What are some common Go code smells and how to avoid them?
Go code smells are indications of potential issues in code that may affect maintainability, readability, or performance. Here are some common Go code smells and ways to avoid them:
-
Long Functions or Methods:
- Smell: Functions or methods that are excessively long and complex, often performing multiple unrelated tasks.
- Avoidance: Break down long functions into smaller, more focused functions. Each function should ideally perform a single task, adhering to the Single Responsibility Principle.
-
Nested Code:
- Smell: Deeply nested conditional statements or loops that make the code hard to read and follow.
- Avoidance: Simplify logic where possible. Use guard clauses to exit functions early and reduce nesting. Consider extracting nested logic into separate functions.
-
Duplicated Code:
- Smell: Code that appears in multiple places within the project, leading to maintenance issues.
- Avoidance: Extract duplicated code into a common function or method that can be called from multiple places. Use generics where appropriate to reduce duplication across types.
-
Global Variables:
- Smell: Overuse of global variables can lead to tight coupling and make code harder to test and maintain.
- Avoidance: Minimize the use of global variables. Instead, pass variables as parameters to functions or use dependency injection to manage state.
-
Excessive Use of Interfaces:
- Smell: Defining too many interfaces, which can lead to over-abstraction and complexity.
- Avoidance: Use interfaces judiciously. Only define interfaces when they provide a clear benefit, such as for dependency injection or when the interface is used by multiple types.
-
Ignoring Error Handling:
- Smell: Not properly handling or logging errors, which can lead to silent failures.
- Avoidance: Always handle errors explicitly. Use error wrapping and logging to provide context about where and why errors occur.
-
Inconsistent Naming:
- Smell: Inconsistent naming conventions that make the code harder to understand and maintain.
- Avoidance: Follow Go's naming conventions religiously. Use clear and descriptive names for variables, functions, and types.
By addressing these code smells, you can significantly improve the quality and maintainability of your Go code.
How can I refactor my Go code to improve its maintainability?
Refactoring Go code to improve maintainability involves several strategies:
-
Extract Functions:
- Break down large functions into smaller, more manageable ones. This improves readability and makes the code easier to test.
-
Simplify Conditional Logic:
- Use guard clauses to simplify conditional logic and reduce nesting. Early returns can help make the code flow more naturally.
-
Remove Duplication:
- Identify and extract duplicated code into reusable functions or methods. This not only reduces the amount of code but also makes it easier to maintain.
-
Improve Naming:
- Rename variables, functions, and types to be more descriptive and consistent with Go naming conventions. Good names can significantly improve code readability.
-
Use Interfaces Effectively:
- Use interfaces to define contracts and enable dependency injection. This makes the code more modular and easier to test.
-
Implement Error Handling Properly:
- Refactor error handling to be more explicit and informative. Use error wrapping to provide context about where errors occur.
-
Utilize Go's Concurrency Features:
- Refactor code to make use of goroutines and channels where appropriate. This can improve performance and make the code more Go-idiomatic.
-
Code Review and Testing:
- Regularly review code and write tests to ensure refactored code works as expected. Continuous testing helps maintain code quality and prevents regressions.
By applying these refactoring techniques, you can enhance the maintainability of your Go code, making it easier to understand, modify, and extend.
What tools can I use to detect code smells in Go projects?
Several tools can help you detect code smells in your Go projects:
-
Golangci-lint:
- Golangci-lint is a fast and highly configurable linter that aggregates multiple linters into one. It can detect a wide range of code smells, including issues with naming conventions, unused code, and complex functions.
-
Staticcheck:
- Staticcheck is another static analysis tool that can detect a variety of issues in Go code, including code smells. It is known for its detailed error messages and can help identify problems that other tools might miss.
-
Go Vet:
- Go Vet is a built-in tool that comes with the Go distribution. It detects suspicious constructs, such as printf calls whose arguments do not align with the format string, and can help identify some code smells.
-
Revive:
- Revive is a linter for Go that is highly customizable and can be used to enforce specific coding standards. It can detect code smells related to code complexity, naming, and more.
-
SonarQube:
- SonarQube is a comprehensive code quality and security platform that can be used with Go projects. It can detect code smells, bugs, and security vulnerabilities.
-
Code Climate:
- Code Climate provides automated code review for Go projects, helping you identify code smells and maintain high code quality standards.
Using these tools in combination can help you thoroughly analyze your Go code and detect a broad range of code smells, allowing you to improve the overall quality of your codebase.
What are the best practices for writing clean and efficient Go code?
Writing clean and efficient Go code involves following several best practices:
-
Follow Go Idioms:
- Adhere to Go's idioms and conventions, such as naming conventions, error handling, and use of goroutines and channels. This makes your code more readable and maintainable.
-
Keep Functions Small and Focused:
- Write functions that do one thing well. Smaller functions are easier to understand, test, and maintain.
-
Use Interfaces Judiciously:
- Define interfaces only when they provide a clear benefit, such as for dependency injection or when used by multiple types. Overusing interfaces can lead to unnecessary complexity.
-
Handle Errors Explicitly:
- Always handle errors explicitly. Use error wrapping to provide context about where errors occur and consider logging errors for debugging purposes.
-
Avoid Global Variables:
- Minimize the use of global variables. Instead, pass variables as parameters to functions or use dependency injection to manage state.
-
Utilize Go's Concurrency Features:
- Leverage Go's goroutines and channels to write concurrent code. This can improve performance and make your code more Go-idiomatic.
-
Write Tests:
- Write unit tests for your code to ensure it works as expected. Testing helps catch bugs early and makes it easier to refactor code safely.
-
Use Static Analysis Tools:
- Regularly use static analysis tools like Golangci-lint, Staticcheck, and Go Vet to detect code smells and maintain code quality.
-
Code Review:
- Conduct regular code reviews to ensure that the code adheres to best practices and to catch any issues early.
-
Optimize for Readability:
- Prioritize code readability. Clear and well-commented code is easier to maintain and extend.
By following these best practices, you can write Go code that is not only clean and efficient but also easy to maintain and extend over time.
The above is the detailed content of What are some common Go code smells and how to avoid them?. For more information, please follow other related articles on the PHP Chinese website!
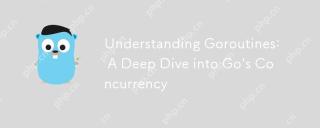
GoroutinesarefunctionsormethodsthatrunconcurrentlyinGo,enablingefficientandlightweightconcurrency.1)TheyaremanagedbyGo'sruntimeusingmultiplexing,allowingthousandstorunonfewerOSthreads.2)Goroutinesimproveperformancethrougheasytaskparallelizationandeff
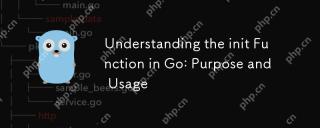
ThepurposeoftheinitfunctioninGoistoinitializevariables,setupconfigurations,orperformnecessarysetupbeforethemainfunctionexecutes.Useinitby:1)Placingitinyourcodetorunautomaticallybeforemain,2)Keepingitshortandfocusedonsimpletasks,3)Consideringusingexpl
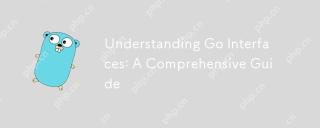
Gointerfacesaremethodsignaturesetsthattypesmustimplement,enablingpolymorphismwithoutinheritanceforcleaner,modularcode.Theyareimplicitlysatisfied,usefulforflexibleAPIsanddecoupling,butrequirecarefulusetoavoidruntimeerrorsandmaintaintypesafety.
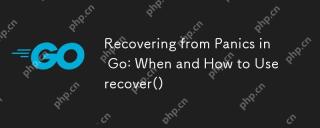
Use the recover() function in Go to recover from panic. The specific methods are: 1) Use recover() to capture panic in the defer function to avoid program crashes; 2) Record detailed error information for debugging; 3) Decide whether to resume program execution based on the specific situation; 4) Use with caution to avoid affecting performance.
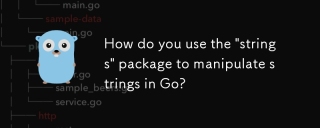
The article discusses using Go's "strings" package for string manipulation, detailing common functions and best practices to enhance efficiency and handle Unicode effectively.
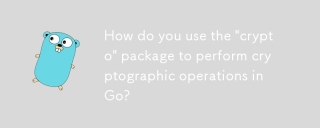
The article details using Go's "crypto" package for cryptographic operations, discussing key generation, management, and best practices for secure implementation.Character count: 159
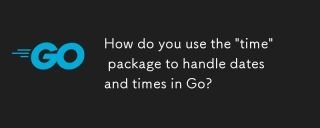
The article details the use of Go's "time" package for handling dates, times, and time zones, including getting current time, creating specific times, parsing strings, and measuring elapsed time.
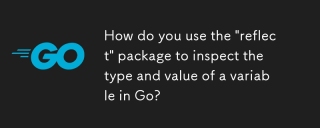
Article discusses using Go's "reflect" package for variable inspection and modification, highlighting methods and performance considerations.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
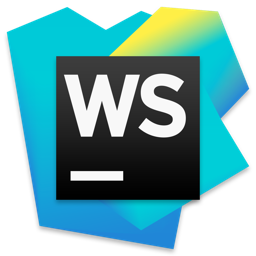
WebStorm Mac version
Useful JavaScript development tools
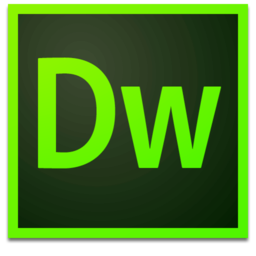
Dreamweaver Mac version
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment
