


How can I protect my Yii application against cross-site scripting (XSS) attacks?
How can I protect my Yii application against cross-site scripting (XSS) attacks?
Protecting your Yii application against cross-site scripting (XSS) attacks involves implementing several layers of security measures. Here are some key strategies to safeguard your application:
-
Input Validation: Validate all user inputs to ensure they conform to expected formats. Use Yii's built-in validation rules or custom rules to filter out malicious data. For example, you can use
safe
andfilter
validators to sanitize inputs. -
Output Encoding: Always encode output data that is sent to the browser. Yii provides helpers like
Html::encode()
to escape special characters, preventing them from being interpreted as HTML or JavaScript. - Use of CSRF Protection: Yii automatically includes CSRF (Cross-Site Request Forgery) protection in forms. Ensure that this feature is enabled and correctly implemented in your application.
- Content Security Policy (CSP): Implement a Content Security Policy to reduce the risk of XSS attacks. You can set CSP headers using Yii's response object to define which sources of content are allowed.
- Regular Security Updates: Keep your Yii framework and all related libraries up to date to benefit from the latest security patches and enhancements.
-
Security Headers: Utilize security headers like
X-Content-Type-Options
,X-Frame-Options
, andX-XSS-Protection
to enhance browser security settings.
By combining these practices, you can significantly reduce the vulnerability of your Yii application to XSS attacks.
What are the best practices for input validation in Yii to prevent XSS vulnerabilities?
Implementing robust input validation in Yii is critical to preventing XSS vulnerabilities. Here are some best practices:
-
Use Yii's Validation Rules: Leverage Yii's built-in validation rules in your models to enforce data integrity. Common rules include
required
,string
,number
,email
, andurl
. For example:public function rules() { return [ [['username'], 'required'], [['username'], 'string', 'max' => 255], [['email'], 'email'], ]; }
- Custom Validation: For more complex validations, use custom validator functions. You can create custom rules to check for specific conditions or patterns in the input data.
-
Sanitization: Use filters to sanitize user input. Yii provides the
filter
validator, which can be used to apply various filters liketrim
,strip_tags
, or custom filters. - Whitelist Approach: Adopt a whitelist approach to validate inputs. Only allow inputs that meet your predefined criteria and reject all others.
- Validate All Inputs: Ensure every piece of user input is validated, including form data, URL parameters, and cookies.
-
Regular Expressions: Utilize regular expressions for more granular control over input validation. For example, to validate a username:
public function rules() { return [ [['username'], 'match', 'pattern' => '/^[a-zA-Z0-9_] $/'], ]; }
By adhering to these practices, you can effectively validate input in Yii and reduce the risk of XSS vulnerabilities.
How can I implement output encoding in Yii to safeguard against XSS attacks?
Implementing output encoding in Yii is crucial for safeguarding against XSS attacks. Here's how you can do it:
-
Using Html::encode(): Use the
Html::encode()
method to encode any output that is rendered as HTML. This method converts special characters to their HTML entities, preventing the browser from interpreting them as code.echo Html::encode($userInput);
-
HtmlPurifier Extension: For more robust HTML output sanitization, you can use the HtmlPurifier extension. This extension can remove malicious HTML while keeping the content safe.
use yii\htmlpurifier\HtmlPurifier; $purifier = new HtmlPurifier(); echo $purifier->process($userInput);
-
Json Encoding: When outputting JSON data, use
Json::encode()
with theJSON_HEX_TAG
andJSON_HEX_AMP
options to prevent XSS in JSON responses.use yii\helpers\Json; echo Json::encode($data, JSON_HEX_TAG | JSON_HEX_AMP);
-
Attribute Encoding: For HTML attributes, use
Html::encode()
or specific attribute encoders likeHtml::attributeEncode()
to ensure safe attribute values.echo '<input type="text" value="' . Html::encode($userInput) . '">';
-
CSP Headers: In addition to encoding, implementing Content Security Policy headers can further protect against XSS by restricting the sources of executable scripts.
Yii::$app->response->headers->add('Content-Security-Policy', "default-src 'self'; script-src 'self' 'unsafe-inline';");
By consistently applying these output encoding techniques, you can significantly enhance the security of your Yii application against XSS attacks.
Are there any Yii extensions that can help enhance security against XSS?
Yes, several Yii extensions can help enhance security against XSS attacks. Here are some notable ones:
-
yii2-htmlpurifier: This extension integrates HTML Purifier into your Yii application. HTML Purifier is a powerful library that can sanitize HTML input to remove malicious code while preserving safe content.
composer require --prefer-dist yiidoc/yii2-htmlpurifier
-
yii2-esecurity: This extension provides additional security features, including XSS filtering, CSRF protection, and more advanced security headers.
composer require --prefer-dist mihaildev/yii2-elasticsearch
-
yii2-csrf: This extension enhances Yii's built-in CSRF protection, making it more robust and configurable.
composer require --prefer-dist 2amigos/yii2-csrf
-
yii2-csp: This extension helps implement and manage Content Security Policy headers in your Yii application, which can further protect against XSS by restricting script sources.
composer require --prefer-dist linslin/yii2-csp
-
yii2-secure-headers: This extension adds security headers to your application, including those that can mitigate XSS attacks, like
X-XSS-Protection
andContent-Security-Policy
.composer require --prefer-dist wbraganca/yii2-secure-headers
By integrating these extensions into your Yii application, you can bolster its defenses against XSS attacks and enhance overall security.
