Implementing Rate Limiting and API Throttling in Laravel Applications
Rate limiting and API throttling are crucial for protecting your Laravel applications from abuse and ensuring the stability and performance of your services. Laravel provides built-in mechanisms to easily implement these security measures. The primary tool is the throttle
middleware. This middleware checks against a cache (typically configured to use Redis or database) to track the number of requests made from a given IP address within a specified time window. If the limit is exceeded, the middleware returns a 429 Too Many Requests HTTP response.
To implement rate limiting, you'll typically add the throttle
middleware to your API routes. For example, in your routes/api.php
file:
Route::middleware('auth:sanctum', 'throttle:60,1')->group(function () { Route::get('/users', [UserController::class, 'index']); Route::post('/users', [UserController::class, 'store']); });
This code snippet limits requests to 60 requests per minute (60 requests, 1 minute). The auth:sanctum
middleware ensures only authenticated users can access these routes, further enhancing security. The throttle
middleware parameters are flexible; you can adjust the number of requests and the time window to suit your application's needs. Remember to configure your caching system appropriately. Redis is highly recommended for performance, especially under high load.
Best Practices for Securing Laravel APIs Using Rate Limiting
While the throttle
middleware is a great starting point, several best practices can further enhance your API's security:
- Granular Control: Don't apply a single rate limit to your entire API. Implement different limits for different endpoints based on their resource intensity and sensitivity. For example, a resource-intensive endpoint might have a lower limit than a less demanding one.
- User-Based Throttling: Instead of just IP-based throttling, consider user-based throttling. This limits requests based on authenticated users, allowing more flexibility and fairer treatment of legitimate users. You can achieve this by adding user-specific identifiers to the throttle key.
- Combining with other security measures: Rate limiting should be part of a layered security strategy. Combine it with input validation, authentication (e.g., using Sanctum, Passport, or other authentication providers), authorization, and output sanitization.
- Monitoring and Alerting: Monitor your rate limiting statistics to identify potential abuse patterns or bottlenecks. Set up alerts to notify you when rate limits are frequently reached, allowing you to proactively address potential issues.
- Regular Review and Adjustment: Regularly review your rate limiting configuration. As your application grows and usage patterns change, you may need to adjust your limits to maintain optimal performance and security.
Customizing Error Responses for Rate-Limited Requests in Laravel
Laravel's default 429 response provides basic information. You can customize this to provide more user-friendly and informative error messages. You can achieve this using exception handling and custom responses.
For example, create a custom exception handler:
<?php namespace App\Exceptions; use Illuminate\Http\JsonResponse; use Illuminate\Validation\ValidationException; use Illuminate\Auth\AuthenticationException; use Illuminate\Foundation\Exceptions\Handler as ExceptionHandler; use Symfony\Component\HttpKernel\Exception\HttpException; use Throwable; use Illuminate\Http\Response; use Symfony\Component\HttpFoundation\Response as SymfonyResponse; class Handler extends ExceptionHandler { public function render($request, Throwable $exception) { if ($exception instanceof HttpException && $exception->getStatusCode() === SymfonyResponse::HTTP_TOO_MANY_REQUESTS) { return response()->json([ 'error' => 'Too Many Requests', 'message' => 'Rate limit exceeded. Please try again later.', 'retry_after' => $exception->getHeaders()['Retry-After'] ?? 60, //Seconds ], SymfonyResponse::HTTP_TOO_MANY_REQUESTS); } return parent::render($request, $exception); } }
This code intercepts the 429 response and returns a custom JSON response with more descriptive information, including a retry_after
field indicating when the user can retry. You can further customize this to include more context-specific information based on the type of rate limiting being used.
Different Rate Limiting Strategies in Laravel and Choosing the Right One
Laravel's throttle
middleware primarily offers IP-address-based rate limiting. However, you can achieve more sophisticated strategies through custom logic and cache key manipulation.
- IP-based: The simplest approach, limiting requests based on the client's IP address. Suitable for general protection against basic attacks but can be bypassed with proxies or shared IP addresses.
- User-based: Limits requests based on authenticated users. This offers a more nuanced approach, allowing more requests from legitimate users while still protecting against abuse. This requires user authentication.
- Endpoint-specific: Different rate limits for different API endpoints. This allows tailoring protection based on the resource intensity and sensitivity of each endpoint.
- Combined strategies: You can combine these strategies. For example, you might have an IP-based limit for unauthenticated requests and a more generous user-based limit for authenticated users. You can achieve this by crafting custom cache keys that incorporate both IP addresses and user IDs.
Choosing the best strategy depends on your application's specific needs and security requirements. For a simple API, IP-based limiting might suffice. For more complex applications with user authentication, a combination of IP-based and user-based limiting offers stronger protection. Always prioritize granular control and regular review to adapt to changing usage patterns and potential threats.
The above is the detailed content of How to Implement Rate Limiting and API Throttling in Laravel Applications?. For more information, please follow other related articles on the PHP Chinese website!

