


How do I integrate MongoDB with different programming languages (Python, Java, Node.js)?
Integrating MongoDB with Different Programming Languages (Python, Java, Node.js)
MongoDB offers official drivers for a wide variety of programming languages, making integration relatively straightforward. Here's a breakdown for Python, Java, and Node.js:
Python: The official MongoDB driver for Python is pymongo
. It provides a robust and easy-to-use API for interacting with MongoDB. Installation is typically done via pip: pip install pymongo
. Connecting to a MongoDB instance and performing basic operations (like inserting, querying, and updating documents) involves instantiating a MongoClient
object, specifying the connection string (including hostname, port, and potentially authentication details), accessing a database, and then a collection within that database. For example:
import pymongo client = pymongo.MongoClient("mongodb://localhost:27017/") # Replace with your connection string db = client["mydatabase"] # Replace with your database name collection = db["mycollection"] # Replace with your collection name # Insert a document document = {"name": "John Doe", "age": 30} result = collection.insert_one(document) print(f"Inserted document with ID: {result.inserted_id}") # Query documents query = {"age": {"$gt": 25}} cursor = collection.find(query) for document in cursor: print(document)
Java: The MongoDB Java driver, available through Maven or Gradle, offers similar functionality. You'll need to include the necessary dependencies in your pom.xml
(Maven) or build.gradle
(Gradle) file. The core process involves creating a MongoClient
, accessing a database and collection, and then using methods to perform CRUD (Create, Read, Update, Delete) operations. Example using a simplified approach (error handling omitted for brevity):
import com.mongodb.MongoClient; import com.mongodb.client.MongoCollection; import com.mongodb.client.MongoDatabase; import org.bson.Document; MongoClient mongoClient = new MongoClient("localhost", 27017); // Replace with your connection string MongoDatabase database = mongoClient.getDatabase("mydatabase"); // Replace with your database name MongoCollection<Document> collection = database.getCollection("mycollection"); // Replace with your collection name Document doc = new Document("name", "Jane Doe").append("age", 28); collection.insertOne(doc); // ... further operations ... mongoClient.close();
Node.js: The official Node.js driver, mongodb
, provides a highly asynchronous API leveraging Node.js's event loop. Installation is via npm: npm install mongodb
. Similar to Python and Java, you'll connect to the database, access collections, and perform operations. Example (error handling simplified):
const { MongoClient } = require('mongodb'); const uri = "mongodb://localhost:27017/"; // Replace with your connection string const client = new MongoClient(uri); async function run() { try { await client.connect(); const database = client.db('mydatabase'); // Replace with your database name const collection = database.collection('mycollection'); // Replace with your collection name const doc = { name: "Peter Pan", age: 35 }; const result = await collection.insertOne(doc); console.log(`Inserted document with ID: ${result.insertedId}`); } finally { await client.close(); } } run().catch(console.dir);
Best Practices for Securing a MongoDB Database Integrated with Various Programming Languages
Securing your MongoDB database is crucial, regardless of the programming language used. Here are some key best practices:
-
Authentication: Always enable authentication. Use strong passwords and avoid default credentials. MongoDB supports various authentication mechanisms like SCRAM-SHA-1 and X.509 certificates. Configure authentication in your
mongod.conf
file and ensure your drivers are configured to use the appropriate credentials. - Authorization: Implement role-based access control (RBAC) to grant users only the necessary permissions. Avoid granting excessive privileges. Define roles with specific permissions for read, write, and other database operations.
- Network Security: Restrict network access to your MongoDB instance. Use firewalls to limit access only to authorized IP addresses or networks. Avoid exposing your database to the public internet.
- Connection String Security: Never hardcode connection strings directly into your application code. Instead, store them securely using environment variables or a secrets management system.
- Input Validation: Sanitize and validate all user inputs before they are used in database queries. This helps prevent injection attacks like NoSQL injection.
- Regular Updates and Patching: Keep your MongoDB instance and drivers updated with the latest security patches to address known vulnerabilities.
- Data Encryption: Encrypt sensitive data at rest and in transit using TLS/SSL encryption. Consider using encryption at the application level as well.
- Monitoring and Auditing: Regularly monitor your database for suspicious activity and implement auditing to track user actions and identify potential security breaches.
Which Programming Language is Most Efficient for Connecting to and Querying a MongoDB Database?
The efficiency of connecting to and querying a MongoDB database depends less on the programming language itself and more on factors like:
- Driver Optimization: The efficiency of the MongoDB driver for a specific language plays a significant role. Generally, the official drivers are well-optimized.
- Query Optimization: The efficiency of your queries is paramount. Using appropriate indexes, employing efficient query patterns, and avoiding unnecessary data retrieval are crucial for performance.
- Network Latency: Network conditions and the distance between your application and the database server significantly impact performance.
- Application Design: The overall architecture of your application and how it interacts with the database will affect performance.
While there might be subtle differences in performance between drivers for different languages, they are often negligible in practice. The choice of programming language should primarily be driven by other factors like developer expertise, project requirements, and existing infrastructure.
Common Challenges Faced When Integrating MongoDB with Different Programming Languages, and How to Overcome Them
Some common challenges include:
