How do I implement logging and monitoring in Swoole?
Implementing logging and monitoring in Swoole involves setting up both basic and advanced functionalities to track the performance and behavior of your application. Below is a step-by-step guide to get you started:
1. Basic Logging:
To implement basic logging in Swoole, you can use the built-in PHP logging capabilities or external libraries. Here's a simple example using PHP's built-in logging functions within a Swoole server:
$http = new Swoole\Http\Server("0.0.0.0", 9501); $http->on('request', function ($request, $response) { $logFile = 'swoole.log'; $logMessage = "New request received: " . date('Y-m-d H:i:s') . "\n"; file_put_contents($logFile, $logMessage, FILE_APPEND); $response->end("Hello World\n"); }); $http->start();
This code snippet logs every incoming request to a file named swoole.log
.
2. Advanced Logging with Libraries:
For more advanced logging needs, you might want to use a library such as Monolog, which is well-suited for Swoole applications due to its robust features and ease of integration:
use Monolog\Logger; use Monolog\Handler\StreamHandler; $logger = new Logger('swoole_app'); $logger->pushHandler(new StreamHandler('swoole_app.log', Logger::DEBUG)); $http = new Swoole\Http\Server("0.0.0.0", 9501); $http->on('request', function ($request, $response) use ($logger) { $logger->info('New request received', ['method' => $request->server['request_method'], 'uri' => $request->server['request_uri']]); $response->end("Hello World\n"); }); $http->start();
3. Monitoring:
For monitoring, you can use tools like Prometheus and Grafana to collect metrics and visualize the performance of your Swoole server. You'll need to install and configure the swoole/prometheus
library:
use Swoole\Prometheus\CollectorRegistry; use Swoole\Prometheus\MetricFamilySamples; $registry = new CollectorRegistry(); $http = new Swoole\Http\Server("0.0.0.0", 9501); $http->on('request', function ($request, $response) use ($registry) { $counter = $registry->getOrRegisterCounter('swoole_app', 'requests_total', 'Total number of requests'); $counter->inc(); $response->end("Hello World\n"); }); $http->on('start', function ($server) use ($registry) { swoole_timer_tick(1000, function () use ($registry, $server) { $exporter = new Swoole\Prometheus\Exporter($registry); $exporter->setListenAddress('0.0.0.0:9090'); $exporter->start(); }); }); $http->start();
This code sets up a Prometheus exporter to expose metrics about your Swoole server.
What are the best practices for setting up logging in Swoole applications?
Setting up logging in Swoole applications should follow these best practices:
1. Use Asynchronous Logging:
Swoole's event-driven architecture means you should avoid blocking operations as much as possible. Utilize asynchronous logging libraries to prevent I/O operations from impacting your server's performance. Monolog with the SwooleHandler
is an excellent choice for this.
2. Structured Logging:
Implement structured logging to make it easier to parse and analyze logs. JSON-formatted logs can be quickly ingested by various monitoring tools.
$logger->info('New request received', ['method' => $request->server['request_method'], 'uri' => $request->server['request_uri']]);
3. Log Levels and Rotation:
Use different log levels (e.g., DEBUG, INFO, WARNING, ERROR) to categorize logs based on severity. Implement log rotation to manage log file sizes and ensure older logs are archived or deleted to save disk space.
4. Contextual Information:
Include as much contextual information in your logs as possible, such as user IDs, request IDs, timestamps, and source IPs. This helps in tracing issues and understanding the flow of requests through your application.
5. Integration with Centralized Logging:
Integrate your Swoole application logs with centralized logging services like ELK (Elasticsearch, Logstash, Kibana) or AWS CloudWatch for better log management and analysis.
How can I use monitoring tools to improve the performance of my Swoole server?
Monitoring tools are crucial for optimizing and troubleshooting your Swoole server. Here are ways to leverage these tools:
1. Metrics Collection:
Use a metrics collector like Prometheus to gather key performance indicators (KPIs) such as request rate, response time, and error rate. Integrating Swoole with Prometheus involves:
- Installing and configuring
swoole/prometheus
- Exposing metrics in your Swoole application
- Setting up Prometheus to scrape these metrics
2. Visualization with Grafana:
After collecting metrics with Prometheus, use Grafana to create dashboards that visualize your server's performance. This helps in quickly identifying trends and issues.
3. Alerting:
Set up alerting rules in Prometheus to notify you when certain thresholds are breached. This can help you address issues before they impact your users.
4. Performance Tuning:
Use the data from your monitoring tools to identify bottlenecks and optimize your application:
- Request Latency: If certain endpoints are slow, you may need to optimize database queries or improve caching mechanisms.
- Error Rate: High error rates on specific endpoints indicate areas needing attention, perhaps through better error handling or code fixes.
- Resource Usage: Monitor CPU, memory, and network usage to understand and improve resource allocation.
5. Load Testing:
Integrate load testing tools like Apache JMeter or Locust with your monitoring tools to simulate high traffic scenarios and understand your server's performance under stress.
Which Swoole-specific libraries or tools should I use for effective logging and monitoring?
Here are several Swoole-specific libraries and tools that can help you with logging and monitoring effectively:
1. Monolog with SwooleHandler:
Monolog is a popular PHP logging library, and the SwooleHandler
specifically designed for Swoole helps in implementing non-blocking asynchronous logging.
use Monolog\Logger; use Monolog\Handler\SwooleHandler; $logger = new Logger('swoole_app'); $logger->pushHandler(new SwooleHandler('swoole_app.log'));
2. Swoole/Prometheus:
This library provides an exporter that can expose metrics from your Swoole application, making it compatible with Prometheus for monitoring and alerting.
use Swoole\Prometheus\CollectorRegistry; $registry = new CollectorRegistry();
3. Swoole/Tracer:
This tool can be used for distributed tracing, which is helpful for understanding the flow of requests through your Swoole application. It integrates well with systems like Jaeger for visualization.
use Swoole\Tracer\Tracer; $tracer = new Tracer();
4. Swoole/Elastic:
For integration with Elasticsearch, this library provides convenient ways to log data into Elasticsearch, which can be part of an ELK stack for centralized logging and monitoring.
use Swoole\Elastic\Elastic; $elastic = new Elastic('http://localhost:9200');
5. Swoole/Grafana:
Although Grafana itself is not Swoole-specific, combining it with Swoole/Prometheus to create detailed dashboards gives you powerful visualization and monitoring capabilities.
By using these tools and libraries, you can create a robust logging and monitoring system for your Swoole applications that helps maintain and improve performance.
The above is the detailed content of How do I implement logging and monitoring in Swoole?. For more information, please follow other related articles on the PHP Chinese website!
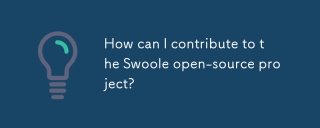
The article outlines ways to contribute to the Swoole project, including reporting bugs, submitting features, coding, and improving documentation. It discusses required skills and steps for beginners to start contributing, and how to find pressing is
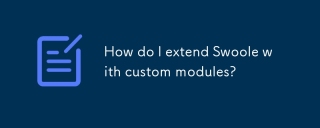
Article discusses extending Swoole with custom modules, detailing steps, best practices, and troubleshooting. Main focus is enhancing functionality and integration.
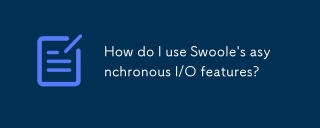
The article discusses using Swoole's asynchronous I/O features in PHP for high-performance applications. It covers installation, server setup, and optimization strategies.Word count: 159
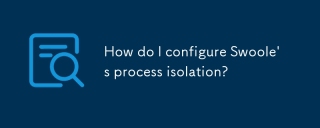
Article discusses configuring Swoole's process isolation, its benefits like improved stability and security, and troubleshooting methods.Character count: 159
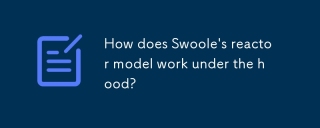
Swoole's reactor model uses an event-driven, non-blocking I/O architecture to efficiently manage high-concurrency scenarios, optimizing performance through various techniques.(159 characters)
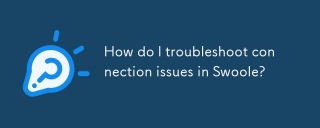
Article discusses troubleshooting, causes, monitoring, and prevention of connection issues in Swoole, a PHP framework.
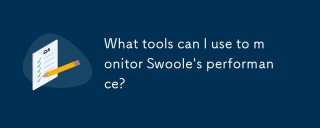
The article discusses tools and best practices for monitoring and optimizing Swoole's performance, and troubleshooting methods for performance issues.
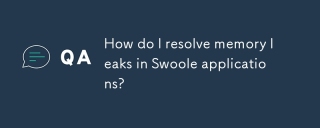
Abstract: The article discusses resolving memory leaks in Swoole applications through identification, isolation, and fixing, emphasizing common causes like improper resource management and unmanaged coroutines. Tools like Swoole Tracker and Valgrind


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
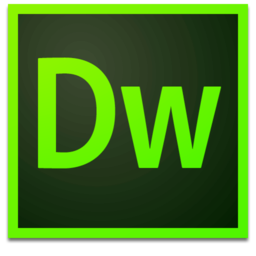
Dreamweaver Mac version
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
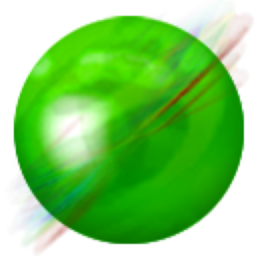
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!