


How does Laravel's service container work and how can I use it for dependency injection?
How does Laravel's service container work and how can I use it for dependency injection?
Laravel's service container is a powerful tool for managing class dependencies and performing dependency injection. It acts as a registry for dependencies and a method of resolving them when needed. Here's how it works and how you can use it:
Service Container Operation:
- Registration: You can register bindings (relationships between interfaces and their concrete implementations) in the container.
- Resolution: When a class is instantiated, the container will automatically resolve and inject any dependencies that are type-hinted in the class constructor.
Using for Dependency Injection:
To use the service container for dependency injection, you typically follow these steps:
-
Define your classes and interfaces: Start by defining your classes and interfaces. For example, you might have an interface
PaymentGateway
and a concrete implementationStripePaymentGateway
. -
Register bindings: In your service provider (usually
AppServiceProvider
), you bind the interface to the concrete implementation:public function register() { $this->app->bind(PaymentGateway::class, StripePaymentGateway::class); }
-
Type-hint dependencies: In the constructor of the class that needs the dependency, type-hint the interface:
class OrderController extends Controller { public function __construct(PaymentGateway $paymentGateway) { $this->paymentGateway = $paymentGateway; } }
When Laravel instantiates OrderController
, it will automatically resolve and inject an instance of StripePaymentGateway
because of the binding you set up.
What are the benefits of using Laravel's service container for managing dependencies in my application?
Using Laravel's service container for dependency management offers several benefits:
- Loose Coupling: By injecting interfaces instead of concrete implementations, your classes are loosely coupled, making it easier to change or swap implementations without modifying dependent classes.
- Testability: Dependency injection makes unit testing easier because you can easily mock dependencies.
- Flexibility: The service container allows you to change the behavior of your application at runtime by modifying bindings.
- Centralized Management: All dependency configurations are managed in one place (service providers), making it easier to maintain and understand your application's architecture.
- Automatic Resolution: Laravel automatically resolves dependencies, saving you from manually instantiating objects.
How can I bind interfaces to concrete implementations using Laravel's service container?
To bind interfaces to concrete implementations in Laravel's service container, you can use several methods, depending on your needs:
-
Simple Binding:
Use thebind
method in a service provider to bind an interface to a concrete class:$this->app->bind(InterfaceName::class, ConcreteImplementation::class);
-
Singleton Binding:
If you want a single instance of a class shared across your application, usesingleton
:$this->app->singleton(InterfaceName::class, ConcreteImplementation::class);
-
Instance Binding:
To bind an existing instance, useinstance
:$instance = new ConcreteImplementation(); $this->app->instance(InterfaceName::class, $instance);
-
Closure Binding:
For more complex scenarios, you can use a closure to define how the instance should be created:$this->app->bind(InterfaceName::class, function ($app) { return new ConcreteImplementation($app->make(Dependency::class)); });
These bindings are typically set up in the register
method of a service provider.
What best practices should I follow when utilizing Laravel's dependency injection features?
To make the most of Laravel's dependency injection features, follow these best practices:
- Use Interfaces: Define interfaces for your dependencies. This allows for greater flexibility and testability.
- Constructor Injection: Prefer constructor injection over setter injection. This makes dependencies explicit and easier to manage.
- Avoid Global State: Use dependency injection to avoid relying on global state or singletons, which can make your code harder to test and maintain.
- Keep Controllers Thin: Use dependency injection to keep your controllers focused on handling HTTP requests and responses, delegating business logic to injected services.
-
Organize Bindings: Keep your bindings organized by grouping related bindings in specific service providers (e.g., a
PaymentServiceProvider
for payment-related bindings). - Use Laravel's Facades Sparingly: While Laravel's facades are convenient, they can hide dependencies. Prefer explicit dependency injection where possible.
- Test Your Bindings: Ensure your bindings work as expected by writing unit tests that verify the correct instances are being injected.
- Document Your Bindings: Clearly document your bindings in your service providers, so other developers can understand the dependency structure of your application.
By following these practices, you'll create a more maintainable, testable, and flexible application using Laravel's powerful dependency injection system.
The above is the detailed content of How does Laravel's service container work and how can I use it for dependency injection?. For more information, please follow other related articles on the PHP Chinese website!

Laravel can be used for front-end development. 1) Use the Blade template engine to generate HTML. 2) Integrate Vite to manage front-end resources. 3) Build SPA, PWA or static website. 4) Combine routing, middleware and EloquentORM to create a complete web application.

PHP and Laravel can be used to build efficient server-side applications. 1.PHP is an open source scripting language suitable for web development. 2.Laravel provides routing, controller, EloquentORM, Blade template engine and other functions to simplify development. 3. Improve application performance and security through caching, code optimization and security measures. 4. Test and deployment strategies to ensure stable operation of applications.

Laravel and Python have their own advantages and disadvantages in terms of learning curve and ease of use. Laravel is suitable for rapid development of web applications. The learning curve is relatively flat, but it takes time to master advanced functions. Python's grammar is concise and the learning curve is flat, but dynamic type systems need to be cautious.

Laravel's advantages in back-end development include: 1) elegant syntax and EloquentORM simplify the development process; 2) rich ecosystem and active community support; 3) improved development efficiency and code quality. Laravel's design allows developers to develop more efficiently and improve code quality through its powerful features and tools.

Choosing Laravel or Python depends on the project requirements: 1) If the project is mainly web development and needs to quickly build complex applications, choose Laravel; 2) If data science, machine learning or more flexibility is involved, choose Python.

Laravel optimizes the web development process including: 1. Use the routing system to manage the URL structure; 2. Use the Blade template engine to simplify view development; 3. Handle time-consuming tasks through queues; 4. Use EloquentORM to simplify database operations; 5. Follow best practices to improve code quality and maintainability.

Laravel is a modern PHP framework that provides a powerful tool set, simplifies development processes and improves maintainability and scalability of code. 1) EloquentORM simplifies database operations; 2) Blade template engine makes front-end development intuitive; 3) Artisan command line tools improve development efficiency; 4) Performance optimization includes using EagerLoading, caching mechanism, following MVC architecture, queue processing and writing test cases.

Laravel's MVC architecture improves the structure and maintainability of the code through models, views, and controllers for separation of data logic, presentation and business processing. 1) The model processes data, 2) The view is responsible for display, 3) The controller processes user input and business logic. This architecture allows developers to focus on business logic and avoid falling into the quagmire of code.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
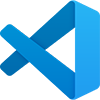
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft