory for a type without the overhead of additional setup that
make
performs for slices, maps, and channels,new
is more appropriate.zeroInt := new(int) // Efficient way to get a zeroed int pointer
-
For compatibility with older code or libraries: In some cases, older Go code or libraries might expect pointers to types, and
new
can be used to satisfy these expectations.
In summary, new
is more appropriate when you need a pointer to a zeroed value of any type, especially when working with custom types, structs, or basic types where you want to initialize to zero values before further manipulation.
The above is the detailed content of Explain the difference between make and new in Go. When would you use each?. For more information, please follow other related articles on the PHP Chinese website!
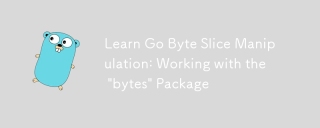
ThebytespackageinGoisessentialformanipulatingbytesliceseffectively.1)Usebytes.Jointoconcatenateslices.2)Employbytes.Bufferfordynamicdataconstruction.3)UtilizeIndexandContainsforsearching.4)ApplyReplaceandTrimformodifications.5)Usebytes.Splitforeffici
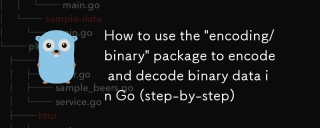
Tousethe"encoding/binary"packageinGoforencodinganddecodingbinarydata,followthesesteps:1)Importthepackageandcreateabuffer.2)Usebinary.Writetoencodedataintothebuffer,specifyingtheendianness.3)Usebinary.Readtodecodedatafromthebuffer,againspeci
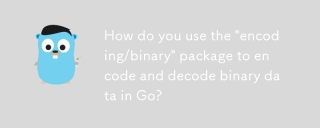
The encoding/binary package provides a unified way to process binary data. 1) Use binary.Write and binary.Read functions to encode and decode various data types such as integers and floating point numbers. 2) Custom types can be handled by implementing the binary.ByteOrder interface. 3) Pay attention to endianness selection, data alignment and error handling to ensure the correctness and efficiency of the data.

Go's strings package is not suitable for all use cases. It works for most common string operations, but third-party libraries may be required for complex NLP tasks, regular expression matching, and specific format parsing.
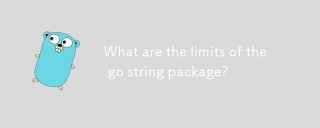
The strings package in Go has performance and memory usage limitations when handling large numbers of string operations. 1) Performance issues: For example, strings.Replace and strings.ReplaceAll are less efficient when dealing with large-scale string replacements. 2) Memory usage: Since the string is immutable, new objects will be generated every operation, resulting in an increase in memory consumption. 3) Unicode processing: It is not flexible enough when handling complex Unicode rules, and may require the help of other packages or libraries.
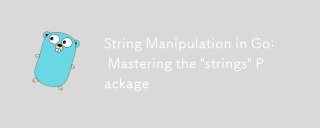
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
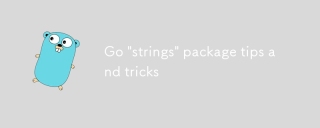
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
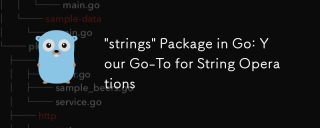
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
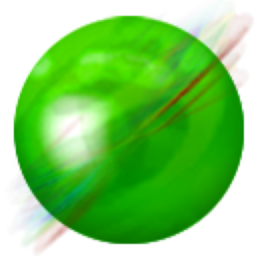
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
