How do you create a Pandas DataFrame from a CSV file?
To create a Pandas DataFrame from a CSV file, you will primarily use the pandas.read_csv()
function. This function is part of the Pandas library in Python, which is extensively used for data manipulation and analysis. Here’s a step-by-step guide on how to do it:
-
Install Pandas: First, ensure that you have Pandas installed. You can install it using pip if you haven't already:
<code>pip install pandas</code>
-
Import Pandas: Next, import the Pandas library into your Python script or Jupyter notebook:
import pandas as pd
-
Read the CSV File: Use the
read_csv()
function to read the CSV file into a DataFrame. You need to provide the file path as an argument:df = pd.read_csv('path_to_your_file.csv')
Replace
'path_to_your_file.csv'
with the actual path to your CSV file. -
Explore the DataFrame: After loading the data, you can start exploring it using various Pandas functions. For example:
print(df.head()) # Displays the first few rows of the DataFrame print(df.info()) # Shows information about the DataFrame, including column data types and non-null counts
This basic procedure allows you to create a DataFrame from a CSV file. The flexibility of pd.read_csv()
includes numerous parameters to handle various data formats and issues, which we will discuss in the following sections.
What are the common parameters used when reading a CSV file into a Pandas DataFrame?
When using pd.read_csv()
, there are several commonly used parameters that enhance the flexibility and control over how the CSV file is read into a DataFrame. Here are some of the most used ones:
-
sep
ordelimiter
: Specifies the delimiter used in the CSV file. By default, it is set to','
, but you can change it to another character if needed, like'\t'
for tab-separated values. -
header
: Specifies which row to use as the column names. It defaults to0
, meaning the first row is used. You can set it toNone
if your CSV file doesn't have a header row. -
names
: Used to specify column names if the CSV file does not have a header. It should be a list of strings. -
index_col
: Specifies which column to use as the index of the DataFrame. Can be a single column name or a list of column names for a multi-index. -
usecols
: Specifies which columns to read, which can be useful for handling large datasets. You can pass a list of column names or indices. -
dtype
: Specifies the data type for one or more columns. It can be a dictionary mapping column names to data types. -
na_values
: Specifies additional strings to recognize as NA/NaN. It can be a string or a list of strings. -
skiprows
: Specifies rows to skip at the beginning of the file, can be an integer or a list of integers. -
nrows
: Limits the number of rows to read from the file, useful for reading a subset of a large file. -
encoding
: Specifies the encoding used to decode the file, such as'utf-8'
or'latin1'
.
These parameters allow you to tailor the reading process to meet your specific data requirements, ensuring that the data is imported correctly into your DataFrame.
How can you handle missing data when importing a CSV file into a Pandas DataFrame?
Handling missing data effectively is crucial when importing a CSV file into a Pandas DataFrame. Pandas provides various methods to manage and manipulate missing values during the import process:
-
Identifying Missing Values: By default, Pandas recognizes common representations of missing data, such as
NaN
,NA
, or empty strings. You can also specify additional strings to be recognized as missing using thena_values
parameter:df = pd.read_csv('path_to_your_file.csv', na_values=['', 'NA', 'n/a', 'None'])
-
Filling Missing Values: Once the DataFrame is created, you can use methods like
fillna()
to replace missing data with a specific value, the mean, median, or any other calculation:df['column_name'].fillna(df['column_name'].mean(), inplace=True)
-
Dropping Missing Values: If rows or columns with missing values are not useful, you can drop them using
dropna()
:df.dropna(inplace=True) # Drops rows with any missing values df.dropna(axis=1, inplace=True) # Drops columns with any missing values
-
Interpolation: For numerical data, Pandas supports interpolation of missing values using the
interpolate()
method:df['column_name'].interpolate(inplace=True)
By using these methods strategically, you can effectively manage missing data when importing and processing a CSV file into a Pandas DataFrame.
