


How can I use Workerman's global data feature for shared data between processes?
This article explores Workerman's global data feature for inter-process communication, highlighting its use for sharing data via shared memory. It discusses performance challenges like contention and serialization overhead, emphasizing the need for
How can I use Workerman's global data feature for shared data between processes?
Workerman's global data feature, primarily accessed through Workerman\Worker::$global_data
, allows you to share data across all worker processes within a Workerman application. This is achieved by storing data in a shared memory segment. Any changes made to Workerman\Worker::$global_data
in one worker process will be immediately reflected in other processes. The data is typically serialized using PHP's built-in serialization mechanism.
To use it, simply access and modify the Workerman\Worker::$global_data
array. For example:
// In your worker class class MyWorker extends \Workerman\Worker { public function onWorkerStart() { // Accessing global data $count = isset(self::$global_data['counter']) ? self::$global_data['counter'] : 0; echo "Counter: " . $count . PHP_EOL; // Modifying global data self::$global_data['counter'] ; echo "Counter incremented to: " . self::$global_data['counter'] . PHP_EOL; } } // Initialize the worker $worker = new MyWorker(); $worker->count = 4; // Number of worker processes Workerman\Worker::runAll();
This example shows how to access and increment a counter stored in the global data. Remember that the data type stored within Workerman\Worker::$global_data
must be serializable. Complex objects might require custom serialization and deserialization logic to ensure data integrity.
What are the potential performance implications of using Workerman's global data feature?
Using Workerman's global data feature introduces several potential performance implications:
- Contention: Accessing and modifying shared data creates a critical section. Multiple processes attempting to simultaneously read or write to the same data element will lead to contention, potentially causing performance bottlenecks and slowing down your application. The more processes you have and the more frequently the data is accessed, the more severe this bottleneck becomes.
- Serialization Overhead: Data serialization and deserialization add overhead to every access. While PHP's serialization is relatively efficient, it still consumes processing time. This overhead becomes significant with large or complex data structures.
-
Memory Management: Shared memory is a limited resource. Storing large amounts of data in
Workerman\Worker::$global_data
can lead to memory exhaustion, especially with a large number of worker processes. Improperly managing data within the shared memory can also lead to memory leaks. -
Atomicity Issues: Without proper locking mechanisms, updating complex data structures within
Workerman\Worker::$global_data
might not be atomic. This can lead to data corruption or inconsistencies if multiple processes try to modify the same data concurrently.
How do I ensure data consistency when using Workerman's global data feature across multiple processes?
Ensuring data consistency when using shared memory is crucial. Workerman doesn't provide built-in locking mechanisms for Workerman\Worker::$global_data
. Therefore, you need to implement your own locking mechanism to guarantee atomicity and prevent race conditions. Here are a few strategies:
-
Semaphores: Use system semaphores (e.g.,
sem_acquire
andsem_release
in PHP's PECL semaphore extension) or similar inter-process communication (IPC) mechanisms to protect critical sections of your code that access and modifyWorkerman\Worker::$global_data
. Acquire the semaphore before accessing the data, perform the operation, and then release the semaphore. - File Locking: While less efficient, you can use file locking to synchronize access to the data. This involves creating a lock file and using file locking functions to ensure only one process can access the data at a time.
- Atomic Operations (if applicable): If you're only performing simple atomic operations like incrementing a counter, you might be able to leverage atomic operations provided by the underlying operating system. However, this is highly dependent on the specific operation and the operating system.
Remember to choose a locking mechanism that is appropriate for your application's performance requirements and complexity. Improper locking can lead to deadlocks.
Is there an alternative to Workerman's global data feature for sharing data between processes, and if so, what are its advantages and disadvantages?
Yes, several alternatives exist for sharing data between processes in a Workerman application, offering different trade-offs:
-
Message Queues (e.g., Redis, RabbitMQ): Message queues provide a robust and scalable way to share data asynchronously. Processes communicate by sending and receiving messages, avoiding the complexities of shared memory.
- Advantages: Improved scalability, better fault tolerance, simpler data consistency management.
- Disadvantages: Adds network latency, requires an external message broker, more complex to set up.
-
Shared Database: Using a shared database (e.g., MySQL, PostgreSQL) is another common approach. Processes can read and write data to the database, ensuring data consistency through database transactions.
- Advantages: Data persistence, well-established data consistency mechanisms, mature technology.
- Disadvantages: Database access can be slower than shared memory, introduces database-related complexities.
-
Memcached: Memcached is an in-memory key-value store that can be used for caching frequently accessed data. It offers better performance and scalability than shared memory for data sharing across processes, but doesn't inherently provide the same direct access as
Workerman\Worker::$global_data
.- Advantages: Improved performance and scalability compared to shared memory.
- Disadvantages: Requires an external Memcached server, data is not persistent unless configured for persistence.
The best alternative depends on your application's specific requirements, performance needs, and complexity constraints. For simple applications with low concurrency, Workerman's global data might suffice with careful implementation of locking mechanisms. However, for more complex and scalable applications, message queues or a shared database are generally preferred for better data consistency, fault tolerance, and performance.
The above is the detailed content of How can I use Workerman's global data feature for shared data between processes?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
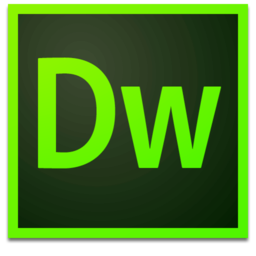
Dreamweaver Mac version
Visual web development tools
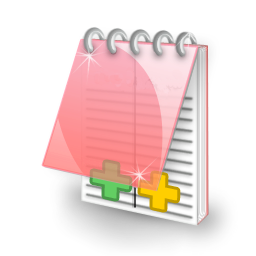
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Atom editor mac version download
The most popular open source editor