


How do I customize the behavior of Bootstrap's JavaScript plugins?
Customizing the behavior of Bootstrap's JavaScript plugins involves several methods, each tailored to different needs and levels of modification. Here’s how you can do it:
-
Initialization Options: When initializing a Bootstrap plugin, you can pass an options object to modify its default behavior. For example, to initialize a modal with a custom backdrop, you could do the following:
$('#myModal').modal({ backdrop: 'static', keyboard: false });
This code sets the modal to have a static backdrop (which doesn't close when clicking outside the modal) and disables keyboard events.
-
Methods and Events: Bootstrap plugins provide methods and events that allow for dynamic interaction. You can use these to manipulate the plugin's state. For instance, to programmatically show and hide a modal:
$('#myModal').modal('show'); // Show the modal $('#myModal').modal('hide'); // Hide the modal
-
Data Attributes: Some configurations can be set directly via data attributes in HTML. For instance, to set a button to dismiss a modal:
<button type="button" class="btn btn-default" data-dismiss="modal">Close</button>
- JavaScript Overrides: For more advanced customizations, you might need to modify or extend the JavaScript itself. This could involve changing the plugin's source code or creating a custom plugin that inherits from the Bootstrap base.
By understanding and utilizing these techniques, you can effectively tailor Bootstrap's JavaScript plugins to fit your project's specific needs.
What are the available options for configuring Bootstrap's JS components?
Bootstrap provides a variety of options for configuring its JavaScript components. These options allow you to customize the behavior and appearance of components like modals, tooltips, popovers, and more. Here’s a list of some common configuration options for different components:
-
Modal:
-
backdrop
: Boolean or the string'static'
. Specifystatic
for a backdrop that doesn't close the modal on click. -
keyboard
: Boolean. Closes the modal when escape key is pressed. -
show
: Boolean. Shows the modal when initialized.
-
-
Tooltip:
-
animation
: Boolean. Apply a CSS fade transition to the tooltip. -
placement
: String or function. How to position the tooltip - top | bottom | left | right | auto. -
title
: String or function. Default title value iftitle
attribute isn't present.
-
-
Popover:
-
animation
: Boolean. Apply a CSS fade transition to the popover. -
placement
: String or function. How to position the popover - top | bottom | left | right | auto. -
content
: String or function. Default content value if thedata-content
attribute isn't present.
-
-
Carousel:
-
interval
: Number. The amount of time to delay between automatically cycling an item. If false, carousel will not automatically cycle. -
pause
: String or false. Pauses the cycling of the carousel on mouseenter and resumes the cycling on mouseleave. -
wrap
: Boolean. Whether the carousel should cycle continuously or have hard stops.
-
These are just examples, and each component has its own set of configurable options. You can find the full list of options in Bootstrap's documentation for each component.
How can I override default settings in Bootstrap's JavaScript plugins?
To override the default settings in Bootstrap's JavaScript plugins, you can use several approaches, each suited for different scenarios:
-
Initialization Options: As mentioned earlier, you can pass an options object to override defaults at the time of initialization. For example, to change the default behavior of a tooltip's animation:
$('#example').tooltip({ animation: false });
-
Global Defaults: Some plugins allow you to change global defaults that affect all instances of the component. For instance, to change the default placement for tooltips globally, you might do something like this:
$.fn.tooltip.Constructor.Default.placement = 'bottom';
- Customizing Source Code: For more profound changes, you might need to modify the source code of the plugin itself. This approach is more complex and less maintainable but can be necessary for advanced customizations. You could fork the Bootstrap repository, modify the JavaScript files, and then use your custom version.
-
Using Data Attributes: You can use data attributes in HTML to override default settings for individual instances. For example, to change a tooltip's placement for a specific element:
<a href="#" data-toggle="tooltip" data-placement="left" title="Hello World!">Hover over me</a>
By applying these methods, you can effectively override the default settings of Bootstrap's JavaScript plugins to meet your specific requirements.
Can I extend the functionality of Bootstrap's JS plugins to meet specific needs?
Yes, you can extend the functionality of Bootstrap's JavaScript plugins to meet specific needs. Bootstrap’s plugins are built with extensibility in mind, and there are several ways to do this:
-
Inheritance and Extension: You can create your own plugins that inherit from Bootstrap's base classes. For example, if you want to create a custom modal that includes additional features, you might start by extending the
Modal
class:var MyCustomModal = Modal.extend({ constructor: function(element, options) { Modal.call(this, element, options); // Additional initialization }, show: function() { Modal.prototype.show.call(this); // Additional logic when showing the modal } });
-
Event Hooks: Bootstrap plugins often trigger custom events at key points. You can use these events to hook into the plugin's lifecycle and extend its functionality. For instance, to add custom behavior when a modal is shown:
$('#myModal').on('shown.bs.modal', function (e) { // Your custom behavior });
-
Method Overriding: You can override existing methods of a plugin to change its behavior. This requires careful consideration to ensure you maintain the original functionality where needed:
var originalShowMethod = Modal.prototype.show; Modal.prototype.show = function() { // Your custom logic before showing the modal originalShowMethod.call(this); // Your custom logic after showing the modal };
-
Adding New Methods: You can also add new methods to existing plugins, allowing for new functionalities without altering the core behavior. For instance, adding a new method to the
Modal
class:Modal.prototype.myNewMethod = function() { // New functionality here };
By leveraging these extension techniques, you can tailor Bootstrap's JavaScript plugins to meet the unique requirements of your project, ensuring a high level of customization and flexibility.
The above is the detailed content of How do I customize the behavior of Bootstrap's JavaScript plugins?. For more information, please follow other related articles on the PHP Chinese website!

Bootstrap is a framework developed by Twitter to help quickly build responsive, mobile-first websites and applications. 1. Ease of use and rich component libraries make development faster. 2. The huge community provides support and solutions. 3. Introduce and use class names to control styles through CDN, such as creating responsive grids. 4. Customizable styles and extension components. 5. Advantages include rapid development and responsive design, while disadvantages are style consistency and learning curve.

Bootstrapisafree,open-sourceCSSframeworkthatsimplifiesresponsiveandmobile-firstwebsitedevelopment.Itofferspre-styledcomponentsandagridsystem,streamliningthecreationofaestheticallypleasingandfunctionalwebdesigns.

What makes web design easier is Bootstrap? Its preset components, responsive design and rich community support. 1) Preset component libraries and styles allow developers to avoid writing complex CSS code; 2) Built-in grid system simplifies the creation of responsive layouts; 3) Community support provides rich resources and solutions.

Bootstrap accelerates web development, and by providing predefined styles and components, developers can quickly build responsive websites. 1) It shortens development time, such as completing the basic layout within a few days in the project. 2) Through Sass variables and mixins, Bootstrap allows custom styles to meet specific needs. 3) Using the CDN version can optimize performance and improve loading speed.

Bootstrap is an open source front-end framework, and its main function is to help developers quickly build responsive websites. 1) It provides predefined CSS classes and JavaScript plug-ins to facilitate the implementation of complex UI effects. 2) The working principle of Bootstrap relies on its CSS and JavaScript components to realize responsive design through media queries. 3) Examples of usage include basic usage, such as creating buttons, and advanced usage, such as custom styles. 4) Common errors include misspelling of class names and incorrectly introducing files. It is recommended to use browser developer tools to debug. 5) Performance optimization can be achieved through custom build tools, best practices include predefined using semantic HTML and Bootstrap

Bootstrap implements responsive design through grid systems and media queries, making the website adapted to different devices. 1. Use a predefined class (such as col-sm-6) to define the column width. 2. The grid system is based on 12 columns, and it is necessary to note that the sum does not exceed 12. 3. Use breakpoints (such as sm, md, lg) to define the layout under different screen sizes.

Bootstrap is an open source front-end framework for rapid development of responsive websites and applications. 1. It provides the advantages of responsive design, consistent UI components and rapid development. 2. The grid system uses flexbox layout, based on 12-column structure, and is implemented through classes such as .container, .row and .col-sm-6. 3. Custom styles can be implemented by modifying SASS variables or overwriting CSS. 4. Commonly used JavaScript components include modal boxes, carousel diagrams and folding. 5. Optimization performance can be achieved by loading only necessary components, using CDN, and compressing merge files.

Bootstrap and JavaScript can be seamlessly integrated to give web pages dynamic functionality. 1) Use JavaScript to manipulate Bootstrap components, such as modal boxes and navigation bars. 2) Ensure jQuery loads correctly and avoid common integration problems. 3) Achieve complex user interaction and dynamic effects through event monitoring and DOM operations.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
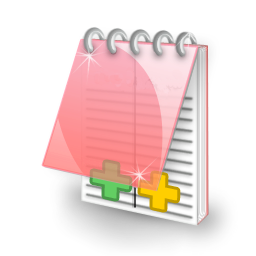
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
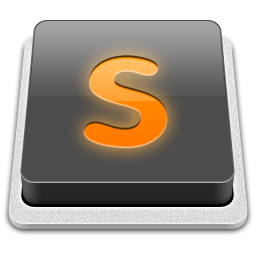
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.