This article explains SQL scalar and table-valued functions. Scalar functions return single values per row, while table-valued functions return result sets. Choosing between them depends on whether the task requires single or multiple row outputs,
What are the different types of functions in SQL (scalar, table-valued)?
SQL functions broadly fall into two categories: scalar functions and table-valued functions. Understanding the distinction is crucial for efficient database design and query optimization.
Scalar Functions: These functions operate on a single row of data and return a single value. They take input parameters (zero or more) and produce a single output value for each row processed. Think of them as analogous to standard mathematical functions – they take input, perform a calculation, and return a single result. Examples include functions that calculate the square root of a number, convert a string to uppercase, or determine the length of a string. Scalar functions are commonly used in SELECT
, WHERE
, HAVING
, and ORDER BY
clauses within SQL queries.
Table-Valued Functions (TVFs): Unlike scalar functions, TVFs return a result set – essentially a table – rather than a single value. They can take input parameters and process multiple rows of data to generate a table as the output. This is particularly useful when you need to perform more complex operations that involve returning multiple rows of data based on the input parameters. For instance, a TVF might return a list of all products from a specific category, or all customers within a particular geographic region. TVFs can be used in FROM
clauses of SQL queries, just like regular tables. They are often more efficient than using multiple scalar functions or complex subqueries to achieve the same result, especially when dealing with large datasets. There are two main types of TVFs: inline and multi-statement. Inline TVFs are defined within a single RETURN
statement, whereas multi-statement TVFs can contain multiple SQL statements.
How do I choose the appropriate SQL function type for a specific task?
The choice between a scalar and table-valued function depends entirely on the nature of the task and the desired output.
- Choose a scalar function if: You need to perform a calculation or transformation on a single row and return a single value for each row. If your operation is simple and only requires processing one row at a time to produce a single output, a scalar function is the appropriate choice.
-
Choose a table-valued function if: You need to perform an operation that returns multiple rows of data. If your operation involves processing multiple rows or generating a result set, a TVF is the better option. Consider using a TVF if you are performing complex logic or retrieving data from multiple tables, as it often leads to more readable and maintainable code compared to using multiple scalar functions or nested queries. This is especially true when dealing with tasks that would otherwise require joining multiple tables or using subqueries within a
SELECT
statement. TVFs can significantly simplify complex data retrieval processes.
What are the performance implications of using different SQL function types?
The performance implications of using scalar versus table-valued functions can be significant, particularly with large datasets.
- Scalar Functions: While simple to use, scalar functions can be less efficient than TVFs when used repeatedly within a query. This is because the scalar function is called for each row processed by the query. This can lead to performance degradation, especially when dealing with millions of rows. The repeated execution of the function adds significant overhead.
- Table-Valued Functions: TVFs generally offer better performance for complex operations returning multiple rows. Because the function executes once and returns a result set, the database engine can optimize the execution plan more effectively. This is especially true for inline TVFs, which are often compiled and optimized as part of the main query. Multi-statement TVFs might have slightly lower performance than inline TVFs, but they still offer advantages over multiple scalar function calls in many cases. The database engine can perform operations on the entire result set returned by the TVF, leading to improved efficiency.
Can I create my own custom SQL functions, and if so, how?
Yes, you can create your own custom SQL functions. The syntax varies slightly depending on the specific database system (e.g., SQL Server, MySQL, PostgreSQL), but the general principles remain the same. Below is an example of creating a scalar function and a table-valued function in SQL Server (T-SQL):
Scalar Function Example (SQL Server):
CREATE FUNCTION dbo.GetFullName (@FirstName VARCHAR(50), @LastName VARCHAR(50)) RETURNS VARCHAR(100) AS BEGIN RETURN @FirstName ' ' @LastName; END;
This function takes two input parameters (@FirstName
and @LastName
) and returns a single string value representing the full name.
Table-Valued Function Example (SQL Server):
CREATE FUNCTION dbo.GetProductsByCategory (@Category VARCHAR(50)) RETURNS @Products TABLE ( ProductID INT, ProductName VARCHAR(100), Price DECIMAL(10, 2) ) AS BEGIN INSERT INTO @Products (ProductID, ProductName, Price) SELECT ProductID, ProductName, Price FROM Products WHERE Category = @Category; RETURN; END;
This function takes a category name as input and returns a table containing product information for that category. Note the use of a table variable @Products
to hold the result set.
Remember to adapt the syntax to your specific database system's dialect when creating your custom functions. Always thoroughly test your functions to ensure they produce the correct results and perform efficiently.
The above is the detailed content of What are the different types of functions in SQL (scalar, table-valued)?. For more information, please follow other related articles on the PHP Chinese website!

SQL is a language used to manage and operate relational databases. 1. Create a table: Use CREATETABLE statements, such as CREATETABLEusers(idINTPRIMARYKEY, nameVARCHAR(100), emailVARCHAR(100)); 2. Insert, update, and delete data: Use INSERTINTO, UPDATE, DELETE statements, such as INSERTINTOusers(id, name, email)VALUES(1,'JohnDoe','john@example.com'); 3. Query data: Use SELECT statements, such as SELEC

The relationship between SQL and MySQL is: SQL is a language used to manage and operate databases, while MySQL is a database management system that supports SQL. 1.SQL allows CRUD operations and advanced queries of data. 2.MySQL provides indexing, transactions and locking mechanisms to improve performance and security. 3. Optimizing MySQL performance requires attention to query optimization, database design and monitoring and maintenance.

SQL is used for database management and data operations, and its core functions include CRUD operations, complex queries and optimization strategies. 1) CRUD operation: Use INSERTINTO to create data, SELECT reads data, UPDATE updates data, and DELETE deletes data. 2) Complex query: Process complex data through GROUPBY and HAVING clauses. 3) Optimization strategy: Use indexes, avoid full table scanning, optimize JOIN operations and paging queries to improve performance.

SQL is suitable for beginners because it is simple in syntax, powerful in function, and widely used in database systems. 1.SQL is used to manage relational databases and organize data through tables. 2. Basic operations include creating, inserting, querying, updating and deleting data. 3. Advanced usage such as JOIN, subquery and window functions enhance data analysis capabilities. 4. Common errors include syntax, logic and performance issues, which can be solved through inspection and optimization. 5. Performance optimization suggestions include using indexes, avoiding SELECT*, using EXPLAIN to analyze queries, normalizing databases, and improving code readability.

In practical applications, SQL is mainly used for data query and analysis, data integration and reporting, data cleaning and preprocessing, advanced usage and optimization, as well as handling complex queries and avoiding common errors. 1) Data query and analysis can be used to find the most sales product; 2) Data integration and reporting generate customer purchase reports through JOIN operations; 3) Data cleaning and preprocessing can delete abnormal age records; 4) Advanced usage and optimization include using window functions and creating indexes; 5) CTE and JOIN can be used to handle complex queries to avoid common errors such as SQL injection.

SQL is a standard language for managing relational databases, while MySQL is a specific database management system. SQL provides a unified syntax and is suitable for a variety of databases; MySQL is lightweight and open source, with stable performance but has bottlenecks in big data processing.

The SQL learning curve is steep, but it can be mastered through practice and understanding the core concepts. 1. Basic operations include SELECT, INSERT, UPDATE, DELETE. 2. Query execution is divided into three steps: analysis, optimization and execution. 3. Basic usage is such as querying employee information, and advanced usage is such as using JOIN connection table. 4. Common errors include not using alias and SQL injection, and parameterized query is required to prevent it. 5. Performance optimization is achieved by selecting necessary columns and maintaining code readability.

SQL commands are divided into five categories in MySQL: DQL, DDL, DML, DCL and TCL, and are used to define, operate and control database data. MySQL processes SQL commands through lexical analysis, syntax analysis, optimization and execution, and uses index and query optimizers to improve performance. Examples of usage include SELECT for data queries and JOIN for multi-table operations. Common errors include syntax, logic, and performance issues, and optimization strategies include using indexes, optimizing queries, and choosing the right storage engine.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
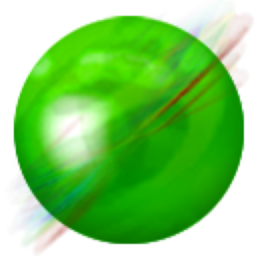
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment