This article details Java's socket API for network communication, covering client-server setup, data handling, and crucial considerations like resource management, error handling, and security. It also explores performance optimization techniques, i
Using Java's Sockets API for Network Communication
Java's java.net
package provides a robust set of classes for network communication, primarily through the Socket
and ServerSocket
classes. To establish a client-server connection, you'll typically follow these steps:
Server-side:
-
Create a ServerSocket: This listens for incoming connections on a specified port. You specify the port number (e.g., 8080) when creating the
ServerSocket
. Example:ServerSocket serverSocket = new ServerSocket(8080);
-
Accept a Connection: The
accept()
method blocks until a client connects. This returns aSocket
object representing the connection. Example:Socket clientSocket = serverSocket.accept();
-
Receive and Send Data: Use
InputStream
andOutputStream
obtained from theSocket
to read and write data. Often, you'll useBufferedReader
andPrintWriter
for text-based communication, orDataInputStream
andDataOutputStream
for binary data. Example:
BufferedReader in = new BufferedReader(new InputStreamReader(clientSocket.getInputStream())); PrintWriter out = new PrintWriter(clientSocket.getOutputStream(), true); String message = in.readLine(); out.println("Server received: " message);
-
Close the Connection: Always close the
Socket
andServerSocket
usingclose()
to release resources. Example:clientSocket.close(); serverSocket.close();
Client-side:
-
Create a Socket: This connects to the server on a specified IP address and port. Example:
Socket socket = new Socket("localhost", 8080);
-
Send and Receive Data: Similar to the server, use
InputStream
andOutputStream
to communicate. -
Close the Connection: Close the
Socket
usingclose()
.
Common Pitfalls to Avoid When Using Java Sockets
Several common issues can arise when working with Java sockets:
-
Resource Leaks: Failing to close sockets properly leads to resource exhaustion. Always use
finally
blocks or try-with-resources to ensure closure, even if exceptions occur. -
Blocking Operations:
accept()
andread()
methods can block indefinitely if no connection or data is available. Use timeouts or asynchronous I/O (e.g., usingjava.nio
) to avoid blocking. - Incorrect Error Handling: Network operations can fail for various reasons (e.g., connection refused, network timeout). Implement proper exception handling to gracefully manage errors and prevent application crashes.
-
Ignoring
shutdownOutput()
: Before closing a socket, it's crucial to callshutdownOutput()
on theSocket
'sOutputStream
to signal the peer that no more data will be sent. This prevents unexpected behavior and ensures clean closure. -
Inefficient Data Handling: Reading and writing data in small chunks can be inefficient. Use buffered streams (
BufferedReader
,BufferedWriter
) for better performance. - Security Vulnerabilities: Using sockets without proper security measures can expose your application to attacks. Always validate inputs and consider using SSL/TLS for secure communication.
Improving the Performance of My Java Socket-Based Application
Optimizing the performance of a Java socket application involves several strategies:
-
Using Non-Blocking I/O: The
java.nio
package provides non-blocking I/O capabilities, allowing your application to handle multiple connections concurrently without blocking on individual I/O operations. This significantly improves scalability and responsiveness. -
Thread Pooling: For handling multiple clients, use a thread pool (e.g.,
ExecutorService
) to manage threads efficiently, avoiding the overhead of creating and destroying threads for each connection. - Efficient Data Serialization: Choose an efficient data serialization method (e.g., Protocol Buffers, Avro) instead of relying on simple text-based protocols, especially for large datasets.
- Connection Pooling: For frequently used connections, a connection pool can reduce the overhead of establishing new connections each time.
- Buffering: Using sufficiently large buffers for reading and writing data minimizes the number of system calls, improving performance.
- Asynchronous Programming: Utilizing asynchronous programming models (e.g., using CompletableFuture) can help avoid blocking and improve overall responsiveness.
Using Java Sockets to Create a Secure Connection (e.g., using SSL/TLS)
Yes, Java sockets can be used to create secure connections using SSL/TLS. The javax.net.ssl
package provides classes for this purpose. You'll typically use SSLSocketFactory
to create SSLSocket
objects, which handle the SSL/TLS handshake and encryption.
Here's a basic example of a client-side SSL connection:
SSLSocketFactory sslSocketFactory = (SSLSocketFactory) SSLSocketFactory.getDefault(); SSLSocket socket = (SSLSocket) sslSocketFactory.createSocket("server-address", 443); // 443 is a common HTTPS port // ... send and receive data ... socket.close();
On the server-side, you'll need to use an SSLServerSocketFactory
to create an SSLServerSocket
that listens for secure connections. You'll also need to configure a keystore containing your server's certificate and private key. Proper certificate management is crucial for secure communication. Remember to choose strong cipher suites to enhance security. Libraries like Netty can simplify the process of creating secure socket connections.
