How can I use Swoole's memory pool to reduce memory fragmentation?
To use Swoole's memory pool to reduce memory fragmentation, you need to understand how it operates and configure it appropriately for your application. Swoole's memory pool is designed to manage memory more efficiently by reducing the frequency of memory allocations and deallocations, which can lead to fragmentation over time.
-
Enable the Memory Pool: First, ensure that the memory pool is enabled in your Swoole server configuration. You can do this by setting the
use_memory_pool
option totrue
in your Swoole server settings:$server = new Swoole\Server("0.0.0.0", 9501, SWOOLE_PROCESS); $server->set([ 'use_memory_pool' => true, ]);
-
Proper Sizing: Allocate the memory pool with a size that suits your application's needs. If the pool is too small, it won't be effective, and if it's too large, it may waste resources. You can set the size of the memory pool using the
memory_pool_size
option:$server->set([ 'use_memory_pool' => true, 'memory_pool_size' => 64 * 1024 * 1024, // 64MB ]);
- Reuse Memory: Encourage your application to reuse memory within the pool. Instead of repeatedly allocating and freeing small chunks of memory, try to keep objects alive for reuse. For example, you can store frequently used data structures in the memory pool rather than recreating them on each request.
- Avoid Large Allocations: Try to keep allocations within the memory pool small and manageable. Large allocations might not fit within the pool and could lead to external fragmentation.
By following these steps, you can effectively utilize Swoole's memory pool to mitigate memory fragmentation.
What are the best practices for configuring Swoole's memory pool to optimize memory usage?
Configuring Swoole's memory pool properly can significantly optimize your application's memory usage. Here are some best practices:
- Determine the Right Size: Evaluate your application's memory usage patterns to determine the optimal size for the memory pool. Start with a smaller size and gradually increase it until you find the right balance. Monitor your application's performance to ensure the size is adequate without being overly large.
-
Use Multiple Pools: For larger applications, consider using multiple memory pools for different purposes. This can help isolate memory usage and prevent one part of the application from impacting others. You can configure multiple pools with different sizes:
$server->set([ 'use_memory_pool' => true, 'memory_pool_size' => 64 * 1024 * 1024, // 64MB for general use 'huge_page_size' => 128 * 1024 * 1024, // 128MB for larger allocations ]);
-
Adjust the Pool's Allocation Strategy: Swoole provides options to control the allocation strategy within the pool. The
memory_pool_trim
option allows you to control how often the memory pool is trimmed to release unused memory back to the system. Setting this to a lower value can help in freeing up memory more frequently:$server->set([ 'memory_pool_trim' => 10, // Trimming every 10 seconds ]);
- Monitor and Tune: Continuously monitor your application's memory usage and adjust the memory pool configuration as needed. This might involve tweaking the size of the pool, the frequency of trimming, or even the number of pools.
By following these best practices, you can configure Swoole's memory pool to achieve optimal memory usage and performance.
How does Swoole's memory pool help in managing memory allocation and deallocation?
Swoole's memory pool plays a crucial role in managing memory allocation and deallocation, primarily by reducing the overhead associated with these operations. Here's how it works:
- Pre-Allocated Memory: The memory pool pre-allocates a chunk of memory when the server starts. This chunk is divided into smaller blocks, which can be quickly allocated and deallocated without needing to interact with the operating system as frequently.
- Faster Allocation: When your application requests memory, the memory pool can allocate it from the pre-allocated chunk more quickly than if it had to request it from the OS. This reduces the time spent on memory operations and improves overall application performance.
- Reduced Fragmentation: By reusing memory within the pool, the memory pool helps reduce fragmentation. Instead of continuously allocating and freeing memory, which can lead to fragmented memory, the pool encourages reuse of existing memory blocks.
- Efficient Deallocation: Deallocating memory within the pool is also faster because it simply marks the memory block as available for reuse rather than returning it to the OS. This reduces the overhead of deallocation and helps maintain the pool in a healthy state.
- Controlled Memory Usage: The memory pool allows you to control memory usage more effectively by limiting the size of the pool. This can prevent your application from using too much memory and helps in managing resources more predictably.
Overall, Swoole's memory pool enhances memory management by providing a faster, more controlled, and less fragmented approach to memory allocation and deallocation.
Can I monitor and analyze the performance of Swoole's memory pool to further reduce fragmentation?
Yes, you can monitor and analyze the performance of Swoole's memory pool to identify potential issues and further reduce fragmentation. Here's how you can do it:
-
Use Swoole's Built-in Statistics: Swoole provides statistics that can be accessed through the
Swoole\Server::stats()
method. These statistics include information about memory usage, which can help you understand how the memory pool is performing:$stats = $server->stats(); echo "Memory usage: " . $stats['worker_memory_usage'] . " bytes\n";
- Third-Party Monitoring Tools: You can use third-party monitoring tools to get more detailed insights into your application's memory usage. Tools like Prometheus and Grafana can help you visualize and analyze memory pool performance over time.
-
Custom Logging and Metrics: Implement custom logging and metrics in your application to track memory pool usage. For example, you can log the size of allocations and deallocations to identify patterns that might lead to fragmentation:
function logMemoryOperation($operation, $size) { error_log("Memory $operation: $size bytes"); } // Use this in your code logMemoryOperation('allocate', 1024); logMemoryOperation('deallocate', 1024);
- Profiling Tools: Use profiling tools like Xdebug or Blackfire to get detailed information about memory usage within your application. These tools can help you identify memory-intensive operations and optimize them to reduce fragmentation.
- Analyze Memory Pool Configuration: Regularly review and analyze your memory pool configuration to ensure it's optimal for your application. Adjust the size of the pool, the frequency of trimming, and other settings based on your analysis.
By monitoring and analyzing the performance of Swoole's memory pool, you can gain insights into your application's memory usage and make informed decisions to further reduce fragmentation and optimize memory management.
The above is the detailed content of How can I use Swoole's memory pool to reduce memory fragmentation?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
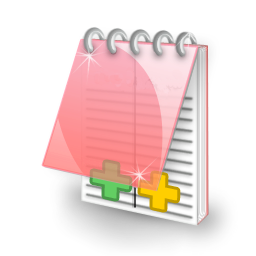
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment
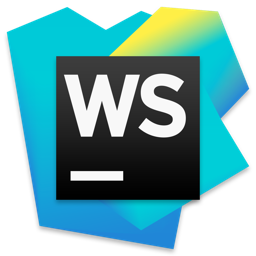
WebStorm Mac version
Useful JavaScript development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.