How do I optimize Vue.js application bundle size for faster loading?
Optimizing the bundle size of a Vue.js application is crucial for enhancing the loading speed and overall user experience. Here are some steps you can take to achieve this:
-
Use Production Mode: Always build your application in production mode. This can be done using the
--mode production
flag when running your build command, which enables optimizations like minification and tree-shaking. - Leverage Tree-Shaking: Vue CLI uses webpack under the hood, which supports tree-shaking. This feature removes unused code from your bundle. Ensure that your code is written in a way that allows for effective tree-shaking, such as using ES6 module syntax.
- Minimize External Libraries: Only include necessary external libraries and consider using them via CDN to reduce the size of the main bundle.
-
Optimize Images: Use modern image formats like WebP and optimize images before bundling. Tools like
image-webpack-loader
can help automate this process. - Use Async Components: Implement asynchronous components to load components on demand, which can significantly reduce the initial bundle size.
- Remove Unused CSS: Use tools like PurgeCSS to remove unused styles from your CSS files.
-
Optimize Your Vue Build Configuration: Adjust your
vue.config.js
file to optimize various build parameters, such as setting the appropriateruntimeCompiler
andproductionSourceMap
options.
By applying these techniques, you can effectively reduce the size of your Vue.js application's bundle, leading to faster load times.
What are the best practices for reducing bundle size in Vue.js applications?
Reducing bundle size in Vue.js applications involves adhering to a set of best practices that can streamline your application and improve performance. Here are some key best practices:
- Avoid Global Components: Instead of registering components globally, register them locally where needed to prevent unnecessary imports.
- Use Lazy Loading: Implement lazy loading for routes and components to defer the loading of resources that are not immediately needed.
- Optimize Third-Party Dependencies: Evaluate and minimize the use of third-party libraries. If a library is large, consider using a subset of its features or finding a lighter alternative.
- Code Splitting: Utilize code splitting techniques to break your application into smaller chunks that can be loaded as needed.
- Minimize Vue Build Options: Disable unnecessary options in your Vue build configuration, such as source maps in production builds, to reduce bundle size.
- Use Modern JavaScript Features: Write your code using modern JavaScript features, which can help with tree-shaking and thus reduce the bundle size.
-
Regularly Audit Dependencies: Use tools like
npm ls
to keep track of your dependencies and remove any unused ones.
By following these best practices, you can significantly reduce the bundle size of your Vue.js applications, leading to faster loading times and a better user experience.
How can I use code splitting to improve the loading speed of my Vue.js app?
Code splitting is a powerful technique to improve the loading speed of your Vue.js application by splitting your code into smaller chunks that can be loaded on demand. Here's how you can implement code splitting in Vue.js:
-
Route-Based Code Splitting: Use dynamic imports in your route definitions to load components on demand. In your
router/index.js
, you can set up routes like this:const router = new VueRouter({ routes: [ { path: '/about', component: () => import(/* webpackChunkName: "about" */ '../views/About.vue') } ] })
This tells webpack to create a separate chunk for the
About
component, which will be loaded when the/about
route is accessed. -
Component-Based Code Splitting: For large components that are not immediately needed, you can use asynchronous components:
Vue.component('async-example', () => import('./AsyncComponent.vue'))
This will load
AsyncComponent.vue
only when it's actually used. -
Manual Code Splitting: You can manually split your code using webpack's
import()
function. For example:button.addEventListener('click', () => { import(/* webpackChunkName: "print" */ './print').then(module => { module.default() }) })
This will load the
print
module only when the button is clicked. -
Optimizing Code Splitting: Use webpack's optimization options, such as
splitChunks
, to further optimize how your code is split. In yourvue.config.js
, you can configure it like this:module.exports = { configureWebpack: { optimization: { splitChunks: { chunks: 'all' } } } }
This configuration will split all chunks, including initial and async chunks, potentially reducing the initial load time.
By effectively using code splitting, you can significantly enhance the loading speed of your Vue.js application, as users will only load the code they need when they need it.
What tools can help me analyze and minimize the bundle size of my Vue.js project?
There are several tools available that can help you analyze and minimize the bundle size of your Vue.js project. Here are some of the most effective ones:
-
Webpack Bundle Analyzer: This tool provides a visual representation of your bundle, showing the size of each module and how they contribute to the overall bundle size. It can be integrated into your Vue.js project by adding it to your
vue.config.js
:const BundleAnalyzerPlugin = require('webpack-bundle-analyzer').BundleAnalyzerPlugin module.exports = { configureWebpack: { plugins: [ new BundleAnalyzerPlugin() ] } }
Running your build command will then generate an interactive treemap that you can use to identify large chunks and dependencies.
- Source Map Explorer: This tool allows you to explore the contents of your source maps and see which files contribute most to your bundle size. It's particularly useful for identifying large, unnecessary dependencies.
- Bundlephobia: While not a tool that integrates directly with your project, Bundlephobia is a web-based tool that can help you estimate the size of npm packages before you decide to include them in your project.
-
PurgeCSS: This tool can be used to remove unused CSS from your project, which can significantly reduce the size of your bundle. You can integrate it with your Vue.js build process by adding it to your
vue.config.js
:const PurgecssPlugin = require('purgecss-webpack-plugin') const glob = require('glob-all') module.exports = { configureWebpack: { plugins: [ new PurgecssPlugin({ paths: glob.sync([ path.join(__dirname, './**/*.{vue,js,jsx,ts,tsx}'), path.join(__dirname, './public/index.html') ]) }) ] } }
- Size Limit: This tool allows you to set size limits for your bundle and fail the build if those limits are exceeded. It can be configured to run as part of your CI/CD pipeline.
By using these tools, you can gain insights into your bundle's composition, identify areas for optimization, and take concrete steps to minimize your Vue.js project's bundle size.
The above is the detailed content of How do I optimize Vue.js application bundle size for faster loading?. For more information, please follow other related articles on the PHP Chinese website!