The above is the detailed content of How do I use Java's sockets API for network communication?. For more information, please follow other related articles on the PHP Chinese website!
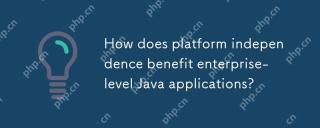
Java is widely used in enterprise-level applications because of its platform independence. 1) Platform independence is implemented through Java virtual machine (JVM), so that the code can run on any platform that supports Java. 2) It simplifies cross-platform deployment and development processes, providing greater flexibility and scalability. 3) However, it is necessary to pay attention to performance differences and third-party library compatibility and adopt best practices such as using pure Java code and cross-platform testing.
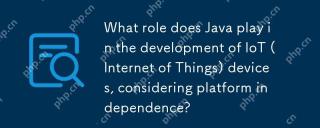
JavaplaysasignificantroleinIoTduetoitsplatformindependence.1)Itallowscodetobewrittenonceandrunonvariousdevices.2)Java'secosystemprovidesusefullibrariesforIoT.3)ItssecurityfeaturesenhanceIoTsystemsafety.However,developersmustaddressmemoryandstartuptim
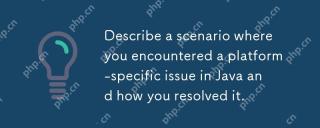
ThesolutiontohandlefilepathsacrossWindowsandLinuxinJavaistousePaths.get()fromthejava.nio.filepackage.1)UsePaths.get()withSystem.getProperty("user.dir")andtherelativepathtoconstructthefilepath.2)ConverttheresultingPathobjecttoaFileobjectifne
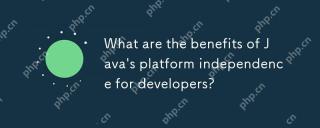
Java'splatformindependenceissignificantbecauseitallowsdeveloperstowritecodeonceandrunitonanyplatformwithaJVM.This"writeonce,runanywhere"(WORA)approachoffers:1)Cross-platformcompatibility,enablingdeploymentacrossdifferentOSwithoutissues;2)Re
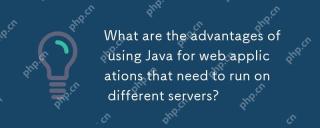
Java is suitable for developing cross-server web applications. 1) Java's "write once, run everywhere" philosophy makes its code run on any platform that supports JVM. 2) Java has a rich ecosystem, including tools such as Spring and Hibernate, to simplify the development process. 3) Java performs excellently in performance and security, providing efficient memory management and strong security guarantees.
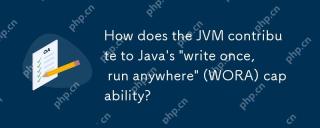
JVM implements the WORA features of Java through bytecode interpretation, platform-independent APIs and dynamic class loading: 1. Bytecode is interpreted as machine code to ensure cross-platform operation; 2. Standard API abstract operating system differences; 3. Classes are loaded dynamically at runtime to ensure consistency.
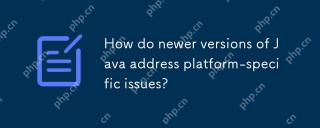
The latest version of Java effectively solves platform-specific problems through JVM optimization, standard library improvements and third-party library support. 1) JVM optimization, such as Java11's ZGC improves garbage collection performance. 2) Standard library improvements, such as Java9's module system reducing platform-related problems. 3) Third-party libraries provide platform-optimized versions, such as OpenCV.
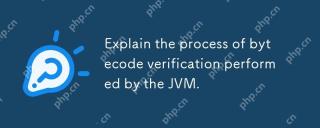
The JVM's bytecode verification process includes four key steps: 1) Check whether the class file format complies with the specifications, 2) Verify the validity and correctness of the bytecode instructions, 3) Perform data flow analysis to ensure type safety, and 4) Balancing the thoroughness and performance of verification. Through these steps, the JVM ensures that only secure, correct bytecode is executed, thereby protecting the integrity and security of the program.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
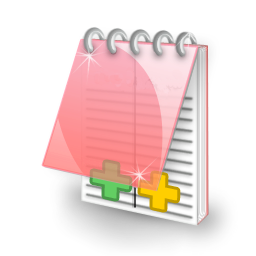
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
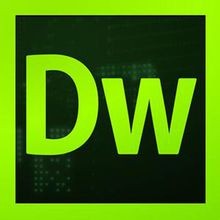
Dreamweaver CS6
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor
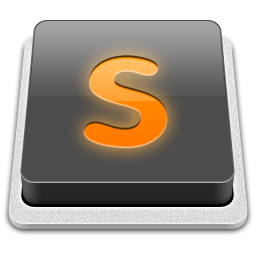
SublimeText3 Mac version
God-level code editing software (SublimeText3)
