


What are promises in JavaScript, and how can they be used to handle asynchronous operations?
What are promises in JavaScript, and how can they be used to handle asynchronous operations?
Understanding JavaScript Promises
In JavaScript, a Promise is an object representing the eventual completion (or failure) of an asynchronous operation, and its resulting value. Instead of directly returning a value, an asynchronous function returns a Promise. This Promise acts as a placeholder for the eventual result. The key benefit is that it provides a cleaner, more manageable way to handle asynchronous operations compared to traditional callback functions.
Handling Asynchronous Operations with Promises
A Promise can be in one of three states:
- Pending: The initial state, neither fulfilled nor rejected.
- Fulfilled: The operation completed successfully. The Promise now holds a resolved value.
- Rejected: The operation failed. The Promise now holds a reason for the failure (usually an error object).
Promises utilize the .then()
method to handle successful completion and the .catch()
method to handle rejection. The .then()
method takes two arguments: a function to execute if the Promise is fulfilled, and an optional function to execute if the Promise is rejected (though this is better handled with .catch()
for clarity). The .catch()
method specifically handles rejected Promises.
Here's a simple example:
function fetchData(url) { return new Promise((resolve, reject) => { fetch(url) .then(response => response.json()) .then(data => resolve(data)) .catch(error => reject(error)); }); } fetchData('https://api.example.com/data') .then(data => { console.log('Data received:', data); }) .catch(error => { console.error('Error fetching data:', error); });
This example demonstrates how fetchData
returns a Promise. The .then()
method handles the successful retrieval of JSON data, and .catch()
handles any errors during the fetch process. This structured approach significantly improves the readability and manageability of asynchronous code.
How do JavaScript promises improve code readability and maintainability compared to callbacks?
Promises vs. Callbacks: A Readability and Maintainability Comparison
Callbacks, while functional, often lead to nested structures known as "callback hell" when dealing with multiple asynchronous operations. This deeply nested structure makes code difficult to read, understand, and maintain. Promises offer a significant improvement in this regard.
Improved Readability: Promises utilize a cleaner, linear structure. Instead of nested callbacks, .then()
methods chain together sequentially, making the flow of asynchronous operations much easier to follow. The code becomes more declarative and easier to visually parse.
Enhanced Maintainability: The linear structure of Promise chains also improves maintainability. Adding or modifying asynchronous operations within a Promise chain is simpler and less error-prone than modifying deeply nested callbacks. The clear separation of success and error handling (via .then()
and .catch()
) also makes debugging and troubleshooting much easier.
Consider this example illustrating the difference:
Callback Hell:
fetchData(url1, (data1) => { fetchData(url2, (data2) => { fetchData(url3, (data3) => { // Process data1, data2, data3 }, error3 => { // Handle error3 }); }, error2 => { // Handle error2 }); }, error1 => { // Handle error1 });
Promise Chain:
fetchData(url1) .then(data1 => fetchData(url2)) .then(data2 => fetchData(url3)) .then(data3 => { // Process data1, data2, data3 }) .catch(error => { // Handle errors from any fetchData call });
The Promise-based approach is clearly more readable and maintainable.
What are the common methods used with JavaScript promises, and how do they work in practice?
Common Promise Methods and Their Practical Applications
Beyond .then()
and .catch()
, several other useful methods enhance Promise functionality:
-
.then(onFulfilled, onRejected)
: As discussed earlier, this method handles the fulfilled and (optionally) rejected states of a Promise.onFulfilled
receives the resolved value, whileonRejected
receives the reason for rejection. -
.catch(onRejected)
: This is a shorthand for.then(null, onRejected)
, specifically designed for handling rejected Promises. It simplifies error handling by centralizing rejection handling. -
.finally(onFinally)
: This method executes a callback function regardless of whether the Promise was fulfilled or rejected. It's useful for cleanup tasks, such as closing connections or releasing resources. -
Promise.all([promise1, promise2, ...])
