This article discusses optimizing SQL joins for performance. Key strategies include choosing the right join type (INNER JOIN preferred), creating appropriate indexes, filtering before joining, and avoiding common pitfalls like Cartesian products and
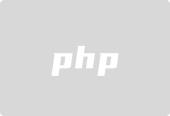
How Do I Optimize Joins for Performance in SQL?
Optimizing joins for performance in SQL involves several strategies aimed at minimizing the amount of data processed and the number of comparisons made. Here's a breakdown of key techniques:
-
Choosing the Right Join Type: Selecting the most appropriate join type (INNER, LEFT, RIGHT, FULL OUTER) is crucial. Unnecessary data retrieval associated with less restrictive join types like
FULL OUTER JOIN
can significantly impact performance. If you only need matching data, stick with INNER JOIN
.
-
Indexing: Properly indexed columns used in join conditions are essential. Indexes allow the database to quickly locate matching rows without resorting to full table scans. Create indexes on the columns involved in the
ON
clause of your JOIN
statements, particularly on the smaller table's join column. Consider composite indexes if multiple columns are used in the join condition.
-
Filtering Before Joining: Apply
WHERE
clauses to filter data before the join occurs. This reduces the amount of data involved in the join operation itself, leading to faster processing. Pre-filtering can dramatically decrease the size of intermediate result sets.
-
Using Hints (with caution): Some database systems allow the use of query hints to influence the optimizer's choices. These hints can force the use of specific join algorithms or access paths. However, using hints should be done cautiously and only after careful profiling and benchmarking, as they can sometimes hinder the optimizer's ability to choose the optimal plan.
-
Optimizing Table Structures: Ensure your tables are properly normalized. Avoid redundant data, as this can lead to larger table sizes and slower join operations.
-
Data Type Matching: Ensure that data types used in join conditions are compatible and efficiently comparable. Implicit data type conversions can slow down the join process.
What Are the Common Pitfalls to Avoid When Using Joins in SQL?
Several common mistakes can significantly degrade the performance of SQL joins:
-
Cartesian Products: Failing to specify a join condition can lead to a Cartesian product (cross join), where every row from one table is joined with every row from another. This results in an explosion of data and extremely slow query execution. Always ensure a proper
ON
clause is present in your joins.
-
Inefficient Join Ordering: The order in which joins are performed can impact performance. The database optimizer usually handles this, but in complex queries, analyzing and potentially rearranging the join order can be beneficial.
-
Missing or Ineffective Indexes: As mentioned above, the absence of appropriate indexes on columns used in join conditions is a major performance bottleneck. Furthermore, poorly chosen indexes (e.g., indexes on columns rarely used in
WHERE
clauses) can actually hinder performance.
-
Ignoring Data Volume: Joining large tables without proper optimization strategies can lead to excessive resource consumption and slow query execution. Consider partitioning or sharding large tables to improve join performance.
-
Unnecessary Joins: Sometimes, joins are used when simpler subqueries or other techniques could achieve the same result more efficiently. Review your queries to ensure each join is truly necessary.
-
Lack of Proper Query Analysis: Not using database profiling tools to identify performance bottlenecks related to joins can lead to inefficient query optimization efforts.
Which Join Type Is Most Efficient for Different Database Scenarios?
The most efficient join type depends heavily on the specific scenario and the desired outcome. Generally:
-
INNER JOIN: This is often the most efficient when you only need rows where the join condition is met in both tables. It avoids processing unmatched rows, leading to faster execution.
-
LEFT (OUTER) JOIN: More computationally expensive than
INNER JOIN
because it includes all rows from the left table, even if there's no match in the right table. Use this when you need all rows from the left table and matching rows from the right.
-
RIGHT (OUTER) JOIN: Similar to
LEFT JOIN
, but it includes all rows from the right table, even if there's no match in the left.
-
FULL (OUTER) JOIN: The most computationally expensive join type. It returns all rows from both tables, regardless of whether there's a match in the other table. Use only when absolutely necessary, as it can be significantly slower than other join types.
How Can I Identify and Resolve Performance Bottlenecks Caused by Inefficient Joins in My SQL Queries?
Identifying and resolving performance bottlenecks from inefficient joins involves a multi-step process:
-
Query Profiling: Use your database system's built-in profiling tools to analyze the execution plan of your queries. This will reveal which parts of the query are consuming the most resources, often highlighting inefficient joins.
-
Execution Plan Analysis: Examine the execution plan to identify full table scans, which indicate a lack of suitable indexes. Look for nested loop joins, which can be inefficient for large tables.
-
Indexing Optimization: Based on the execution plan analysis, create or optimize indexes on the columns used in join conditions. Consider composite indexes if multiple columns are involved.
-
Join Type Selection: Review the join types used in your queries. If a
FULL OUTER JOIN
or LEFT/RIGHT JOIN
is used when an INNER JOIN
would suffice, consider switching to the more efficient option.
-
Data Filtering: Implement
WHERE
clauses to filter data before joining, reducing the amount of data processed.
-
Query Rewriting: Consider rewriting your queries to improve their efficiency. This might involve using subqueries, common table expressions (CTEs), or other techniques to optimize the join process.
-
Database Tuning: In some cases, database-level tuning might be necessary to improve join performance. This could involve adjusting buffer pool sizes, increasing memory allocation, or other database-specific optimizations.
-
Monitoring and Iteration: Continuously monitor your query performance and iterate on your optimization strategies. Performance can change over time as data volume grows, so regular review is crucial.
The above is the detailed content of How do I optimize joins for performance in SQL?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn