


How do I use dependency injection (DI) in Java with frameworks like Spring or Guice?
How to Use Dependency Injection (DI) in Java with Frameworks like Spring or Guice?
Implementing Dependency Injection with Spring:
Spring is a widely-used framework that simplifies DI implementation in Java. It primarily uses XML configuration, annotations, or Java-based configuration to manage dependencies.
- XML Configuration: This traditional approach involves defining beans and their dependencies in an XML file. Spring's container then reads this file and creates and manages the objects. While powerful, this method can become cumbersome for larger projects.
-
Annotations: This more modern approach utilizes annotations like
@Component
,@Autowired
, and@Inject
to declare beans and their dependencies directly within the Java code. This makes the configuration more concise and maintainable.@Component
marks a class as a Spring-managed bean.@Autowired
automatically injects dependencies by type.@Inject
(requires adding JSR-330 dependency) offers a similar functionality. -
Java-based Configuration: This approach uses Java classes annotated with
@Configuration
to define beans and their dependencies programmatically. This provides a cleaner and more flexible alternative to XML configuration. It leverages methods annotated with@Bean
to create and configure beans.
Implementing Dependency Injection with Guice:
Guice, a lightweight DI framework, uses a different approach. It relies heavily on annotations and a programmatic binding process.
-
Annotations: Guice uses annotations like
@Inject
to indicate dependencies. It also offers@Provides
to define methods that create and configure objects. -
Binding: The core of Guice is its injector, which is responsible for creating and managing objects. You bind interfaces to their implementations using the
bind()
method in a module. This allows for more fine-grained control over the dependency injection process.
Example (Spring with Annotations):
// Service Interface public interface UserService { void greetUser(String name); } // Service Implementation @Component public class UserServiceImpl implements UserService { @Override public void greetUser(String name) { System.out.println("Hello, " name "!"); } } // Client Class @Component public class Client { @Autowired private UserService userService; public void useService(String name) { userService.greetUser(name); } }
What are the Best Practices for Implementing Dependency Injection in a Java Application?
- Favor Interface over Implementation: Inject interfaces rather than concrete classes. This promotes loose coupling and allows for easier swapping of implementations.
- Keep Dependencies Explicit: Clearly define all dependencies. Avoid implicit dependencies or relying on static methods.
- Use Constructor Injection: Prefer constructor injection for mandatory dependencies. This ensures that objects are properly initialized with all necessary dependencies.
- Use Setter Injection for Optional Dependencies: Use setter injection for optional dependencies. This allows for more flexibility and easier testing.
- Avoid Circular Dependencies: Circular dependencies (where A depends on B, and B depends on A) can lead to errors. Carefully design your architecture to avoid such situations.
- Use a DI Framework: Employ a DI framework like Spring or Guice to manage the complexities of dependency injection, especially in larger applications.
- Keep Modules Small and Focused: Organize your code into smaller, well-defined modules with clear responsibilities. This improves maintainability and testability.
How Does Dependency Injection Improve Code Maintainability and Testability in Java Projects?
Dependency injection significantly enhances maintainability and testability in several ways:
- Loose Coupling: DI promotes loose coupling between components. Changes in one part of the application are less likely to affect other parts. This makes the code easier to maintain and refactor.
- Improved Testability: DI makes unit testing much easier. You can easily mock or stub dependencies during testing, isolating the unit under test and ensuring reliable test results. This reduces the reliance on complex test setups and makes testing more efficient.
- Reusability: Components become more reusable because they are independent of their dependencies. They can be easily integrated into different parts of the application or even into other applications.
- Simplified Debugging: The explicit nature of dependencies makes debugging easier. Tracing the flow of data and identifying the source of errors becomes simpler.
What are the Key Differences Between Spring and Guice in Terms of Dependency Injection Mechanisms?
Spring and Guice, while both implementing DI, differ in their approaches:
- Configuration: Spring offers various configuration mechanisms (XML, annotations, JavaConfig), providing flexibility but potentially increasing complexity. Guice primarily uses annotations and programmatic binding, offering a more concise and arguably simpler configuration process.
- XML vs. Code: Spring historically relied heavily on XML configuration, while Guice prioritizes code-based configuration. While Spring has moved towards annotation-based configuration, the XML option remains.
-
Control: Guice provides more fine-grained control over the dependency injection process through its programmatic binding mechanism. Spring's automatic dependency resolution (using
@Autowired
) is convenient but offers less control. - Size and Complexity: Guice is generally considered more lightweight and less complex than Spring, which is a much larger framework offering many features beyond DI (e.g., AOP, transaction management, web framework).
- Learning Curve: Spring, due to its extensive features, might have a steeper learning curve compared to Guice, which is often perceived as simpler to learn and use.
In essence, the choice between Spring and Guice depends on the project's size, complexity, and specific needs. Spring is a powerful, all-in-one framework suitable for large-scale applications, while Guice is a lightweight alternative ideal for smaller projects where a more concise and programmatic approach is preferred.
The above is the detailed content of How do I use dependency injection (DI) in Java with frameworks like Spring or Guice?. For more information, please follow other related articles on the PHP Chinese website!
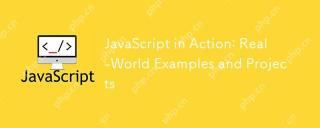
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
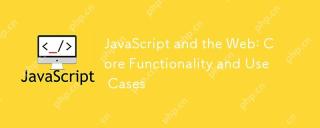
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
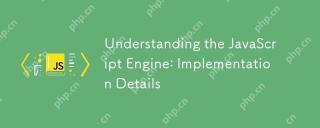
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
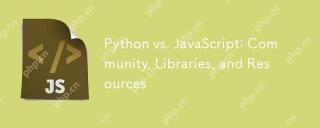
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
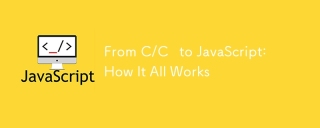
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
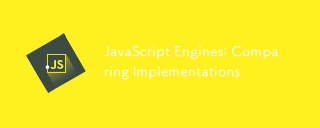
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
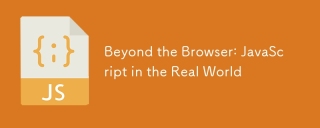
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
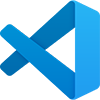
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
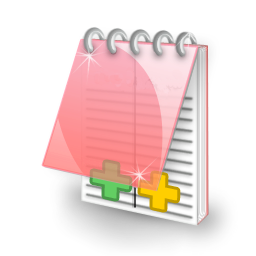
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
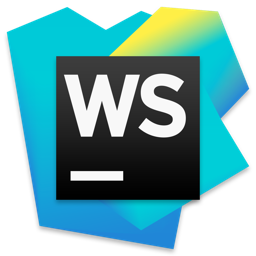
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.