


What are Java's memory models and how do they impact multithreaded applications?
This article explores Java's memory model (JMM), focusing on its impact on multithreaded applications. The JMM defines how threads interact with memory, preventing unpredictable behavior through memory barriers and synchronization. Understanding ha
What are Java's memory models and how do they impact multithreaded applications?
Java's Memory Model and its Impact on Multithreaded Applications:
Java's memory model (JMM) defines how threads interact with the main memory and their own local caches. It dictates the rules for how changes made by one thread become visible to other threads. Without a well-defined memory model, multithreaded applications would be unpredictable and prone to errors. The JMM ensures that all threads see a consistent view of memory, albeit with some carefully managed constraints. It achieves this consistency through the use of memory barriers and synchronization primitives.
Crucially, the JMM doesn't guarantee that all threads see the changes made by other threads immediately. Instead, it allows for optimizations like caching and reordering of instructions, which can improve performance. However, these optimizations can lead to unexpected behavior if not carefully managed. The JMM's rules specify happens-before relationships, which define the order in which memory operations must be observed. If operation A happens-before operation B, then any thread will see the effects of A before the effects of B.
The impact on multithreaded applications is significant. Without a clear memory model, race conditions – where multiple threads access and modify the same shared data concurrently, leading to unpredictable results – would be rampant. The JMM helps prevent these issues by providing a framework for managing memory access and ensuring that changes are properly synchronized. However, programmers still need to understand and apply the JMM's rules correctly to avoid subtle concurrency bugs. Ignoring the JMM can lead to data corruption, incorrect program behavior, and extremely difficult-to-debug problems.
How can I avoid common memory-related bugs in concurrent Java programs?
Avoiding Common Memory-Related Bugs in Concurrent Java Programs:
Avoiding memory-related bugs in concurrent Java programs requires a combination of careful coding practices and the proper use of synchronization mechanisms. Here are some key strategies:
-
Use appropriate synchronization primitives:
synchronized
blocks and methods,ReentrantLock
, and other synchronization mechanisms ensure that only one thread accesses a shared resource at a time, preventing race conditions. Choose the right tool for the job;synchronized
is often simpler for smaller critical sections, whileReentrantLock
offers more fine-grained control. - Understand happens-before relationships: Ensure that memory operations are properly ordered using synchronization or volatile variables. Understanding the happens-before relationship allows you to predict the visibility of changes between threads.
- Avoid shared mutable state: Minimize the use of shared mutable state (data that can be changed by multiple threads). Immutable objects eliminate the need for synchronization altogether, significantly simplifying concurrent programming. Consider using immutable data structures where possible.
-
Use thread-safe collections: Java provides thread-safe collections like
ConcurrentHashMap
andCopyOnWriteArrayList
. These collections handle synchronization internally, eliminating the need for manual synchronization. -
Properly utilize volatile variables: Declare variables as
volatile
only when necessary. Avolatile
variable ensures that all threads see the most up-to-date value, but it doesn't provide the same level of atomicity as synchronization. -
Use atomic operations: Java's
java.util.concurrent.atomic
package provides atomic operations that allow thread-safe updates of individual variables without explicit locking. - Thorough testing: Test your concurrent code extensively under various conditions, including high concurrency loads, to identify potential race conditions and other memory-related bugs.
What are the best practices for optimizing memory usage in multithreaded Java applications?
Best Practices for Optimizing Memory Usage in Multithreaded Java Applications:
Optimizing memory usage in multithreaded Java applications requires a multifaceted approach:
- Object pooling: Reuse objects instead of constantly creating and destroying them. Object pools can significantly reduce the overhead of object creation and garbage collection.
-
Efficient data structures: Choose appropriate data structures based on the access patterns. For example, use
ArrayList
for sequential access andHashMap
for random access. Consider using specialized data structures designed for concurrency, likeConcurrentHashMap
. - Avoid unnecessary object creation: Be mindful of object creation, especially in loops. Reuse objects whenever possible to minimize garbage collection overhead.
-
Proper use of weak references: Use weak references (
WeakReference
) to allow the garbage collector to reclaim objects when memory is low. This is particularly useful for caching. - Tune garbage collection: Experiment with different garbage collection algorithms to find the best balance between throughput and pause times. The choice of garbage collector depends on the application's specific needs.
- Memory profiling: Use memory profiling tools to identify memory leaks and areas for optimization. Tools like JProfiler and YourKit can help pinpoint memory-intensive parts of your application.
- Avoid memory leaks: Carefully manage resources and ensure that objects are properly garbage collected. Pay close attention to long-lived objects that might hold references to other objects, preventing them from being garbage collected.
