Explain the difference between template classes and template functions.
Template classes and template functions are both features of C that allow for generic programming, but they serve different purposes and have distinct characteristics.
- Template Classes: A template class is a class that can operate with generic types. It allows the definition of a class once and then instantiates it with different types. When a template class is used, the compiler generates a separate version of the class for each type it is instantiated with. The primary purpose of template classes is to define data structures or classes that can work with multiple data types without duplicating code. For example, a template class can be used to create a generic list that can store integers, floats, or user-defined types.
- Template Functions: A template function is a function that can be defined to operate on generic types. Like template classes, template functions can be instantiated with different types, and the compiler generates a separate version of the function for each type it is used with. The main use of template functions is to perform operations that are independent of the specific types involved. For example, a template function can be used to implement algorithms like finding the maximum of two values, regardless of the types of the values.
In summary, template classes are used to define generic data structures, while template functions are used to define generic algorithms or operations.
What are the benefits of using template classes over template functions in C programming?
Using template classes in C programming offers several benefits compared to using template functions:
-
Encapsulation: Template classes can encapsulate both data and operations on that data, providing a more comprehensive solution. For example, a template class like
std::vector
not only manages a dynamic array but also provides methods to operate on the array. - Code Organization: Template classes allow for better organization of related functionality. By grouping data and functions into a single class, the code becomes more structured and easier to maintain.
- Type Safety: Template classes can enforce type safety more effectively. For instance, they can ensure that only certain types are used with the class, or they can provide type-specific behavior through specialization.
- State Management: Template classes can maintain state, which is essential for complex data structures like linked lists, trees, or graphs. Template functions, on the other hand, are stateless and cannot manage persistent data.
- Operator Overloading: Template classes allow for the overloading of operators, which can make the use of the class more intuitive and aligned with the built-in types.
In summary, template classes are preferred when the solution requires managing data, maintaining state, and providing a structured approach to generic programming.
How do template functions enhance code reusability compared to template classes?
Template functions enhance code reusability in several ways compared to template classes:
- Lightweight Implementation: Template functions are generally smaller and more focused than template classes. They can be written to perform a specific task across different types without the overhead of managing data structures.
- Easier to Write and Maintain: Since template functions typically contain fewer lines of code than template classes, they are easier to write, understand, and maintain. This simplicity can lead to more reusable code.
- Immediate Applicability: Template functions can be used directly in code without the need to create instances of classes. This immediacy makes them very versatile and easy to integrate into existing codebases.
- Generic Algorithms: Template functions are particularly well-suited for implementing generic algorithms that can operate on different types. For example, a template function can be used to implement sorting algorithms that work on various data types, enhancing code reusability.
- No State Management: Since template functions do not manage state, they can be reused across different contexts without worrying about how state is maintained or changed.
In summary, template functions enhance code reusability by providing a lightweight, focused, and versatile way to implement generic algorithms and operations.
In what scenarios would you choose to use a template class instead of a template function?
There are several scenarios where it would be more appropriate to use a template class instead of a template function:
- Complex Data Structures: When you need to implement a complex data structure like a binary tree, linked list, or graph, a template class is the better choice. These structures require not only operations but also data management, which a template class can handle effectively.
- Maintaining State: If your solution requires maintaining state over time, a template class is necessary. For example, a template class can be used to create a generic stack or queue that manages its own data.
- Encapsulation of Data and Operations: When you need to encapsulate both data and the operations that manipulate that data, a template class is the right tool. This is common in scenarios like creating a generic container class.
- Type-Specific Behavior: If you need to provide type-specific behavior through template specialization, a template class is more appropriate. This allows for custom behavior for certain types while maintaining a generic interface.
-
Operator Overloading: When you want to enable the use of operators like
-
,==
, or!=
with your generic type, a template class can implement these operators, enhancing the usability of the class.
In summary, you should choose a template class over a template function when you need to manage complex data structures, maintain state, encapsulate data and operations, provide type-specific behavior, or enable operator overloading.
The above is the detailed content of Explain the difference between template classes and template functions.. For more information, please follow other related articles on the PHP Chinese website!
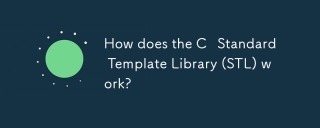
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
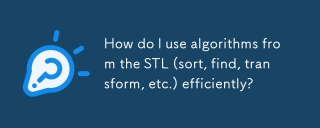
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
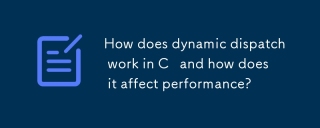
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
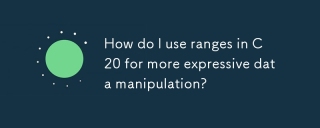
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
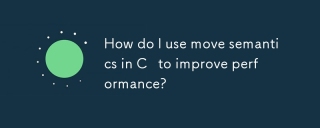
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
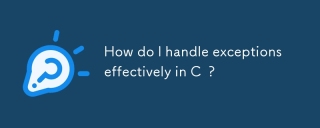
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
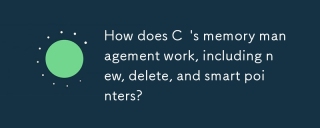
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
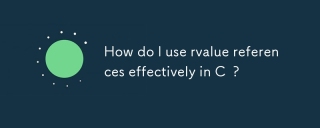
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment
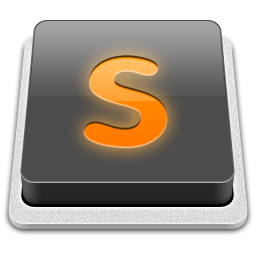
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
