How to Use Views in MySQL to Simplify Complex Queries
MySQL views provide a powerful mechanism for simplifying complex queries by encapsulating them into named, virtual tables. Instead of repeatedly writing the same lengthy or intricate SQL statement, you can create a view that represents the result of that query. Subsequently, you can query the view as if it were a regular table, making your interactions with the database much cleaner and more maintainable.
Let's say you have a complex query involving joins across multiple tables to retrieve specific customer order information:
SELECT c.customer_name, o.order_id, oi.item_name, oi.quantity FROM Customers c JOIN Orders o ON c.customer_id = o.customer_id JOIN OrderItems oi ON o.order_id = oi.order_id WHERE o.order_date >= '2023-01-01';
This query is relatively straightforward, but it could become much more complex with additional joins and conditions. To simplify this, you can create a view:
CREATE VIEW CustomerOrderSummary AS SELECT c.customer_name, o.order_id, oi.item_name, oi.quantity FROM Customers c JOIN Orders o ON c.customer_id = o.customer_id JOIN OrderItems oi ON o.order_id = oi.order_id WHERE o.order_date >= '2023-01-01';
Now, you can query this view:
SELECT * FROM CustomerOrderSummary;
This is significantly easier to read and understand than the original complex query. The view abstracts away the underlying complexity, making your application logic cleaner and less prone to errors. You can also create views on top of other views, building up layers of abstraction.
What Are the Performance Implications of Using Views in MySQL?
The performance impact of using views in MySQL depends on several factors, primarily the complexity of the underlying query and how the view is used. In some cases, views can improve performance, while in others, they can degrade it.
Potential Performance Benefits:
- Caching: The MySQL query optimizer might cache the results of a view's underlying query, especially if the view is frequently accessed and the underlying data doesn't change often. This can lead to faster query execution times.
- Simplified Queries: As discussed above, views simplify complex queries, potentially leading to more efficient query plans generated by the optimizer. A simpler query might be easier for the optimizer to optimize.
Potential Performance Drawbacks:
- Query Rewriting: MySQL needs to rewrite queries against a view to access the underlying tables. This rewriting process adds overhead. The more complex the view's underlying query, the greater the overhead.
- Materialized Views (Not Standard MySQL): Unlike some other database systems, standard MySQL views are not materialized. This means that the underlying query is executed every time the view is accessed. Materialized views, which store the results of the underlying query, can significantly improve performance but require more storage space and need to be refreshed periodically. MySQL offers some functionality that approximates materialized views through techniques like caching and indexing, but doesn't have built-in materialized views like some other databases.
- Inefficient Underlying Queries: If the underlying query of a view is inefficient, the view will inherit this inefficiency. It's crucial to ensure that the base query used to create a view is well-optimized.
Can I Use Views in MySQL to Improve Data Security by Restricting Access to Underlying Tables?
Yes, views can be used to enhance data security in MySQL by restricting access to the underlying tables. You can create views that expose only a subset of columns or rows from the base tables, effectively hiding sensitive information from users who only need access to a limited view of the data.
For instance, suppose you have a table containing employee salary information, but you only want certain users to see employee names and departments, not their salaries. You could create a view that excludes the salary column:
CREATE VIEW EmployeeSummary AS SELECT employee_name, department FROM Employees;
Users granted access to this view can only see the employee name and department, not the salary, even if they have broader privileges on the underlying Employees
table. This provides a layer of data security by restricting access to sensitive information based on user roles and permissions.
How Can I Update Data Through a View in MySQL?
The ability to update data through a view in MySQL depends heavily on the complexity of the underlying query used to define the view. Not all views are updatable. MySQL allows updates through views only under specific conditions:
-
Simple Views: Views based on a single table, without any aggregation functions (like
SUM
,AVG
,COUNT
), and with all columns from the base table, are typically updatable. -
Insertable Views: You can insert new rows into the underlying table via a view, but only if the view contains all columns of the underlying table that have
NOT NULL
constraints. -
Updatable Views: You can update existing rows in the underlying table via a view, but this is possible only under similar conditions to insertable views. The view must select all columns from a single table that have
NOT NULL
constraints and it must not use any aggregation functions.
If the view involves joins, subqueries, or aggregate functions, updates through the view are usually not allowed. Attempting to update data through a non-updatable view will result in an error. In such cases, you must update the underlying tables directly. Always check the specific view definition to determine its updatability using commands like SHOW CREATE VIEW
. Complex views often require direct manipulation of the underlying tables for updates.
