This article details advanced Laravel caching strategies for improved scalability. It emphasizes choosing the right driver (Redis, Memcached), granular cache control with tags and prefixes, effective invalidation methods (tagging, events), and monit
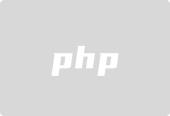
How to Implement Advanced Caching Strategies in Laravel for Better Scalability?
Implementing advanced caching strategies in Laravel involves leveraging its built-in caching system and understanding how to effectively utilize different caching drivers and techniques. The core of Laravel's caching system lies in its Cache
facade, providing a consistent API regardless of the underlying driver. To improve scalability, consider these strategies:
-
Choosing the Right Driver: The optimal driver depends on your application's needs and infrastructure. For high-scalability, Redis or Memcached are generally preferred due to their speed and in-memory nature. However, file-based caching might suffice for smaller applications. Configuration is handled in
config/cache.php
.
-
Granular Cache Control: Instead of caching entire pages, focus on caching specific data fragments. This improves granularity and reduces the impact of cache invalidation. Use tags and prefixes to organize your cached data. For instance, cache user profiles with a
user
prefix and a user ID. This allows you to invalidate only the necessary cache entries.
-
Cache Tagging: Laravel's tagging feature lets you group related cached items. Invalidating a tag invalidates all items associated with it. This is crucial for maintaining data consistency. For example, if you update a product, you can invalidate all cache entries tagged with "products."
-
Cache Key Generation: Use descriptive and consistent key generation methods to avoid collisions. Leverage helper functions or custom methods to generate keys based on the data being cached. This ensures predictable and efficient cache retrieval.
-
Conditional Caching: Employ conditional caching strategies. Before fetching data from the database, check if it's already cached. Only if the cached data is absent or stale, fetch and cache fresh data. This minimizes database load.
-
Using Cache Events: Listen for cache events to perform actions based on cache hits and misses. This provides deeper insight into cache usage and allows you to optimize your strategies.
-
Monitoring Cache Usage: Monitor cache hit and miss rates to identify areas for improvement. Tools like Laravel Telescope can help visualize cache performance and pinpoint inefficiencies.
What are the best caching drivers for Laravel applications needing high scalability?
For Laravel applications demanding high scalability, Redis and Memcached are the top choices.
-
Redis: A highly versatile in-memory data structure store, offering excellent performance and features like pub/sub for real-time updates. It supports various data structures beyond simple key-value pairs, enhancing caching flexibility. Redis is generally considered a strong contender for its robust ecosystem and wide community support.
-
Memcached: A distributed memory object caching system, known for its simplicity and speed. It's particularly efficient for storing smaller data objects. While less feature-rich than Redis, its speed makes it a viable option for applications with intense read operations.
Both Redis and Memcached offer superior performance compared to file-based or database-based caching, especially under heavy load. The choice often comes down to specific needs and infrastructure considerations. Redis's added features might be preferable for more complex scenarios, while Memcached's simplicity can be advantageous for simpler setups.
How can I effectively manage cache invalidation to avoid stale data in a high-traffic Laravel application?
Effective cache invalidation is crucial in high-traffic Laravel applications. Stale data can lead to incorrect information being displayed to users. Here are several strategies:
-
Cache Tagging (Reiterated): This is arguably the most effective method. Group related cache entries under tags. When data changes, invalidate the associated tag, ensuring only relevant cache entries are purged.
-
Event-Driven Invalidation: Trigger cache invalidation based on events. For instance, when a user updates their profile, fire an event that invalidates the corresponding cache entry.
-
Time-to-Live (TTL): Set appropriate TTL values for your cached items. This ensures automatic invalidation after a specified time, minimizing the risk of stale data. However, it's less precise than tag-based invalidation.
-
Cache Interceptors: Use middleware or interceptors to check for cache updates before rendering a response. If data has changed since the last cache update, bypass the cached data.
-
Periodic Purging: For less frequently updated data, consider periodic cache purging. Schedule tasks to clear out old or irrelevant cache entries.
-
Selective Invalidation: Instead of completely invalidating large chunks of data, aim for selective invalidation. Identify the specific parts of the cache that need updating, optimizing performance.
The optimal approach depends on the application's data update frequency and complexity. A combination of techniques, such as tagging and event-driven invalidation, is often the most robust solution.
What are some common performance bottlenecks in Laravel that advanced caching can address?
Advanced caching can significantly alleviate several common performance bottlenecks in Laravel applications:
-
Database Queries: Frequent database queries, especially complex joins or large result sets, are major performance drains. Caching frequently accessed data significantly reduces database load.
-
Slow API Calls: External API calls can be time-consuming. Caching API responses reduces latency and improves response times, particularly for frequently called APIs.
-
Expensive Computations: Complex calculations or data transformations can be costly. Caching the results of these computations avoids redundant calculations.
-
View Rendering: Rendering views, especially those involving numerous database queries or complex logic, can be slow. Caching rendered views, or parts of views, accelerates page load times.
-
Session Management: Session data stored in the database can become a bottleneck. Using a caching mechanism for sessions improves scalability.
By strategically caching data at various levels – database results, API responses, computed values, and rendered views – advanced caching techniques significantly enhance the performance and scalability of Laravel applications. Remember to choose the right caching strategy based on the specific bottleneck and data characteristics.
The above is the detailed content of How to Implement Advanced Caching Strategies in Laravel for Better Scalability?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn