Working with Forms and User Input Validation in Yii
Yii provides a robust framework for handling forms and validating user input. The core component is the yii\widgets\ActiveForm
widget, which simplifies the process significantly. This widget automatically generates HTML for your form fields based on your model's attributes and their validation rules.
Let's illustrate with an example. Suppose you have a ContactForm
model:
<?php namespace app\models; use yii\base\Model; class ContactForm extends Model { public $name; public $email; public $subject; public $body; public function rules() { return [ [['name', 'email', 'subject', 'body'], 'required'], ['email', 'email'], ]; } }
In your view, you would use ActiveForm
like this:
<?php $form = \yii\widgets\ActiveForm::begin(); ?> <?= $form->field($model, 'name')->textInput() ?> <?= $form->field($model, 'email')->textInput() ?> <?= $form->field($model, 'subject')->textInput() ?> <?= $form->field($model, 'body')->textarea(['rows' => 6]) ?> <div class="form-group"> <?= Html::submitButton('Submit', ['class' => 'btn btn-primary']) ?> </div> <?php \yii\widgets\ActiveForm::end(); ?>
This generates a form with input fields for each attribute. The rules()
method in the model defines the validation rules. When the form is submitted, $model->validate()
will check the input against these rules. Error messages are automatically displayed next to the respective fields if validation fails. You can access validated data via $model->attributes
. Remember to handle form submission in your controller action.
Best Practices for Securing Forms in Yii
Securing forms in Yii involves several crucial steps:
- Input Validation: Always validate user input on the server-side, regardless of client-side validation. Never trust data coming from the client. Yii's built-in validation rules are essential for this.
-
Output Encoding: Prevent Cross-Site Scripting (XSS) attacks by encoding user-supplied data before displaying it on the page. Yii's
Html::encode()
function is your friend. Use it to escape HTML characters in any data you display from user input. - SQL Injection Prevention: Use parameterized queries or active record to interact with your database. Avoid directly concatenating user input into SQL queries. Yii's ActiveRecord provides this protection automatically.
-
Cross-Site Request Forgery (CSRF) Protection: Implement CSRF protection using Yii's built-in CSRF validation. This typically involves including a hidden CSRF token in your forms and verifying it on submission. Yii's
yii\web\CsrfToken
component handles this automatically. Ensure you useyii\widgets\ActiveForm
as it automatically includes CSRF protection. -
Mass Assignment Protection: Be mindful of mass assignment vulnerabilities. If you're using ActiveRecord, carefully define the
safeAttributes()
method in your model to specify which attributes are safe to be mass-assigned. - Regular Security Audits: Regularly audit your code for potential vulnerabilities. Keep your Yii framework and its extensions up-to-date to benefit from security patches.
Integrating Form Data with Database Operations in Yii
Yii simplifies database interactions through its ActiveRecord. After validating user input, you can save the data to your database using ActiveRecord's save()
method.
Assuming you have a Contact
model corresponding to a database table, you could do this:
if ($model->load(Yii::$app->request->post()) && $model->validate()) { if ($model->save()) { // Success! Send a confirmation email, etc. } else { // Handle save errors } }
This code first loads the submitted data into the model using load()
. Then, it validates the data. If validation succeeds, it attempts to save the data to the database. The save()
method handles the database interaction, including handling potential database errors.
Implementing Client-Side Validation in Yii Forms
Client-side validation enhances the user experience by providing immediate feedback. Yii integrates seamlessly with JavaScript frameworks like jQuery to achieve this. yii\widgets\ActiveForm
automatically generates client-side validation code based on your model's rules.
You don't need to write much extra code for basic client-side validation; ActiveForm
handles most of it automatically. For more complex scenarios, you can customize the client-side validation logic by using the validate()
method of the ActiveForm
widget and integrating with custom JavaScript functions. However, always remember that client-side validation should be considered a supplementary measure and never a replacement for robust server-side validation.
The above is the detailed content of How do I work with forms in Yii and handle user input validation?. For more information, please follow other related articles on the PHP Chinese website!
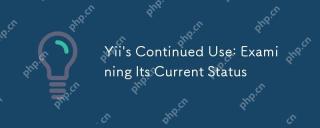
Yii is still competitive in modern development. 1) High performance: adopts lazy loading and caching mechanisms. 2) Security: Built-in CSRF and SQL injection protection. 3) Extensibility: Component-based design is easy to expand and customize.
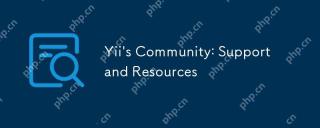
The Yii community provides rich support and resources. 1. Visit the official website and GitHub to get the documentation and code. 2. Use official forums and StackOverflow to solve technical problems. 3. Report bugs and make suggestions through GitHubIssues. 4. Use documents and tutorials to learn the Yii framework.
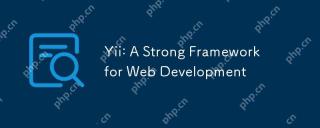
Yii is a high-performance PHP framework designed for fast development and efficient code generation. Its core features include: MVC architecture: Yii adopts MVC architecture to help developers separate application logic and make the code easier to maintain and expand. Componentization and code generation: Through componentization and code generation, Yii reduces the repetitive work of developers and improves development efficiency. Performance Optimization: Yii uses latency loading and caching technologies to ensure efficient operation under high loads and provides powerful ORM capabilities to simplify database operations.
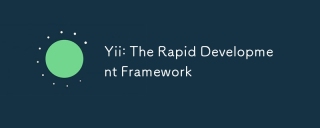
Yii is a high-performance framework based on PHP, suitable for rapid development of web applications. 1) It adopts MVC architecture and component design to simplify the development process. 2) Yii provides rich functions, such as ActiveRecord, RESTfulAPI, etc., which supports high concurrency and expansion. 3) Using Gii tools can quickly generate CRUD code and improve development efficiency. 4) During debugging, you can check configuration files, use debugging tools and view logs. 5) Performance optimization suggestions include using cache, optimizing database queries and maintaining code readability.
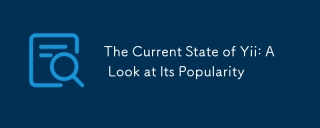
YiiremainspopularbutislessfavoredthanLaravel,withabout14kGitHubstars.ItexcelsinperformanceandActiveRecord,buthasasteeperlearningcurveandasmallerecosystem.It'sidealfordevelopersprioritizingefficiencyoveravastecosystem.
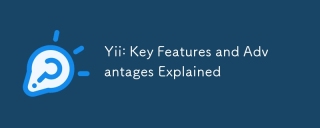
Yii is a high-performance PHP framework that is unique in its componentized architecture, powerful ORM and excellent security. 1. The component-based architecture allows developers to flexibly assemble functions. 2. Powerful ORM simplifies data operation. 3. Built-in multiple security functions to ensure application security.
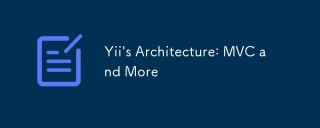
Yii framework adopts an MVC architecture and enhances its flexibility and scalability through components, modules, etc. 1) The MVC mode divides the application logic into model, view and controller. 2) Yii's MVC implementation uses action refinement request processing. 3) Yii supports modular development and improves code organization and management. 4) Use cache and database query optimization to improve performance.
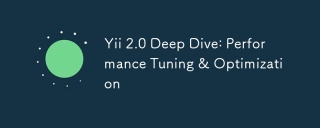
Strategies to improve Yii2.0 application performance include: 1. Database query optimization, using QueryBuilder and ActiveRecord to select specific fields and limit result sets; 2. Caching strategy, rational use of data, query and page cache; 3. Code-level optimization, reducing object creation and using efficient algorithms. Through these methods, the performance of Yii2.0 applications can be significantly improved.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
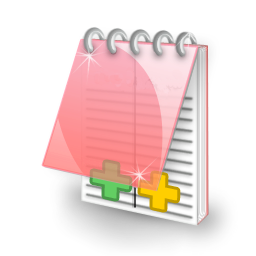
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version

Zend Studio 13.0.1
Powerful PHP integrated development environment