The above is the detailed content of How can I protect my Yii application against cross-site scripting (XSS) attacks?. For more information, please follow other related articles on the PHP Chinese website!
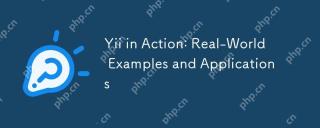
The Yii framework is suitable for developing web applications of all sizes, and its advantages lie in its high performance and rich feature set. 1) Yii adopts an MVC architecture, and its core components include ActiveRecord, Widget and Gii tools. 2) Through the request processing process, Yii efficiently handles HTTP requests. 3) Basic usage shows a simple example of creating controllers and views. 4) Advanced usage demonstrates the flexibility of database operations through ActiveRecord. 5) Debugging skills include using the debug toolbar and logging system. 6) Performance optimization It is recommended to use cache and database query optimization, follow coding specifications and dependency injection to improve code quality.
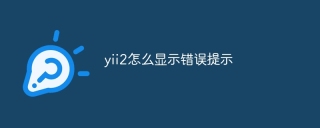
In Yii2, there are two main ways to display error prompts. One is to use Yii::$app->errorHandler->exception() to automatically catch and display errors when an exception occurs. The other is to use $this->addError(), which displays an error when model validation fails and can be accessed in the view through $model->getErrors(). In the view, you can use if ($errors = $model->getErrors())
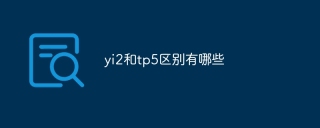
With the continuous development of PHP framework technology, Yi2 and TP5 have attracted much attention as the two mainstream frameworks. They are all known for their outstanding performance, rich functionality and robustness, but they have some differences and advantages and disadvantages. Understanding these differences is crucial for developers to choose frameworks.
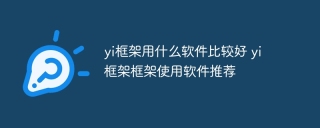
Abstract of the first paragraph of the article: When choosing software to develop Yi framework applications, multiple factors need to be considered. While native mobile application development tools such as XCode and Android Studio can provide strong control and flexibility, cross-platform frameworks such as React Native and Flutter are becoming increasingly popular with the benefits of being able to deploy to multiple platforms at once. For developers new to mobile development, low-code or no-code platforms such as AppSheet and Glide can quickly and easily build applications. Additionally, cloud service providers such as AWS Amplify and Firebase provide comprehensive tools
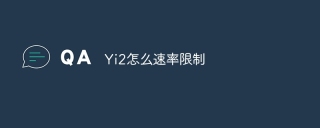
The Yi2 Rate Limiting Guide provides users with a comprehensive guide to how to control the data transfer rate in Yi2 applications. By implementing rate limits, users can optimize application performance, prevent excessive bandwidth consumption and ensure stable and reliable connections. This guide will introduce step-by-step how to configure the rate limit settings of Yi2, covering a variety of platforms and scenarios to meet the different needs of users.
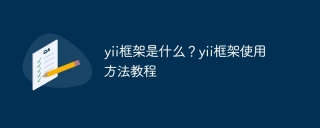
Article Summary: Yii Framework is an efficient and flexible PHP framework for creating dynamic and scalable web applications. It is known for its high performance, lightweight and easy to use features. This article will provide a comprehensive tutorial on the Yii framework, covering everything from installation to configuration to development of applications. This guide is designed to help beginners and experienced developers take advantage of the power of Yii to build reliable and maintainable web solutions.
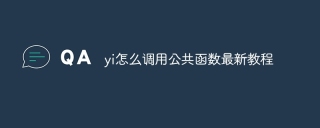
This article introduces the latest tutorial on calling public functions, which is implemented in Easy Language (Yi) language. For beginners, easy-to-language programming languages are easy to learn, and this article provides a detailed step-by-step guide to help users master how to call public functions in Yi applications. By following this tutorial, users will learn how to define, load, and call common functions, thereby enhancing their code reusability and flexibility.
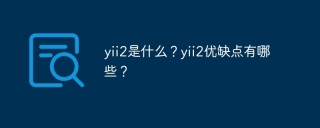
Yii2 is a powerful PHP framework that has been widely praised by developers. With its high performance, scalability and user-friendly interface, it becomes ideal for building large, complex web applications. However, like any framework, Yii2 has some advantages and disadvantages to consider.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
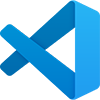
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
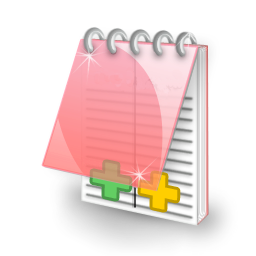
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
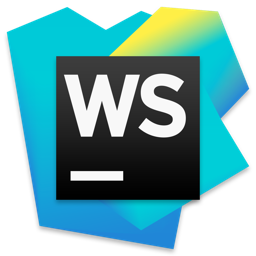
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.