Laravel10,releasedonFebruary7,2023,isthelatestversion.Itfeatures:1)Improvederrorhandlingwithanewreportmethodintheexceptionhandler,2)EnhancedsupportforPHP8.1featureslikeenums,and3)AnewLaravel\Promptspackageforinteractivecommand-lineprompts.

ThelatestLaravelversionenhancesdevelopmentwith:1)Simplifiedroutingusingimplicitmodelbinding,2)EnhancedEloquentcapabilitieswithnewquerymethods,and3)ImprovedsupportformodernPHPfeatureslikenamedarguments,makingcodingmoreefficientandenjoyable.

You can find the release notes for the latest Laravel version at laravel.com/docs. 1) Release Notes provide detailed information on new features, bug fixes and improvements. 2) They contain examples and explanations to help understand the application of new features. 3) Pay attention to the potential complexity and backward compatibility issues of new features. 4) Regular review of release notes can keep it updated and inspire innovation.

Theessentialtoolsforstayingconnectedindistributedteamsinclude:1)CommunicationtoolslikeZoom,MicrosoftTeams,Slack,andDiscordforeffectivecommunication;2)ProjectmanagementtoolssuchasTrello,Asana,andJirafortaskmanagementandworkfloworganization;3)Collabora

Laravel stands out by simplifying the web development process and delivering powerful features. Its advantages include: 1) concise syntax and powerful ORM system, 2) efficient routing and authentication system, 3) rich third-party library support, allowing developers to focus on writing elegant code and improve development efficiency.

Laravelispredominantlyabackendframework,designedforserver-sidelogic,databasemanagement,andAPIdevelopment,thoughitalsosupportsfrontenddevelopmentwithBladetemplates.

Laravel and Python have their own advantages and disadvantages in terms of performance and scalability. Laravel improves performance through asynchronous processing and queueing systems, but due to PHP limitations, there may be bottlenecks when high concurrency is present; Python performs well with the asynchronous framework and a powerful library ecosystem, but is affected by GIL in a multi-threaded environment.

Laravel is suitable for projects that teams are familiar with PHP and require rich features, while Python frameworks depend on project requirements. 1.Laravel provides elegant syntax and rich features, suitable for projects that require rapid development and flexibility. 2. Django is suitable for complex applications because of its "battery inclusion" concept. 3.Flask is suitable for fast prototypes and small projects, providing great flexibility.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Zend Studio 13.0.1
Powerful PHP integrated development environment
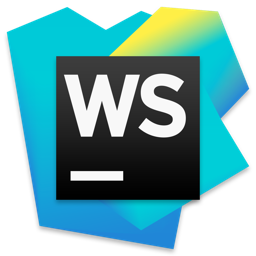
WebStorm Mac version
Useful JavaScript development tools
