What are metaclasses in Python?
In Python, metaclasses are a powerful and advanced feature that allows developers to customize the creation of classes. Essentially, metaclasses are classes for classes. Just as classes define the behavior of objects, metaclasses define the behavior of classes. They are responsible for creating classes and can modify their structure or behavior before they are created.
In Python, the default metaclass is type
. When you define a class in Python, type
is used to create the class object. You can think of metaclasses as factories for classes, where you can alter the way classes are constructed, add methods or attributes, and even change the inheritance hierarchy.
To define a metaclass, you create a class that inherits from type
. Here's a simple example of defining a metaclass:
class MyMeta(type): def __new__(cls, name, bases, dct): # Custom logic for class creation print(f"Creating class {name}") return super().__new__(cls, name, bases, dct) class MyClass(metaclass=MyMeta): pass
In this example, MyMeta
is a metaclass that prints a message when a class is being created. MyClass
uses MyMeta
as its metaclass.
What is the role of metaclasses in customizing class creation?
Metaclasses play a crucial role in customizing class creation by allowing you to define how classes are constructed. They can modify the class dictionary (dct
), the base classes (bases
), and other aspects of the class before it is instantiated. Here are some ways metaclasses can customize class creation:
- Adding Methods or Attributes: Metaclasses can dynamically add methods or attributes to classes. This can be useful for adding utility methods that are common across multiple classes.
-
Modifying Inheritance: Metaclasses can change the inheritance hierarchy by modifying the
bases
argument passed to__new__
. This can be used to enforce specific inheritance patterns. - Validating Class Definitions: Metaclasses can check the class definition for specific requirements or constraints, such as ensuring certain methods or attributes are present.
- Class Registration: Metaclasses can be used to register classes in some global registry, which can be useful in frameworks or when building plugin systems.
Here's an example of a metaclass that adds a method to the class:
class AddMethodMeta(type): def __new__(cls, name, bases, dct): def new_method(self): return f"Hello from {name}" dct['new_method'] = new_method return super().__new__(cls, name, bases, dct) class MyClass(metaclass=AddMethodMeta): pass obj = MyClass() print(obj.new_method()) # Output: Hello from MyClass
In this example, the AddMethodMeta
metaclass adds a new_method
to MyClass
.
How can metaclasses be used to implement singleton patterns in Python?
The Singleton pattern ensures that only one instance of a class is created, and provides a global point of access to that instance. Metaclasses can be used to implement the Singleton pattern by controlling the instantiation process of classes. Here's how you can implement a Singleton using a metaclass:
class SingletonMeta(type): _instances = {} def __call__(cls, *args, **kwargs): if cls not in cls._instances: cls._instances[cls] = super().__call__(*args, **kwargs) return cls._instances[cls] class MyClass(metaclass=SingletonMeta): def __init__(self, value): self.value = value obj1 = MyClass(1) obj2 = MyClass(2) print(obj1.value) # Output: 1 print(obj2.value) # Output: 1 print(obj1 is obj2) # Output: True
In this example, SingletonMeta
ensures that only one instance of MyClass
is created. The __call__
method is overridden to check if an instance of the class already exists. If it does, it returns the existing instance; otherwise, it creates a new instance and stores it.
What are some practical examples of using metaclasses in Python programming?
Metaclasses can be used in various practical scenarios in Python programming. Here are some examples:
- ORM (Object-Relational Mapping) Frameworks: Metaclasses are commonly used in ORM frameworks like SQLAlchemy to map class attributes to database columns. When you define a class, the metaclass can automatically set up the mapping between the class and the database table.
- Automatic Registration of Classes: In plugin systems or frameworks, metaclasses can be used to automatically register classes as they are defined. This can be useful for building extensible applications.
- Enforcing Design Patterns: Metaclasses can enforce specific design patterns, such as the Singleton pattern, as shown earlier. They can also ensure that classes follow certain structural requirements.
- Automating Method Creation: Metaclasses can be used to automatically create methods based on certain rules. For example, in a data processing library, you might use a metaclass to automatically generate methods for processing different data formats.
Here's an example of using a metaclass for automatic registration of classes:
class PluginRegistryMeta(type): registry = [] def __new__(cls, name, bases, dct): new_class = super().__new__(cls, name, bases, dct) cls.registry.append(new_class) return new_class class Plugin(metaclass=PluginRegistryMeta): pass class PluginA(Plugin): pass class PluginB(Plugin): pass for plugin in PluginRegistryMeta.registry: print(plugin.__name__)
In this example, PluginRegistryMeta
automatically registers all classes that use it as a metaclass in a registry. This can be useful for managing plugins in a system.
These examples demonstrate the versatility of metaclasses in Python and how they can be used to solve various programming challenges.
The above is the detailed content of What are metaclasses in Python?. For more information, please follow other related articles on the PHP Chinese website!
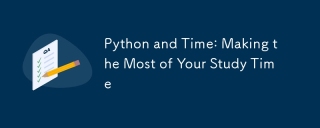
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
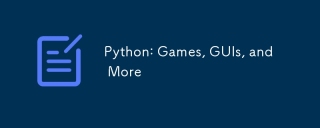
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
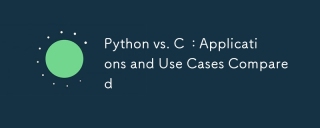
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
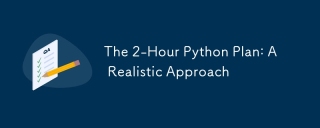
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
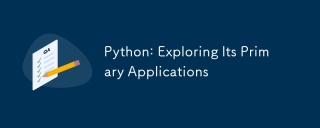
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
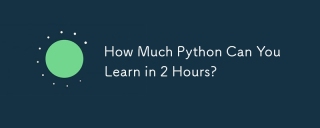
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
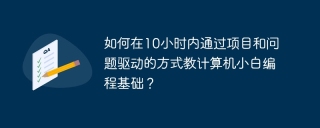
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
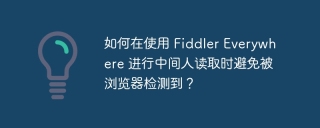
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.