This article explores implementing caching in Yii framework to boost database performance. It details strategies like data fragment, page, query, and object caching, discusses various backend options (Memcached, Redis), and emphasizes effective dep
Implementing Caching in Yii for Database Performance Improvement
Yii provides robust caching capabilities to significantly enhance database performance. The core of Yii's caching mechanism revolves around the Yii::$app->cache
component, which acts as an interface to various backend storage options. Implementing caching involves identifying data frequently accessed from the database and storing it in the cache. When the application needs this data again, it first checks the cache. If the data is found (a "cache hit"), it's retrieved from the cache, bypassing the database query. If not (a "cache miss"), the database is queried, the result is stored in the cache, and then returned to the application.
You can leverage Yii's caching capabilities through its helper methods like Yii::$app->cache->get()
and Yii::$app->cache->set()
. For instance, to cache the result of a database query fetching user details:
$userId = 1; $cacheKey = 'user_' . $userId; $userData = Yii::$app->cache->get($cacheKey); if ($userData === false) { // Cache miss $userData = User::findOne($userId); Yii::$app->cache->set($cacheKey, $userData, 3600); // Store for 1 hour } // Use $userData
This code first checks the cache for data associated with $cacheKey
. If it's not present, it queries the database, stores the result in the cache with a 1-hour expiration time, and then uses the retrieved data. Remember to choose appropriate cache keys that uniquely identify your data.
Best Caching Strategies for High Database Load in Yii
For Yii applications with high database load, employing a multi-layered caching strategy is highly effective. This involves utilizing different caching levels to optimize performance based on data access patterns.
- Data Fragment Caching: Cache frequently accessed individual data elements, such as user profiles, product details, or news items. This is ideal for read-heavy operations where the data changes infrequently. Use appropriate expiration times based on data volatility.
- Page Caching: Cache entire rendered pages. This is particularly beneficial for static or infrequently changing content like landing pages or blog posts. This significantly reduces the load on the database and application logic. However, be cautious about dynamic content and ensure proper invalidation mechanisms.
- Query Caching: Cache the results of complex database queries. Yii's caching mechanism works well with database query results. This can significantly reduce the database load for frequently executed queries. Remember to manage cache invalidation effectively.
- Object Caching: Cache entire model objects. This can be efficient for scenarios where you repeatedly access related data within a model. This requires careful consideration of object lifecycles and potential data inconsistencies.
The optimal strategy depends on your application's specific needs. Profiling your application to identify performance bottlenecks will help determine which caching strategies are most beneficial.
Choosing the Right Caching Backend for Yii
Several caching backends are compatible with Yii, including Memcached, Redis, and APC (although APC is largely deprecated). The best choice depends on your application's requirements and scaling needs.
- Memcached: A high-performance, distributed memory object caching system. It's relatively simple to set up and offers good performance for straightforward caching needs. However, it doesn't support persistent storage, meaning data is lost on server restarts.
- Redis: A more versatile in-memory data structure store. Besides caching, it supports various data structures like lists, sets, and sorted sets, making it suitable for more complex caching scenarios and other functionalities like session management or message queues. Redis also offers persistence options, allowing data to survive server restarts.
- Database Caching (e.g., using a dedicated caching table): While less efficient than dedicated caching solutions, it can be a simple option for smaller applications. However, it adds overhead to your database and might not scale well.
For most Yii applications with significant database load, Redis is generally preferred due to its flexibility, performance, and persistence capabilities. Memcached remains a viable option for simpler applications with less stringent requirements.
Effectively Configuring and Managing Cache Dependencies in Yii
To avoid stale data, effective cache dependency management is crucial. Yii allows you to associate dependencies with cached data. When a dependency changes, the associated cached data is automatically invalidated. This ensures that your application always serves fresh data.
Yii provides several dependency types:
- Tag Dependencies: Assign tags to cached data. Invalidating data with a specific tag invalidates all cached items associated with that tag.
-
Callback Dependencies: Define a callback function that determines whether the cached data is still valid. The callback is executed before retrieving cached data. If the callback returns
false
, the cache is considered invalid. - File Dependencies: Invalidate cached data based on changes to specific files. This is useful for caching data derived from files.
- Database Dependencies: Invalidate cache entries based on changes within database tables. This requires more complex setup but is the most effective method to ensure data consistency.
Properly configuring these dependencies involves associating them with your cached data using Yii::$app->cache->set()
's dependency
parameter. For instance, using a tag dependency:
$dependency = new \yii\caching\TagDependency(['tags' => 'user_profile']); Yii::$app->cache->set($cacheKey, $userData, 3600, $dependency);
This code associates the cached userData
with the user_profile
tag. Invalidating this tag will automatically remove the cached data. Choosing the right dependency type is crucial for maintaining data consistency and avoiding stale data issues. Remember to carefully consider the trade-offs between performance and data freshness when implementing cache dependencies.
