This tutorial will explain how to create pie, ring, and bubble charts using Chart.js. Previously, we have learned four chart types of Chart.js: line chart and bar chart (tutorial 2), as well as radar chart and polar region chart (tutorial 3).
Create pie and ring charts
Pie charts and ring charts are ideal for showing the proportions of a whole that is divided into different parts. For example, a pie chart can be used to show the percentage of male lions, female lions and young lions in a safari, or the percentage of votes that different candidates receive in the election.
Pie charts are only suitable for comparing single parameters or datasets. It should be noted that the pie chart cannot draw entities with zero value because the angle of the fan in the pie chart depends on the numerical size of the data point. This means that any entity with a proportion of zero will not be displayed on the chart. Similarly, negative values cannot be drawn on pie charts.
In Chart.js, you can create a pie chart by setting type
key to pie
, and a ring chart by setting type
key to doughnut
. Here is an example of creating both charts:
var pieChart = new Chart(votesCanvas, { type: 'pie', data: votesData, options: chartOptions }); var doughnutChart = new Chart(votesCanvas, { type: 'doughnut', data: votesData, options: chartOptions });
Let's create a pie chart showing oil export data (unit: billion US dollars) for the top five oil exporters in 2015.
var data = { labels: [ "Saudi Arabia", "Russia", "Iraq", "UAE", "Canada" ], datasets: [ { data: [133.3, 86.2, 52.2, 51.2, 50.2], backgroundColor: [ "#FF6384", "#63FF84", "#84FF63", "#8463FF", "#6384FF" ] }] };
You can use different keys to control the display of the above chart, such as cutout
, which can be a number or a string. If specified as a number, the value is considered a pixel value; if specified as a string ending in % , the value is considered a percentage of the total radius. You can use rotation
key to specify the starting angle of the chart. Similarly, you can also use circumference
key to specify the angle at which the chart scans when plotting the data. Both angles are expressed in degrees rather than radians.
Two different animations can be activated when drawing a chart. You can use the animateRotate
key to specify whether the chart should have a rotation animation. Similarly, you can also use the animateScale
key to specify whether the ring graph should be scaled from the center. The value of animateRotate
is true
by default, and the value of animateScale
is false
by default.
As usual, you can use backgroundColor
, borderColor
, and borderWidth
keys to control the background color, border color and border width of all data points, respectively. Likewise, the hover values for all these properties can be controlled using hoverBackgroundColor
, hoverBorderColor
, and hoverBorderWidth
keys.
The following is the code to create a ring graph for the above data. Set the value of rotation
to -90
Set the start point to rotate 90 degrees counterclockwise.
var oilData = { labels: ["Saudi Arabia", "Russia", "Iraq", "UAE", "Canada"], datasets: [ { data: [133.3, 86.2, 52.2, 51.2, 50.2], backgroundColor: ["#FF6384", "#63FF84", "#84FF63", "#8463FF", "#6384FF"], borderColor: "black", borderWidth: 2 } ] }; var chartOptions = { rotation: -90, cutout: "45%", plugins: { title: { display: true, position: "bottom", text: "Major oil exporters in 2015", font: { size: 32 } }, legend: { position: "left", align: "start" } }, animation: { animateRotate: false, animateScale: true } }; var donutChart = new Chart(oilCanvas, { type: "doughnut", data: oilData, options: chartOptions });
Create a bubble chart
Bubble charts are used to draw or display three dimensions of data on a chart ( p1 , p2 , p3 ). The position and size of the bubble determine the values of these three data points. The horizontal axis represents the first data point (p1), the vertical axis represents the second data point ( p2 ), and the area of the bubble is used to represent the value of the third data point ( p3 ).
It should be noted that the size of the third data point is not represented by the radius of the bubbles, but by their area. The area of the circle is proportional to the square of the radius. This means you have to make sure that the bubble radius you are drawing is proportional to the square root of the size of the third data point.
In Chart.js, you can create a bubble chart by setting the value of type
key to bubble
. Here is an example of how to create a bubble chart:
var bubbleChart = new Chart(popCanvas, { type: 'bubble', data: popData, options: chartOptions });
Let's use bubble charts to draw the weight of different items in the room. The data of the chart needs to be passed in the object format. The data object needs to have the following interface to draw correctly.