The above is the detailed content of How do I use views in MySQL to simplify complex queries?. For more information, please follow other related articles on the PHP Chinese website!
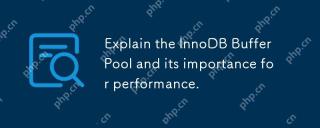
InnoDBBufferPool reduces disk I/O by caching data and indexing pages, improving database performance. Its working principle includes: 1. Data reading: Read data from BufferPool; 2. Data writing: After modifying the data, write to BufferPool and refresh it to disk regularly; 3. Cache management: Use the LRU algorithm to manage cache pages; 4. Reading mechanism: Load adjacent data pages in advance. By sizing the BufferPool and using multiple instances, database performance can be optimized.
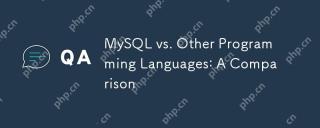
Compared with other programming languages, MySQL is mainly used to store and manage data, while other languages such as Python, Java, and C are used for logical processing and application development. MySQL is known for its high performance, scalability and cross-platform support, suitable for data management needs, while other languages have advantages in their respective fields such as data analytics, enterprise applications, and system programming.
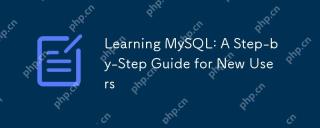
MySQL is worth learning because it is a powerful open source database management system suitable for data storage, management and analysis. 1) MySQL is a relational database that uses SQL to operate data and is suitable for structured data management. 2) The SQL language is the key to interacting with MySQL and supports CRUD operations. 3) The working principle of MySQL includes client/server architecture, storage engine and query optimizer. 4) Basic usage includes creating databases and tables, and advanced usage involves joining tables using JOIN. 5) Common errors include syntax errors and permission issues, and debugging skills include checking syntax and using EXPLAIN commands. 6) Performance optimization involves the use of indexes, optimization of SQL statements and regular maintenance of databases.
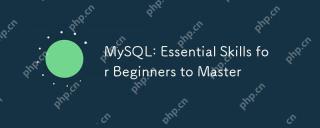
MySQL is suitable for beginners to learn database skills. 1. Install MySQL server and client tools. 2. Understand basic SQL queries, such as SELECT. 3. Master data operations: create tables, insert, update, and delete data. 4. Learn advanced skills: subquery and window functions. 5. Debugging and optimization: Check syntax, use indexes, avoid SELECT*, and use LIMIT.
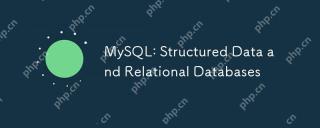
MySQL efficiently manages structured data through table structure and SQL query, and implements inter-table relationships through foreign keys. 1. Define the data format and type when creating a table. 2. Use foreign keys to establish relationships between tables. 3. Improve performance through indexing and query optimization. 4. Regularly backup and monitor databases to ensure data security and performance optimization.
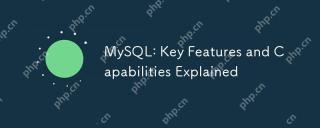
MySQL is an open source relational database management system that is widely used in Web development. Its key features include: 1. Supports multiple storage engines, such as InnoDB and MyISAM, suitable for different scenarios; 2. Provides master-slave replication functions to facilitate load balancing and data backup; 3. Improve query efficiency through query optimization and index use.
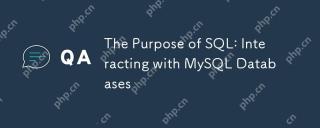
SQL is used to interact with MySQL database to realize data addition, deletion, modification, inspection and database design. 1) SQL performs data operations through SELECT, INSERT, UPDATE, DELETE statements; 2) Use CREATE, ALTER, DROP statements for database design and management; 3) Complex queries and data analysis are implemented through SQL to improve business decision-making efficiency.
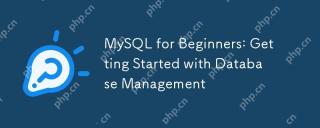
The basic operations of MySQL include creating databases, tables, and using SQL to perform CRUD operations on data. 1. Create a database: CREATEDATABASEmy_first_db; 2. Create a table: CREATETABLEbooks(idINTAUTO_INCREMENTPRIMARYKEY, titleVARCHAR(100)NOTNULL, authorVARCHAR(100)NOTNULL, published_yearINT); 3. Insert data: INSERTINTObooks(title, author, published_year)VA


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
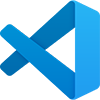
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft