


How to Implement Caching in Java Applications for Improved Performance?
Implementing caching in Java applications involves strategically storing frequently accessed data in a readily available location, like memory, to reduce the latency of retrieving that data. This significantly boosts performance by avoiding expensive database or network calls. Here's a breakdown of the process:
1. Identify Cacheable Data: The first step is to pinpoint data that benefits most from caching. This typically includes frequently accessed, read-heavy data that doesn't change often. Examples include user profiles, product catalogs, or configuration settings. Avoid caching data that changes frequently or is volatile, as this can lead to stale data and inconsistencies.
2. Choose a Caching Strategy: Select an appropriate caching strategy based on your application's needs. Common strategies include:
- Write-through caching: Data is written to both the cache and the underlying data store simultaneously. This ensures data consistency but can be slower.
- Write-back caching (or write-behind caching): Data is written to the cache first, and asynchronously written to the underlying data store later. This is faster but risks data loss if the cache fails before the data is persisted.
- Read-through caching: Data is first checked in the cache; if not found, it's fetched from the underlying data store, added to the cache, and then returned. This is a common and efficient approach.
- Cache eviction policies: When the cache reaches its capacity, you need an eviction policy to remove less frequently used data. Common policies include Least Recently Used (LRU), Least Frequently Used (LFU), and First In, First Out (FIFO).
3. Select a Caching Library: Leverage a robust Java caching library like Caffeine, Ehcache, or Guava's CacheBuilder. These libraries handle complex aspects like eviction policies, concurrency, and serialization efficiently.
4. Implement the Cache: Use the chosen library to create a cache instance, configure its parameters (e.g., maximum size, eviction policy), and integrate it into your application's data access layer. Wrap your database or external service calls with cache checks to retrieve data from the cache first, falling back to the original data source only if a cache miss occurs.
5. Monitor and Tune: Regularly monitor cache hit rates and eviction statistics to fine-tune your caching strategy. Adjust parameters like cache size and eviction policy to optimize performance based on your application's usage patterns.
What Caching Strategies are Best Suited for Different Types of Java Applications?
The optimal caching strategy depends heavily on the application's characteristics:
- High-traffic web applications: Read-through caching with a write-back strategy for updates is generally a good fit. This balances speed and data consistency. LRU or LFU eviction policies are commonly used.
- Real-time applications: Write-through caching might be preferred to ensure data consistency, even at the cost of slightly reduced speed.
- Batch processing applications: Write-back caching could be efficient, as the asynchronous writes to the persistent store can be performed during periods of low activity.
- Applications with frequent updates: A strategy that balances consistency and performance is crucial. Consider using a write-through cache with a smaller size to limit the impact of frequent updates, or implement a more sophisticated caching strategy with multiple cache levels (e.g., a fast, smaller L1 cache and a slower, larger L2 cache).
- Applications with limited memory: Careful consideration of cache size and eviction policies is essential. A smaller cache with an aggressive eviction policy might be necessary to prevent OutOfMemoryErrors.
What are the Common Pitfalls to Avoid When Implementing Caching in Java?
Several common pitfalls can undermine the effectiveness of caching:
- Caching mutable objects: Caching mutable objects can lead to inconsistencies and unexpected behavior. Ensure that objects stored in the cache are immutable or properly synchronized.
- Ignoring cache invalidation: Failing to invalidate cached data when the underlying data changes results in stale data. Implement proper cache invalidation mechanisms, such as time-to-live (TTL) settings or explicit invalidation methods.
- Ignoring cache eviction policies: Improperly configured or chosen eviction policies can lead to cache thrashing (constant eviction and reloading of data).
- Ignoring cache concurrency: Not handling concurrent access to the cache correctly can lead to data corruption or performance degradation. Use thread-safe caching libraries or implement proper synchronization mechanisms.
- Over-reliance on caching: Caching should be used strategically. Don't cache everything; only cache data that significantly benefits from caching.
- Insufficient monitoring: Without monitoring cache hit rates and other metrics, it's impossible to assess the effectiveness of the caching strategy.
Which Java Caching Libraries or Frameworks are Most Efficient and Easy to Integrate?
Several excellent Java caching libraries offer efficiency and ease of integration:
- Caffeine: A high-performance, near-drop-in replacement for Guava's Cache, known for its speed and minimal dependencies. It's excellent for smaller-scale applications or situations requiring high performance.
- Ehcache: A mature and feature-rich library suitable for larger-scale applications. It offers advanced features like distributed caching, persistence, and various eviction policies. It might be slightly more complex to set up initially than Caffeine.
- Hazelcast: A powerful, distributed in-memory data grid that includes caching capabilities. It's ideal for clustered applications requiring distributed caching and data consistency across multiple nodes.
- Guava Cache: Part of the widely used Guava library, it provides a simple and efficient caching implementation. While not as feature-rich as Ehcache, its ease of use makes it a good choice for simpler applications.
The best choice depends on your application's specific requirements. For simpler applications, Caffeine or Guava's Cache might suffice. For larger, more complex applications, or those requiring distributed caching, Ehcache or Hazelcast are better choices. Consider factors like scalability, features, and ease of integration when making your selection.
The above is the detailed content of How do I implement caching in Java applications for improved performance?. For more information, please follow other related articles on the PHP Chinese website!
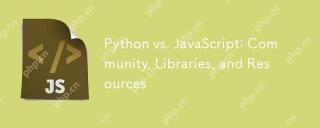
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
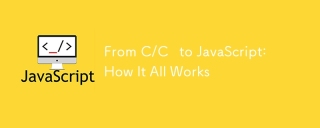
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
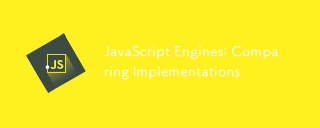
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
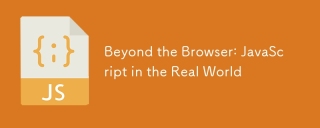
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
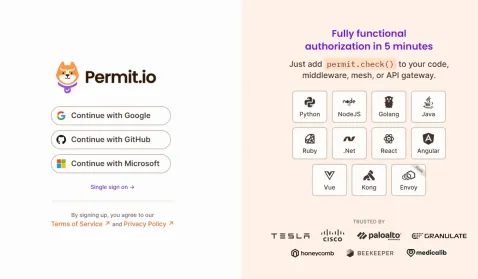
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
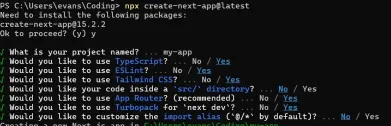
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
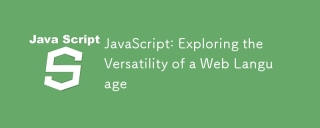
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
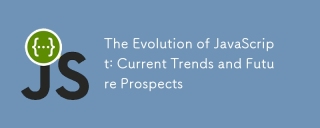
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
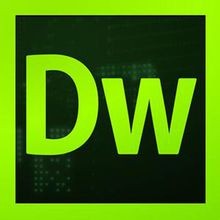
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
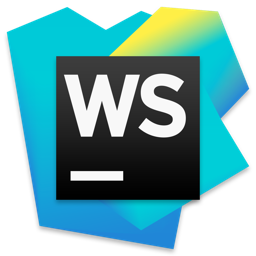
WebStorm Mac version
Useful JavaScript development tools