{ x:<number> , y:<number> , r:<number> }</number></number></number>
An important difference between a bubble chart and all other charts is that the bubble radius does not scale with the chart.
For example, the width of a bar in a bar chart increases or decreases according to the size of the chart. This won't happen with the bubble chart. The radius of the bubble is always equal to the exact number of pixels you specify. This makes sense because in this chart type, the radius is actually used to represent the real data.
Let's create a bubble chart to plot the number of deer herds at different locations in the forest.
var popData = { datasets: [ { label: ["Deer Herd"], data: [ { x: 100, y: 0, r: 10 }, { x: 60, y: 30, r: 20 }, { x: 40, y: 60, r: 25 }, { x: 80, y: 80, r: 30 }, { x: 20, y: 30, r: 25 }, { x: 0, y: 100, r: 5 } ], backgroundColor: "#FF9966" } ] };
Since the radius here is proportional to the square root of the actual number, the number of deer at (80, 80) is 36 times the number of deer at (0, 100).
Like all other chart types, you can use backgroundColor
, borderColor
, and borderWidth
keys to control the background color, border color, and border width of the drawn points. Similarly, you can also use hoverBackgroundColor
, hoverBorderColor
, and hoverBorderWidth
keys to specify the hover background color, hover border color, and hover border width.
You can also use hoverRadius
key to specify the extra radius to add to different bubbles when hovering. Remember that this radius will be added to the original value to draw the hovering bubble. If the radius of the original bubble is 10 and hoverRadius
is set to 5, the radius of the bubble on hover will be equal to 15.
var popData = { datasets: [ { label: ["Deer Herd"], data: [ { x: 100, y: 0, r: 10 }, { x: 60, y: 30, r: 20 }, { x: 40, y: 60, r: 25 } ], backgroundColor: "#9966FF", hoverBackgroundColor: "#FFFFF", hoverBorderColor: "#9966FF", hoverBorderWidth: 5, hoverRadius: 5 }, { label: ["Giraffe Number"], data: [ { x: 80, y: 80, r: 30 }, { x: 20, y: 30, r: 25 }, { x: 0, y: 100, r: 5 } ], backgroundColor: "#FF6600", hoverBackgroundColor: "#FFFFF", hoverBorderColor: "#FF6600", hoverBorderWidth: 5, hoverRadius: 5 } ] }; var chartOptions = { plugins: { title: { display: true, position: "bottom", text: "Number of animals in different hot spots", font: { size: 20 } }, legend: { position: "bottom", align: "center" } }, layout: { padding: 20 } };
The above parameters will create the following bubble chart.
Summarize
In this tutorial, you learned three other chart types available in Chart.js. You should now be able to select the appropriate chart type to plot the data and set specific values for different keys to control its appearance. In the next tutorial, you will learn how to manipulate scales for different chart types.
The above is the detailed content of Getting Started With Chart.js: Pie, Doughnut, and Bubble Charts. For more information, please follow other related articles on the PHP Chinese website!
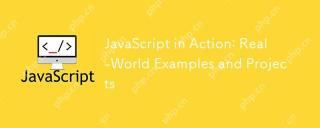
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
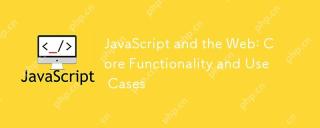
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
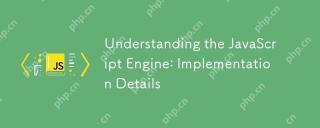
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
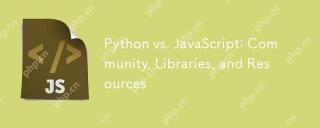
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
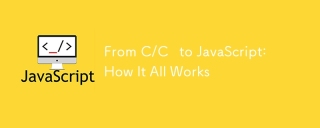
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
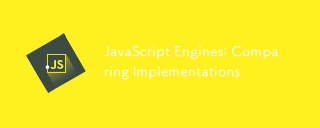
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
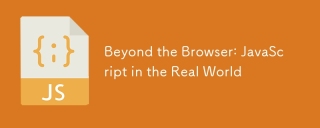
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
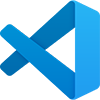
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft