


How do I use mocking frameworks like Mockito or EasyMock in Java unit tests?
How to Use Mocking Frameworks Like Mockito or EasyMock in Java Unit Tests
Mocking frameworks like Mockito and EasyMock allow you to isolate the unit under test from its dependencies during unit testing. This isolation ensures that your tests focus solely on the functionality of the unit itself, preventing external factors from influencing the test results. Let's look at how to use Mockito, a popular choice, as an example.
First, you need to add the Mockito dependency to your project's pom.xml
(for Maven) or build.gradle
(for Gradle). Then, within your test class, you create mock objects using the Mockito.mock()
method. These mock objects simulate the behavior of the real dependencies.
import org.mockito.Mockito; import org.junit.jupiter.api.Test; import static org.junit.jupiter.api.Assertions.*; // ... your classes ... public class MyServiceTest { @Test void testMyMethod() { // Create a mock object of the dependency DependencyInterface dependency = Mockito.mock(DependencyInterface.class); // Set up the behavior of the mock object Mockito.when(dependency.someMethod("input")).thenReturn("expectedOutput"); // Create an instance of the class under test, injecting the mock object MyService service = new MyService(dependency); // Call the method under test String result = service.myMethod("input"); // Assert the expected result assertEquals("expectedOutput", result); } }
In this example, DependencyInterface
is a dependency of MyService
. We create a mock of DependencyInterface
and define its behavior using Mockito.when()
. Mockito.when(dependency.someMethod("input")).thenReturn("expectedOutput")
specifies that when someMethod
is called with "input", it should return "expectedOutput". Finally, we assert that the myMethod
of MyService
returns the expected value. EasyMock follows a similar pattern, though its syntax differs slightly.
Best Practices for Writing Effective Unit Tests Using Mocking Frameworks in Java
Writing effective unit tests with mocking frameworks requires careful consideration of several best practices:
- Test One Thing at a Time: Each test should focus on a single unit of functionality. Avoid testing multiple aspects within a single test. This improves readability and maintainability.
-
Keep Tests Concise and Readable: Tests should be short, easy to understand, and focused. Clear naming conventions (e.g.,
testMethodName_GivenCondition_WhenAction_ThenResult
) help in readability. - Use Descriptive Test Names: The test name should clearly communicate what is being tested and the expected outcome.
- Verify Only Necessary Interactions: Only verify the interactions with mocks that are crucial to the functionality being tested. Over-verification can make tests brittle and harder to maintain.
- Avoid Over-Mocking: While mocking is essential, avoid mocking everything. Mock only the necessary dependencies to isolate the unit under test effectively. Excessive mocking can lead to tests that are not representative of the real-world behavior.
- Use Test Doubles Appropriately: Utilize different types of test doubles (mocks, stubs, spies) strategically. Choose the appropriate type based on the specific needs of the test.
How to Effectively Handle Complex Dependencies When Unit Testing with Mockito or EasyMock
When dealing with complex dependencies, consider these strategies:
- Dependency Injection: Use dependency injection to easily replace real dependencies with mock objects. This allows for cleaner separation of concerns and easier testing.
- Layer Your Dependencies: Break down complex dependencies into smaller, more manageable units. This makes mocking individual components simpler.
-
Partial Mocking: Use
Mockito.spy()
to create a spy object. This allows you to mock specific methods of a real object while leaving others untouched. This is useful when you want to test interactions with a partially mocked dependency. - Abstracting Dependencies: Define interfaces for your dependencies. This allows you to easily switch between real and mock implementations during testing.
Common Pitfalls to Avoid When Using Mocking Frameworks for Java Unit Tests
Several common pitfalls can hinder the effectiveness of your unit tests:
- Incorrect Mocking: Ensure that you correctly set up the expected behavior of your mock objects. Failing to do so can lead to false positives or negatives in your tests.
- Unnecessary Mocking: Avoid mocking components that are not essential for the test. This can lead to overly complex and fragile tests.
-
Ignoring Exceptions: Don't forget to verify that exceptions are thrown when expected. Use
Mockito.doThrow()
to simulate exceptions thrown by mocked dependencies. - Tight Coupling: Avoid tight coupling between your unit under test and its dependencies. This makes testing significantly more difficult.
- Over-Verification: Avoid verifying every single interaction with a mock object. Focus on verifying only the crucial interactions relevant to the test case. Excessive verification can make tests brittle and harder to maintain.
-
Not Using
@InjectMocks
(Mockito): For simpler cases, using@InjectMocks
annotation can reduce boilerplate code for dependency injection. Remember to use@Mock
for your dependencies to let Mockito inject the mock objects.
By following these best practices and avoiding these common pitfalls, you can effectively leverage mocking frameworks like Mockito and EasyMock to write robust and reliable unit tests for your Java applications.
The above is the detailed content of How do I use mocking frameworks like Mockito or EasyMock in Java unit tests?. For more information, please follow other related articles on the PHP Chinese website!
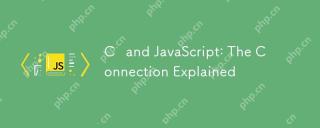
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.
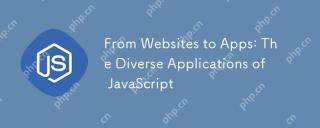
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
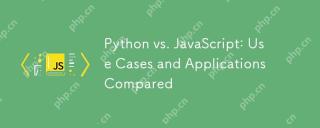
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
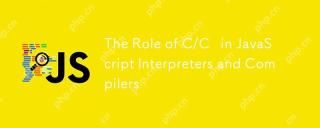
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
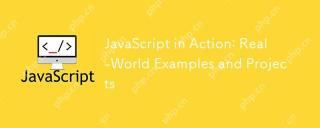
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
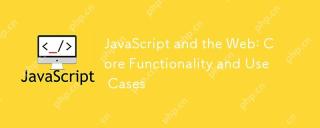
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
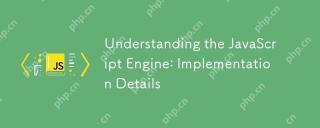
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 English version
Recommended: Win version, supports code prompts!
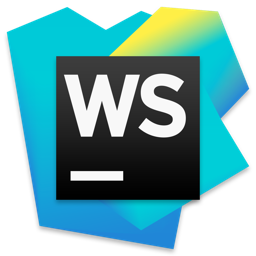
WebStorm Mac version
Useful JavaScript development tools
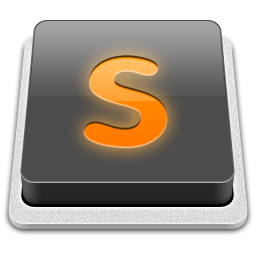
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version