The above is the detailed content of How can I implement caching with Yii to improve database performance?. For more information, please follow other related articles on the PHP Chinese website!
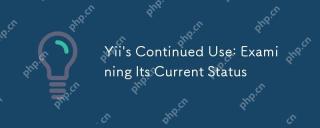
Yii is still competitive in modern development. 1) High performance: adopts lazy loading and caching mechanisms. 2) Security: Built-in CSRF and SQL injection protection. 3) Extensibility: Component-based design is easy to expand and customize.
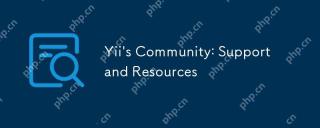
The Yii community provides rich support and resources. 1. Visit the official website and GitHub to get the documentation and code. 2. Use official forums and StackOverflow to solve technical problems. 3. Report bugs and make suggestions through GitHubIssues. 4. Use documents and tutorials to learn the Yii framework.
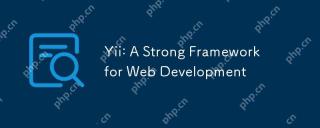
Yii is a high-performance PHP framework designed for fast development and efficient code generation. Its core features include: MVC architecture: Yii adopts MVC architecture to help developers separate application logic and make the code easier to maintain and expand. Componentization and code generation: Through componentization and code generation, Yii reduces the repetitive work of developers and improves development efficiency. Performance Optimization: Yii uses latency loading and caching technologies to ensure efficient operation under high loads and provides powerful ORM capabilities to simplify database operations.
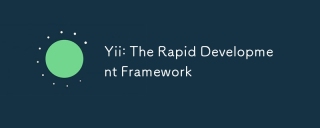
Yii is a high-performance framework based on PHP, suitable for rapid development of web applications. 1) It adopts MVC architecture and component design to simplify the development process. 2) Yii provides rich functions, such as ActiveRecord, RESTfulAPI, etc., which supports high concurrency and expansion. 3) Using Gii tools can quickly generate CRUD code and improve development efficiency. 4) During debugging, you can check configuration files, use debugging tools and view logs. 5) Performance optimization suggestions include using cache, optimizing database queries and maintaining code readability.
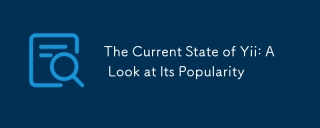
YiiremainspopularbutislessfavoredthanLaravel,withabout14kGitHubstars.ItexcelsinperformanceandActiveRecord,buthasasteeperlearningcurveandasmallerecosystem.It'sidealfordevelopersprioritizingefficiencyoveravastecosystem.
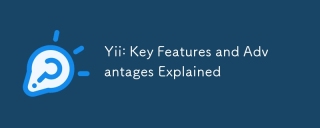
Yii is a high-performance PHP framework that is unique in its componentized architecture, powerful ORM and excellent security. 1. The component-based architecture allows developers to flexibly assemble functions. 2. Powerful ORM simplifies data operation. 3. Built-in multiple security functions to ensure application security.
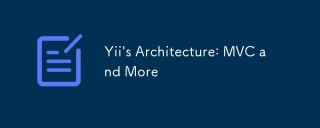
Yii framework adopts an MVC architecture and enhances its flexibility and scalability through components, modules, etc. 1) The MVC mode divides the application logic into model, view and controller. 2) Yii's MVC implementation uses action refinement request processing. 3) Yii supports modular development and improves code organization and management. 4) Use cache and database query optimization to improve performance.
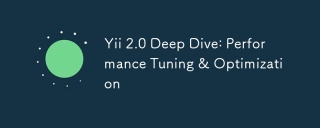
Strategies to improve Yii2.0 application performance include: 1. Database query optimization, using QueryBuilder and ActiveRecord to select specific fields and limit result sets; 2. Caching strategy, rational use of data, query and page cache; 3. Code-level optimization, reducing object creation and using efficient algorithms. Through these methods, the performance of Yii2.0 applications can be significantly improved.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
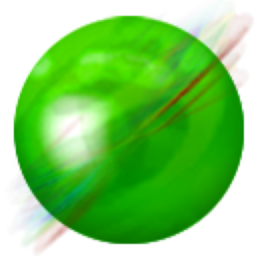
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
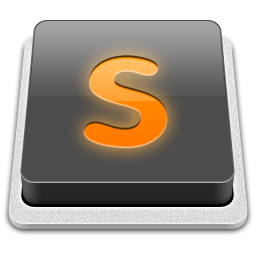
SublimeText3 Mac version
God-level code editing software (SublimeText3)
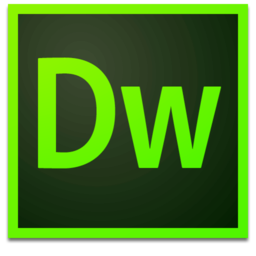
Dreamweaver Mac version
Visual web development tools