What is the Redux middleware? How do you use it?
Redux middleware serves as a critical component in the Redux ecosystem, enhancing the capabilities of Redux by allowing developers to intercept, modify, or augment the normal action dispatch cycle. Essentially, Redux middleware sits between the dispatching of an action and the moment it reaches the reducer. It offers a third-party extension point between dispatching an action and the moment it reaches the reducer.
To use Redux middleware, you typically integrate it into your Redux store using the applyMiddleware
function from the Redux library. Here is a basic example of how to use middleware in setting up a Redux store:
import { createStore, applyMiddleware } from 'redux'; import thunk from 'redux-thunk'; import rootReducer from './reducers'; const store = createStore( rootReducer, applyMiddleware(thunk) ); export default store;
In this example, redux-thunk
is a commonly used middleware that allows you to write action creators that return a function instead of an action. This function can then be used to dispatch multiple actions asynchronously.
What benefits does Redux middleware provide in managing application state?
Redux middleware provides several benefits in managing application state:
-
Asynchronous Operations: Middleware like
redux-thunk
orredux-saga
allows you to handle asynchronous operations such as API calls, ensuring that the application state can be updated once data is received. -
Logging and Debugging: Middleware like
redux-logger
provides a way to log dispatched actions and state changes, which can be invaluable for debugging complex state changes. - Side Effects Management: Middleware can manage side effects, such as making API requests or setting timers, which is crucial for real-world applications where state changes are often coupled with external operations.
- Modularity and Reusability: By using middleware, you can modularize your state management logic, making it easier to reuse across different parts of your application or even in different projects.
- Enhanced Control Over Action Flow: Middleware allows developers to have finer control over how actions are processed, enabling more complex state management scenarios, like conditional dispatching or handling action sequences.
How can you implement custom middleware in a Redux application?
To implement custom middleware in a Redux application, you need to create a function that adheres to the middleware signature. The function should return another function that takes next
as an argument, and this returned function should take action
as an argument. Here's a step-by-step guide to creating and using custom middleware:
-
Define the Middleware Function:
const customMiddleware = store => next => action => { console.log('Action type:', action.type, 'Payload:', action.payload); return next(action); };
-
Integrate the Middleware into the Store:
When creating the store, pass the custom middleware to
applyMiddleware
.import { createStore, applyMiddleware } from 'redux'; import rootReducer from './reducers'; const store = createStore( rootReducer, applyMiddleware(customMiddleware) ); export default store;
This example will log each action's type and payload before passing it to the next middleware or the reducer.
What are some popular Redux middleware libraries and their use cases?
There are several popular Redux middleware libraries, each with specific use cases:
-
Redux Thunk:
- Use Case: Handling asynchronous actions and side effects. It allows action creators to return functions that can dispatch multiple actions over time.
- Example Use: Making an API call and dispatching an action when the data is received.
-
Redux Saga:
- Use Case: Managing side effects and asynchronous flows using ES6 generators. It's ideal for complex asynchronous operations and managing global application states.
- Example Use: Handling user authentication flows, such as login, logout, and session refresh.
-
Redux Observable:
- Use Case: Managing side effects using reactive programming with RxJS. It's particularly useful for handling streams of data and complex event sequences.
- Example Use: Debouncing user inputs for search queries to prevent excessive API calls.
-
Redux Logger:
- Use Case: Logging actions, state changes, and the resulting state after each action, which is invaluable for debugging.
- Example Use: Tracking state changes during development to understand how actions affect the state.
-
Redux Promise Middleware:
- Use Case: Handling asynchronous actions that return promises, allowing you to dispatch actions based on promise resolution or rejection.
- Example Use: Dispatching success or failure actions after an API call's promise is resolved or rejected.
These middleware libraries help enhance the functionality of Redux, making it easier to manage state in complex applications.
The above is the detailed content of What is the Redux middleware? How do you use it?. For more information, please follow other related articles on the PHP Chinese website!

HTML and React can be seamlessly integrated through JSX to build an efficient user interface. 1) Embed HTML elements using JSX, 2) Optimize rendering performance using virtual DOM, 3) Manage and render HTML structures through componentization. This integration method is not only intuitive, but also improves application performance.

React efficiently renders data through state and props, and handles user events through the synthesis event system. 1) Use useState to manage state, such as the counter example. 2) Event processing is implemented by adding functions in JSX, such as button clicks. 3) The key attribute is required to render the list, such as the TodoList component. 4) For form processing, useState and e.preventDefault(), such as Form components.

React interacts with the server through HTTP requests to obtain, send, update and delete data. 1) User operation triggers events, 2) Initiate HTTP requests, 3) Process server responses, 4) Update component status and re-render.

React is a JavaScript library for building user interfaces that improves efficiency through component development and virtual DOM. 1. Components and JSX: Use JSX syntax to define components to enhance code intuitiveness and quality. 2. Virtual DOM and Rendering: Optimize rendering performance through virtual DOM and diff algorithms. 3. State management and Hooks: Hooks such as useState and useEffect simplify state management and side effects handling. 4. Example of usage: From basic forms to advanced global state management, use the ContextAPI. 5. Common errors and debugging: Avoid improper state management and component update problems, and use ReactDevTools to debug. 6. Performance optimization and optimality

Reactisafrontendlibrary,focusedonbuildinguserinterfaces.ItmanagesUIstateandupdatesefficientlyusingavirtualDOM,andinteractswithbackendservicesviaAPIsfordatahandling,butdoesnotprocessorstoredataitself.

React can be embedded in HTML to enhance or completely rewrite traditional HTML pages. 1) The basic steps to using React include adding a root div in HTML and rendering the React component via ReactDOM.render(). 2) More advanced applications include using useState to manage state and implement complex UI interactions such as counters and to-do lists. 3) Optimization and best practices include code segmentation, lazy loading and using React.memo and useMemo to improve performance. Through these methods, developers can leverage the power of React to build dynamic and responsive user interfaces.

React is a JavaScript library for building modern front-end applications. 1. It uses componentized and virtual DOM to optimize performance. 2. Components use JSX to define, state and attributes to manage data. 3. Hooks simplify life cycle management. 4. Use ContextAPI to manage global status. 5. Common errors require debugging status updates and life cycles. 6. Optimization techniques include Memoization, code splitting and virtual scrolling.

React's future will focus on the ultimate in component development, performance optimization and deep integration with other technology stacks. 1) React will further simplify the creation and management of components and promote the ultimate in component development. 2) Performance optimization will become the focus, especially in large applications. 3) React will be deeply integrated with technologies such as GraphQL and TypeScript to improve the development experience.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
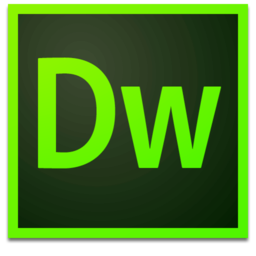
Dreamweaver Mac version
Visual web development tools
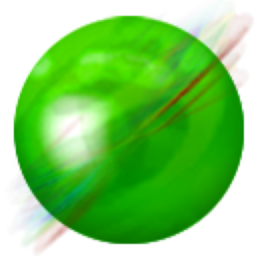
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software