What options are available for specifying the data types of columns when reading a CSV file into a Pandas DataFrame?
Pandas allows you to explicitly set the data types of columns when reading a CSV file, which can be crucial for performance and data integrity. Here are the options available for specifying data types:
-
dtype
Parameter: You can pass a dictionary to thedtype
parameter ofread_csv()
to specify the data type for each column. For example:df = pd.read_csv('path_to_your_file.csv', dtype={'column_name': 'int64', 'another_column': 'float64'})
-
Converters: If you need more control over the conversion of specific columns, you can use the
converters
parameter. This allows you to define custom functions to convert data:df = pd.read_csv('path_to_your_file.csv', converters={'date_column': pd.to_datetime})
-
parse_dates
Parameter: This parameter allows you to specify columns that should be parsed as datetime objects. It can be a list of column names or a dictionary mapping column names to a format:df = pd.read_csv('path_to_your_file.csv', parse_dates=['date_column']) df = pd.read_csv('path_to_your_file.csv', parse_dates={'date_time': ['date', 'time']})
-
After Import: If you prefer to handle data type conversion after the import, you can use the
astype()
method on the DataFrame:df['column_name'] = df['column_name'].astype('float64')
Using these options allows you to ensure that the data is read into your DataFrame with the correct data types, which can improve the efficiency of subsequent data operations and ensure data integrity.
The above is the detailed content of How do you create a Pandas DataFrame from a CSV file?. For more information, please follow other related articles on the PHP Chinese website!
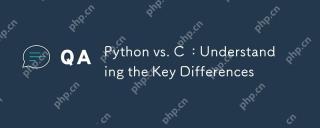
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
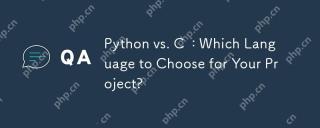
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
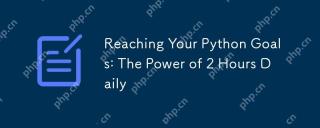
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.
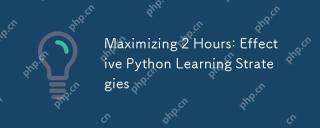
Methods to learn Python efficiently within two hours include: 1. Review the basic knowledge and ensure that you are familiar with Python installation and basic syntax; 2. Understand the core concepts of Python, such as variables, lists, functions, etc.; 3. Master basic and advanced usage by using examples; 4. Learn common errors and debugging techniques; 5. Apply performance optimization and best practices, such as using list comprehensions and following the PEP8 style guide.

Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
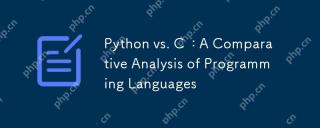
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
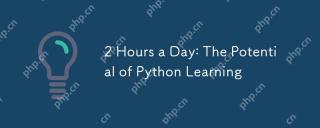
It is feasible to invest two hours a day to learn Python. 1. Learn new knowledge: Learn new concepts in one hour, such as lists and dictionaries. 2. Practice and exercises: Use one hour to perform programming exercises, such as writing small programs. Through reasonable planning and perseverance, you can master the core concepts of Python in a short time.
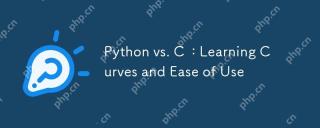
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
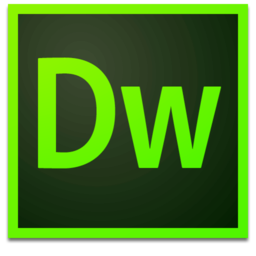
Dreamweaver Mac version
Visual web development tools
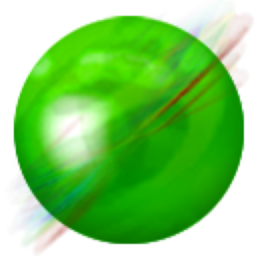
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software