This article explains Java's classloading mechanism, a hierarchical, delegation-based system. It details the three built-in classloaders and how to customize loading via custom classloaders. Common issues like ClassNotFoundException and debugging s
How Does Java's Classloading Mechanism Work and How Can I Customize It?
Java's classloading mechanism is a crucial part of its runtime environment. It's responsible for loading class files (.class files) into the Java Virtual Machine (JVM) at runtime. This process isn't a simple one-time load; it's dynamic and hierarchical. The JVM uses a delegation model, typically involving three built-in classloaders:
-
Bootstrap Classloader: This is the primordial classloader, implemented in native code. It loads core Java classes from the
rt.jar
and other essential libraries located in the$JAVA_HOME/lib
directory. You cannot directly access or customize this classloader. -
Extension Classloader: This loads classes from the extension directory, typically
$JAVA_HOME/lib/ext
or locations specified by thejava.ext.dirs
system property. You can indirectly influence this through system properties but cannot directly customize its behavior. - System/Application Classloader: This loads classes from the application's classpath, which is specified when you run the Java application. This is the classloader you most often interact with and can customize.
The delegation model works as follows: When a class is requested, the system classloader first delegates the request to its parent (the extension classloader). If the parent cannot find the class, it delegates to its parent (the bootstrap classloader). Only if the bootstrap classloader cannot find the class will the system classloader attempt to load it from the application's classpath. This ensures that core Java classes are loaded consistently.
Customizing the Classloading Mechanism:
You can customize the classloading mechanism by creating your own custom classloaders. This is done by extending the ClassLoader
class and overriding its loadClass()
method. Within this method, you can implement your own logic for locating and loading classes from various sources, such as network locations, databases, or encrypted files. For example:
public class MyClassLoader extends ClassLoader { @Override protected Class<?> findClass(String name) throws ClassNotFoundException { byte[] classData = loadClassData(name); // Your custom logic to load class data if (classData == null) { throw new ClassNotFoundException(name); } return defineClass(name, classData, 0, classData.length); } private byte[] loadClassData(String name) { // Your implementation to load class data from a custom source // ... return null; // Replace with actual class data } }
This allows for flexible and powerful control over the classloading process, but requires careful consideration to avoid issues like class conflicts and security vulnerabilities.
What Are the Common Problems Encountered During Java Classloading, and How Can I Debug Them?
Several common problems can arise during Java classloading:
- ClassNotFoundException: This is thrown when the JVM cannot find the class specified by its name. This often occurs due to incorrect classpath settings, misspelled class names, or missing JAR files.
- NoClassDefFoundError: This is a runtime error indicating that a class referenced by a loaded class cannot be found. This usually happens when a dependency is missing.
- ClassCastException: This occurs when you try to cast an object to a class it doesn't belong to. This can be related to classloading if different classloaders load different versions of the same class.
-
LinkageError: This is a broader category encompassing errors that occur during the linking phase of classloading (verification, preparation, resolution).
IncompatibleClassChangeError
andVerifyError
are common subclasses.
Debugging Classloading Issues:
Debugging classloading problems requires careful examination of the classpath, system properties, and the classloader hierarchy. Here are some strategies:
-
Check the Classpath: Ensure that all necessary JAR files and directories are included in the classpath. Use
System.out.println(System.getProperty("java.class.path"));
to verify the classpath at runtime. - Use Logging: Add logging statements to your custom classloaders to track the classloading process and identify where problems occur.
- Inspect Classloaders: Use tools like JConsole or VisualVM to inspect the classloader hierarchy and identify which classloader is loading which classes.
- Use a Debugger: Step through your code using a debugger to examine the classloading process in detail.
-
Analyze Stack Traces: Carefully examine stack traces of
ClassNotFoundException
,NoClassDefFoundError
, andClassCastException
to pinpoint the source of the problem.
How Can I Leverage Java's Classloading Mechanism to Improve the Performance of My Application?
Java's classloading mechanism can be leveraged for performance improvements in several ways:
- Lazy Loading: Instead of loading all classes upfront, load classes only when they are needed. This reduces the initial startup time and memory footprint.
- Class Data Sharing (CDS): This feature, available in recent JDK versions, pre-loads frequently used classes into a shared archive. This reduces the time required to load these classes at startup.
- Pre-loading Critical Classes: Identify critical classes that are frequently used and load them proactively. This can reduce the latency associated with loading them later.
- Optimized Classloading Strategies: For large applications, consider using specialized classloaders or techniques to optimize the classloading process. This might involve caching frequently accessed classes or using parallel classloading.
- Avoid Unnecessary Class Reloading: If you're using a framework or technology that dynamically reloads classes, ensure that this is done efficiently and only when absolutely necessary. Frequent class reloading can be costly.
Can I Use Custom Classloaders to Implement Dynamic Class Loading or Modularity in My Java Application?
Yes, custom classloaders are ideally suited for implementing dynamic class loading and modularity in Java applications.
Dynamic Class Loading: Custom classloaders allow you to load classes from various sources at runtime, enabling features like plugin architectures, dynamic updates, and hot swapping of code. This allows your application to adapt and evolve without requiring a restart.
Modularity: By using separate classloaders for different modules or components of your application, you can isolate them from each other. This enhances maintainability, reduces the risk of conflicts, and allows for independent deployment and updates. If one module encounters a problem, it's less likely to affect other modules.
Example (Illustrative):
You could have a custom classloader that loads plugins from a specific directory. Each plugin would be loaded in its own isolated classloader, preventing conflicts with other plugins or the core application. This architecture supports dynamic extension of functionality without restarting the application. This is a common pattern in many Java frameworks and applications that need flexibility and extensibility. However, careful consideration is needed to manage dependencies and avoid classloading conflicts.
The above is the detailed content of How does Java's classloading mechanism work and how can I customize it?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
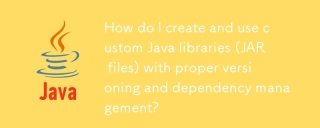
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
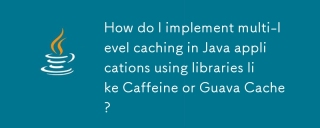
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
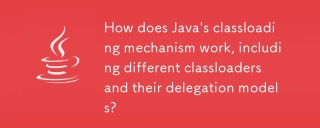
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
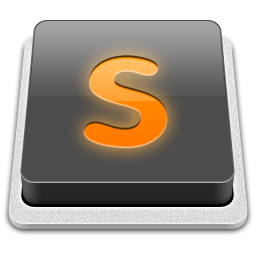
SublimeText3 Mac version
God-level code editing software (SublimeText3)
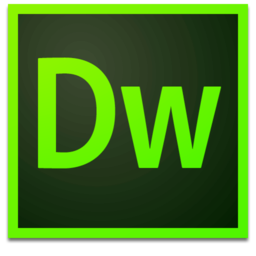
Dreamweaver Mac version
Visual web development tools