Vue.js is a progressive framework suitable for building highly interactive user interfaces. Its core functions include responsive systems, component development and routing management. 1) The responsive system realizes data monitoring through Object.defineProperty or Proxy, and automatically updates the interface. 2) Component development allows the interface to be split into reusable modules. 3) VueRouter supports single-page applications to improve user experience.

The main disadvantages of Vue.js include: 1. The ecosystem is relatively new, and third-party libraries and tools are not as rich as other frameworks; 2. The learning curve becomes steep in complex functions; 3. Community support and resources are not as extensive as React and Angular; 4. Performance problems may be encountered in large applications; 5. Version upgrades and compatibility challenges are greater.

Netflix uses React as its front-end framework. 1.React's component development and virtual DOM mechanism improve performance and development efficiency. 2. Use Webpack and Babel to optimize code construction and deployment. 3. Use code segmentation, server-side rendering and caching strategies for performance optimization.

Reasons for Vue.js' popularity include simplicity and easy learning, flexibility and high performance. 1) Its progressive framework design is suitable for beginners to learn step by step. 2) Component-based development improves code maintainability and team collaboration efficiency. 3) Responsive systems and virtual DOM improve rendering performance.

Vue.js is easier to use and has a smooth learning curve, which is suitable for beginners; React has a steeper learning curve, but has strong flexibility, which is suitable for experienced developers. 1.Vue.js is easy to get started with through simple data binding and progressive design. 2.React requires understanding of virtual DOM and JSX, but provides higher flexibility and performance advantages.

Vue.js is suitable for fast development and small projects, while React is more suitable for large and complex projects. 1.Vue.js is simple and easy to learn, suitable for rapid development and small projects. 2.React is powerful and suitable for large and complex projects. 3. The progressive features of Vue.js are suitable for gradually introducing functions. 4. React's componentized and virtual DOM performs well when dealing with complex UI and data-intensive applications.

Vue.js and React each have their own advantages and disadvantages. When choosing, you need to comprehensively consider team skills, project size and performance requirements. 1) Vue.js is suitable for fast development and small projects, with a low learning curve, but deep nested objects can cause performance problems. 2) React is suitable for large and complex applications, with a rich ecosystem, but frequent updates may lead to performance bottlenecks.

Vue.js is suitable for small to medium-sized projects, while React is suitable for large projects and complex application scenarios. 1) Vue.js is easy to use and is suitable for rapid prototyping and small applications. 2) React has more advantages in handling complex state management and performance optimization, and is suitable for large projects.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
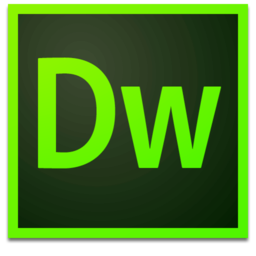
Dreamweaver Mac version
Visual web development tools
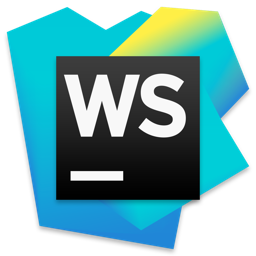
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
