


How do I write unit tests and integration tests in Java using JUnit or TestNG?
How to Write Unit and Integration Tests in Java Using JUnit or TestNG
This section details how to write unit and integration tests in Java using JUnit 5 (for brevity, we'll focus on JUnit 5; TestNG principles are similar). We'll illustrate with examples.
Unit Tests: Unit tests focus on individual units of code, typically a single class or method. They should be isolated from external dependencies like databases or network calls. Mocking frameworks like Mockito are invaluable for this.
import org.junit.jupiter.api.Test; import static org.junit.jupiter.api.Assertions.*; import org.mockito.Mockito; class Calculator { int add(int a, int b) { return a b; } } class CalculatorTest { @Test void testAdd() { Calculator calculator = new Calculator(); assertEquals(5, calculator.add(2, 3)); } }
Integration Tests: Integration tests verify the interaction between different components or modules of your application. They often involve real databases, external services, or other dependencies.
import org.junit.jupiter.api.Test; import static org.junit.jupiter.api.Assertions.*; //Import necessary classes for database interaction (e.g., JDBC) class UserDAO { //Data Access Object interacting with a database //Methods to interact with the database (CRUD operations) } class UserDAOIntegrationTest { @Test void testCreateUser() { UserDAO dao = new UserDAO();//Initialize with database connection // Perform database operation and assert results assertTrue(dao.createUser("testuser", "password")); //Example assertion } }
Remember to include the necessary JUnit 5 dependency in your pom.xml
(if using Maven):
<dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter-api</artifactId> <version>5.11.0-M1</version> <scope>test</scope> </dependency> ``` Replace `5.11.0-M1` with the latest version. For Mockito:
<code><groupid>org.mockito</groupid> <artifactid>mockito-core</artifactid> <version>5.0.0</version> <scope>test</scope></code>
<code> ## Key Differences Between Unit and Integration Tests The core distinction lies in their scope and dependencies: * **Unit Tests:** Test individual units in isolation. They use mocks or stubs to simulate dependencies, ensuring that the test focuses solely on the unit's logic. They are faster to execute and easier to debug. * **Integration Tests:** Test the interaction between multiple units or modules. They use real dependencies, reflecting a closer approximation to the production environment. They are slower to run and more complex to debug due to the involvement of multiple components. Failures can be harder to pinpoint to a specific unit. ## Effectively Structuring Your Java Test Suite A well-structured test suite enhances maintainability and readability. Consider these points: * **Package Structure:** Mirror your production code's package structure for your test code. This makes it easy to locate tests corresponding to specific components. For example, if you have a `com.example.service` package, create a `com.example.service.test` package for its tests. * **Naming Conventions:** Use clear and descriptive names for your test classes and methods. A common convention is to append "Test" to the class name being tested (e.g., `UserServiceTest`). Method names should clearly indicate the tested functionality (e.g., `testCreateUser`, `testUpdateUser`). * **Test Categories (JUnit 5):** Use tags or categories to group tests based on functionality or type (unit vs. integration). This allows for selective test execution. * **Test Suites (JUnit 5 or TestNG):** Combine related tests into suites for easier management and execution. ## Best Practices for Writing High-Quality, Reliable Unit and Integration Tests * **Keep Tests Small and Focused:** Each test should verify a single aspect of the functionality. Avoid large, complex tests that test multiple things at once. * **Use Assertions Effectively:** Clearly state your expected outcomes using assertions. JUnit provides various assertion methods (`assertEquals`, `assertTrue`, `assertNull`, etc.). * **Write Tests First (Test-Driven Development - TDD):** Write your tests *before* implementing the code. This ensures that your code is testable and drives design decisions. * **Test Edge Cases and Boundary Conditions:** Don't just test the happy path. Consider edge cases (e.g., null inputs, empty strings, maximum values) and boundary conditions to ensure robustness. * **Use a Mocking Framework (for Unit Tests):** Mockito is a popular choice. Mocking simplifies testing by isolating units from external dependencies. * **Use a Test Runner (JUnit 5 or TestNG):** These frameworks provide the infrastructure for running your tests and generating reports. * **Automate Test Execution:** Integrate your tests into your CI/CD pipeline for continuous testing. * **Maintain Test Coverage:** Strive for high but realistic test coverage. 100% coverage isn't always necessary or practical, but aim for comprehensive coverage of critical paths and functionality. Tools can help measure test coverage. By following these guidelines, you can create a robust and maintainable test suite for your Java projects, improving code quality and reducing the risk of bugs.</code>
The above is the detailed content of How do I write unit tests and integration tests in Java using JUnit or TestNG?. For more information, please follow other related articles on the PHP Chinese website!
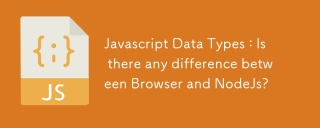
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
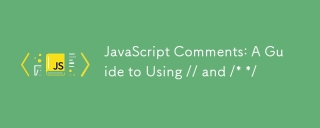
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
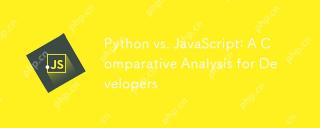
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
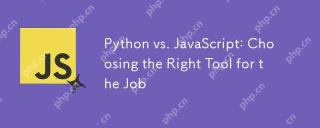
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
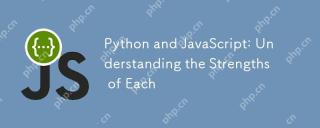
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
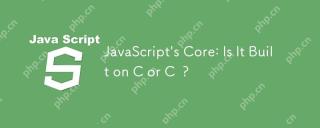
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
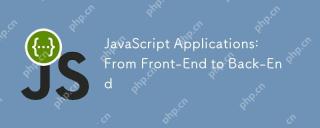
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
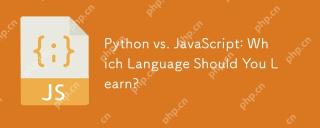
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
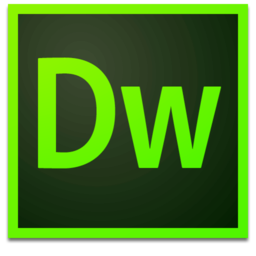
Dreamweaver Mac version
Visual web development tools
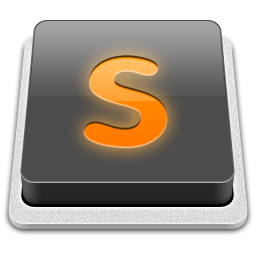
SublimeText3 Mac version
God-level code editing software (SublimeText3)
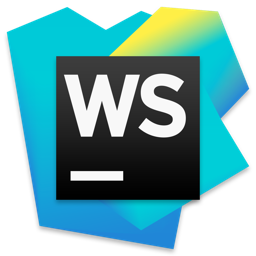
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