: This method takes an array of Promises and returns a new Promise that resolves when all input Promises have resolved. The resolved value is an array of the resolved values from the input Promises. If any input Promise rejects, the resulting Promise also rejects. -
Promise.race([promise1, promise2, ...])
: This method takes an array of Promises and returns a new Promise that resolves or rejects as soon as one of the input Promises resolves or rejects. -
Promise.resolve(value)
: Creates a Promise that is immediately resolved with the givenvalue
. -
Promise.reject(reason)
: Creates a Promise that is immediately rejected with the givenreason
.
Practical Examples:
// Promise.all Promise.all([fetchData('url1'), fetchData('url2')]) .then(results => { console.log('Data from both URLs:', results); }) .catch(error => { console.error('Error fetching data:', error); }); // Promise.race Promise.race([fetchData('url1'), fetchData('url2')]) //Faster URL wins .then(result => { console.log('First data received:', result); }) .catch(error => console.error(error)); // Promise.finally fetchData('url') .then(data => console.log(data)) .catch(error => console.error(error)) .finally(() => console.log('Data fetching complete'));
What are the best practices for using promises to avoid common pitfalls in asynchronous JavaScript programming?
Best Practices for Using Promises
Avoiding common pitfalls when working with Promises requires mindful coding practices:
-
Always handle both fulfillment and rejection: Never rely solely on
.then()
. Always include a.catch()
block to handle potential errors gracefully. Ignoring errors can lead to unexpected application behavior. -
Avoid deeply nested
.then()
chains: Excessive nesting can make code hard to read and maintain. Consider using async/await (a more modern approach built on top of Promises) to flatten the structure. -
Use
Promise.all()
for parallel operations: When multiple asynchronous operations are independent, usePromise.all()
to run them concurrently and improve performance. -
Handle errors centrally: Instead of handling errors in each
.then()
block, consolidate error handling in a single.catch()
block at the end of the chain. -
Use
try...catch
blocks within async functions (when using async/await): This allows for more structured error handling within asynchronous code. -
Don't forget
Promise.finally()
: Utilizefinally
for cleanup tasks that should always execute, regardless of success or failure. - Be mindful of the order of operations: Promises execute asynchronously. Ensure your code correctly handles the order of dependent operations.
- Use descriptive variable and function names: Clear naming conventions improve code readability and maintainability.
- Consider using a library like Axios: Axios simplifies making HTTP requests and provides built-in Promise handling.
By adhering to these best practices, developers can harness the power of Promises effectively while mitigating potential issues in asynchronous JavaScript programming. Remember that clear, well-structured code is paramount for building robust and maintainable applications.
The above is the detailed content of What are promises in JavaScript, and how can they be used to handle asynchronous operations?. For more information, please follow other related articles on the PHP Chinese website!
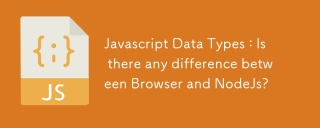
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
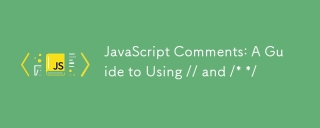
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
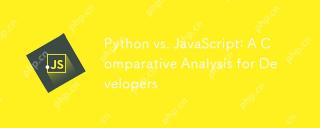
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
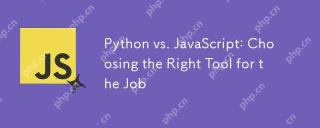
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
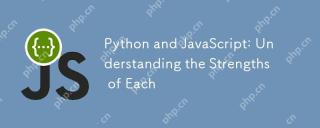
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
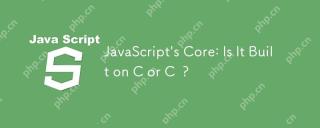
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
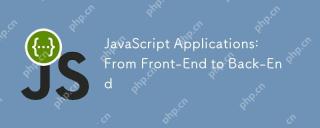
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
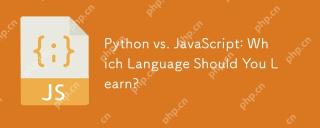
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