- Driver Compatibility: Ensuring compatibility between the MongoDB driver and the specific version of your programming language and its dependencies can be challenging. Always refer to the official documentation for compatibility information and follow best practices for dependency management.
- Error Handling: Proper error handling is crucial. Unhandled exceptions can lead to application crashes or data inconsistencies. Implement robust error handling mechanisms in your code to catch and manage potential errors during database operations.
- Asynchronous Operations (Node.js): Effectively handling asynchronous operations in Node.js requires understanding Promises and async/await. Improper handling can lead to performance issues or race conditions.
- Connection Management: Efficiently managing database connections is essential to avoid resource exhaustion. Use connection pooling techniques to reuse connections and minimize overhead.
- Data Modeling: Designing an efficient data model that suits your application's needs and leverages MongoDB's features (like embedded documents and arrays) is vital for performance and scalability.
- Large Datasets: Handling large datasets efficiently requires optimization strategies like using aggregation pipelines, sharding, and appropriate indexing.
To overcome these challenges:
- Consult Official Documentation: Always refer to the official MongoDB documentation and the documentation for your chosen programming language's driver.
- Use Best Practices: Follow best practices for database design, connection management, error handling, and query optimization.
- Testing and Debugging: Thoroughly test your code and use debugging tools to identify and resolve issues.
- Community Support: Utilize online forums and communities for assistance with specific problems. Many experienced developers are willing to help.
The above is the detailed content of How do I integrate MongoDB with different programming languages (Python, Java, Node.js)?. For more information, please follow other related articles on the PHP Chinese website!
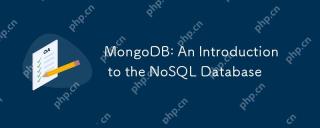
MongoDB is a document-based NoSQL database that uses BSON format to store data, suitable for processing complex and unstructured data. 1) Its document model is flexible and suitable for frequently changing data structures. 2) MongoDB uses WiredTiger storage engine and query optimizer to support efficient data operations and queries. 3) Basic operations include inserting, querying, updating and deleting documents. 4) Advanced usage includes using an aggregation framework for complex data analysis. 5) Common errors include connection problems, query performance problems, and data consistency problems. 6) Performance optimization and best practices include index optimization, data modeling, sharding, caching, monitoring and tuning.
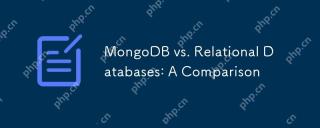
MongoDB is suitable for scenarios that require flexible data models and high scalability, while relational databases are more suitable for applications that complex queries and transaction processing. 1) MongoDB's document model adapts to the rapid iterative modern application development. 2) Relational databases support complex queries and financial systems through table structure and SQL. 3) MongoDB achieves horizontal scaling through sharding, which is suitable for large-scale data processing. 4) Relational databases rely on vertical expansion and are suitable for scenarios where queries and indexes need to be optimized.
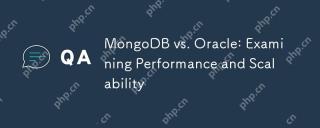
MongoDB performs excellent in performance and scalability, suitable for high scalability and flexibility requirements; Oracle performs excellent in requiring strict transaction control and complex queries. 1.MongoDB achieves high scalability through sharding technology, suitable for large-scale data and high concurrency scenarios. 2. Oracle relies on optimizers and parallel processing to improve performance, suitable for structured data and transaction control needs.
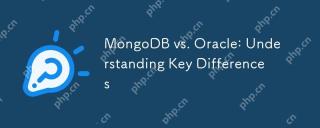
MongoDB is suitable for handling large-scale unstructured data, and Oracle is suitable for enterprise-level applications that require transaction consistency. 1.MongoDB provides flexibility and high performance, suitable for processing user behavior data. 2. Oracle is known for its stability and powerful functions and is suitable for financial systems. 3.MongoDB uses document models, and Oracle uses relational models. 4.MongoDB is suitable for social media applications, while Oracle is suitable for enterprise-level applications.
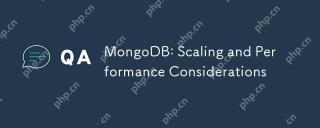
MongoDB's scalability and performance considerations include horizontal scaling, vertical scaling, and performance optimization. 1. Horizontal expansion is achieved through sharding technology to improve system capacity. 2. Vertical expansion improves performance by increasing hardware resources. 3. Performance optimization is achieved through rational design of indexes and optimized query strategies.
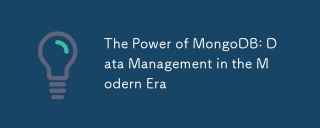
MongoDB is a NoSQL database because of its flexibility and scalability are very important in modern data management. It uses document storage, is suitable for processing large-scale, variable data, and provides powerful query and indexing capabilities.
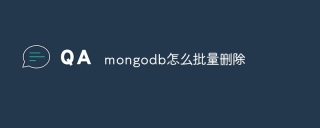
You can use the following methods to delete documents in MongoDB: 1. The $in operator specifies the list of documents to be deleted; 2. The regular expression matches documents that meet the criteria; 3. The $exists operator deletes documents with the specified fields; 4. The find() and remove() methods first get and then delete the document. Please note that these operations cannot use transactions and may delete all matching documents, so be careful when using them.

To set up a MongoDB database, you can use the command line (use and db.createCollection()) or the mongo shell (mongo, use and db.createCollection()). Other setting options include viewing database (show dbs), viewing collections (show collections), deleting database (db.dropDatabase()), deleting collections (db.<collection_name>.drop()), inserting documents (db.<collecti


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
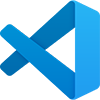
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
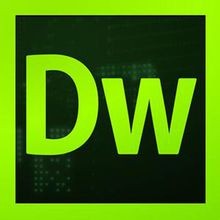
Dreamweaver CS6
Visual web development tools