What are the differences between Java's memory model and other languages' memory models?
Differences Between Java's Memory Model and Other Languages' Memory Models:
Java's memory model differs from those of other languages in several key aspects:
- Explicitness of synchronization: Java's memory model explicitly defines synchronization primitives and their effects on memory visibility. Some languages have weaker memory models where synchronization is less explicit or relies on compiler optimizations.
- Happens-before relationship: The happens-before relationship is a key concept in Java's memory model, providing a clear way to reason about memory ordering and visibility. Other languages may have different mechanisms for defining memory ordering.
- Data races: Java's memory model clearly defines data races and their potential consequences. Other languages might have less rigorous definitions or enforcement of data race prevention.
- Hardware dependence: Java's memory model attempts to abstract away the underlying hardware architecture, providing a more portable and predictable model. Some languages' memory models are more closely tied to specific hardware architectures.
For instance, C and C have weaker memory models than Java, offering less explicit control over memory visibility and requiring more careful management of synchronization by the programmer. Languages like Go offer features like goroutines and channels which abstract away some of the complexities of concurrency, simplifying the development of concurrent programs, albeit with a different approach to memory management compared to Java's JMM. Each language's memory model is tailored to its design philosophy and target use cases, leading to differences in complexity and programmer responsibility for concurrent code correctness.
The above is the detailed content of What are Java's memory models and how do they impact multithreaded applications?. For more information, please follow other related articles on the PHP Chinese website!
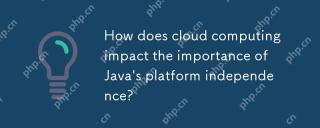
Cloud computing significantly improves Java's platform independence. 1) Java code is compiled into bytecode and executed by the JVM on different operating systems to ensure cross-platform operation. 2) Use Docker and Kubernetes to deploy Java applications to improve portability and scalability.
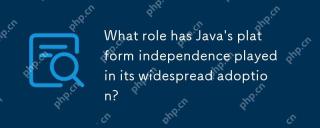
Java'splatformindependenceallowsdeveloperstowritecodeonceandrunitonanydeviceorOSwithaJVM.Thisisachievedthroughcompilingtobytecode,whichtheJVMinterpretsorcompilesatruntime.ThisfeaturehassignificantlyboostedJava'sadoptionduetocross-platformdeployment,s
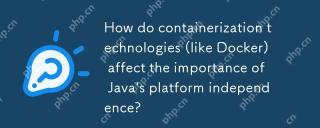
Containerization technologies such as Docker enhance rather than replace Java's platform independence. 1) Ensure consistency across environments, 2) Manage dependencies, including specific JVM versions, 3) Simplify the deployment process to make Java applications more adaptable and manageable.
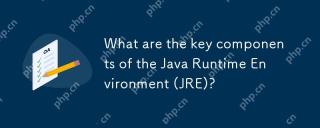
JRE is the environment in which Java applications run, and its function is to enable Java programs to run on different operating systems without recompiling. The working principle of JRE includes JVM executing bytecode, class library provides predefined classes and methods, configuration files and resource files to set up the running environment.
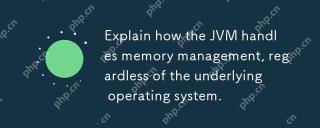
JVM ensures efficient Java programs run through automatic memory management and garbage collection. 1) Memory allocation: Allocate memory in the heap for new objects. 2) Reference count: Track object references and detect garbage. 3) Garbage recycling: Use the tag-clear, tag-tidy or copy algorithm to recycle objects that are no longer referenced.
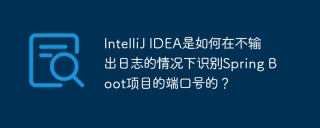
Start Spring using IntelliJIDEAUltimate version...
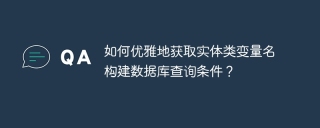
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
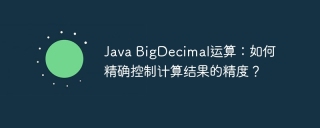
Java...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
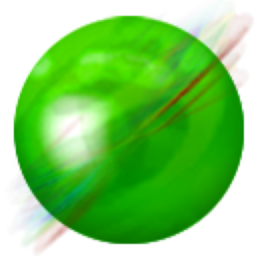
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
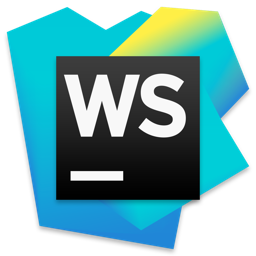
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor