What are Best Practices for Memory Management in PHP 8?
Best Practices for Efficient Memory Management in PHP 8
PHP 8, while boasting performance improvements, still requires mindful memory management to avoid performance bottlenecks and crashes. Here are some best practices:
-
Utilize Object-Oriented Programming (OOP): OOP promotes code reusability and maintainability, indirectly contributing to better memory management. Proper object destruction through destructors (
__destruct()
) ensures resources are released when objects are no longer needed. - Avoid Global Variables: Global variables persist throughout the script's lifecycle, consuming memory unnecessarily. Favor local variables and dependency injection for cleaner code and better memory control.
-
Use appropriate data structures: Choose data structures that best suit your needs. For instance, if you need to store a large number of key-value pairs, consider using
SplFixedArray
instead of a standard array if you know the size beforehand, asSplFixedArray
allocates memory upfront and avoids reallocations. -
Efficient String Manipulation: String operations can be memory-intensive. Avoid unnecessary string concatenation in loops. Instead, use functions like
sprintf()
or dedicated string builder classes for more efficient handling. Consider usingmb_substr()
for multibyte string manipulation to avoid unexpected memory issues. -
Proper Resource Handling: Always close database connections, file handles, and other resources using
mysqli_close()
,fclose()
, etc. This explicitly releases the associated memory. Utilize try-catch blocks to ensure resources are released even in case of errors. - Garbage Collection Awareness: While PHP's garbage collector handles most memory cleanup, understanding its cycle helps anticipate potential issues. Avoid creating unnecessary circular references, which can hinder garbage collection.
- Use Generators and Iterators: For large datasets, generators and iterators process data incrementally, reducing memory consumption compared to loading the entire dataset into memory at once.
- Profiling and Monitoring: Regularly profile your application using tools like Xdebug or Blackfire.io to identify memory hotspots and areas for optimization. Monitor memory usage in your production environment to detect potential leaks early on.
-
Employ Memory Limit Settings: Use the
memory_limit
directive in yourphp.ini
file to set a reasonable upper bound for memory usage. This prevents runaway memory consumption from crashing your application. However, set this value appropriately to avoid unnecessary limitations.
How can I identify and resolve memory leaks in my PHP 8 applications?
Identifying and Resolving Memory Leaks
Memory leaks in PHP 8 manifest as steadily increasing memory consumption over time, eventually leading to performance degradation or crashes. Here's how to identify and resolve them:
- Profiling Tools: Use profiling tools like Xdebug or Blackfire.io. These tools provide detailed information on memory allocation, allowing you to pinpoint functions or code sections that are consuming excessive memory. Look for objects that are not being properly released.
- Memory Usage Monitoring: Monitor your application's memory usage in your production environment using tools provided by your hosting provider or system monitoring software. Sudden spikes or a consistent upward trend indicate a potential leak.
-
Circular References: Circular references occur when two or more objects reference each other, preventing the garbage collector from reclaiming their memory. Use tools like
xhprof
to detect these. Refactoring your code to break these circular references is crucial. - Unclosed Resources: Ensure that all database connections, file handles, and other resources are properly closed using their respective closing functions. Use try-catch blocks to handle potential errors and guarantee resource closure.
- Static Variables: Excessive use of static variables can lead to memory leaks, as they persist throughout the script's lifetime. Minimize the use of static variables, and ensure that any resources they hold are released when appropriate.
- Large Data Structures: Avoid loading extremely large datasets into memory all at once. Use iterators or generators to process data in chunks.
-
Debugging Techniques: Use
var_dump()
orprint_r()
strategically to examine the contents of variables and identify unexpectedly large objects that are not being released. Employ logging to track memory usage at critical points in your application.
What are the key differences in memory management between PHP 7 and PHP 8?
Key Differences in Memory Management between PHP 7 and PHP 8
While PHP 8 didn't introduce radical changes to the core memory management system, there are subtle differences and improvements:
- Improved Garbage Collection: PHP 8 generally benefits from refinements in the garbage collection algorithm, leading to potentially more efficient memory reclamation and reduced fragmentation. However, the underlying mechanism remains largely the same.
- JIT Compiler Optimizations: The Just-In-Time (JIT) compiler in PHP 8 can optimize memory usage in specific scenarios by reducing redundant allocations and improving code efficiency. This leads to better overall performance, indirectly impacting memory consumption.
- Union Types and Attributes: While not directly related to memory management itself, the introduction of union types and attributes in PHP 8 contributes to cleaner and more maintainable code. This indirect improvement reduces the likelihood of memory-related errors arising from complex or poorly structured code.
- No Major Architectural Changes: The fundamental memory management mechanisms (reference counting, cyclic garbage collection) remain consistent between PHP 7 and PHP 8. The improvements are primarily incremental optimizations and refinements within the existing framework.
What techniques can improve the performance of my PHP 8 application by optimizing memory usage?
Techniques for Optimizing Memory Usage and Performance in PHP 8
Optimizing memory usage directly translates to performance improvements in PHP 8 applications. Here are some key techniques:
- Caching: Implement caching mechanisms (e.g., opcode caching with Opcache, data caching with Redis or Memcached) to reduce the need to repeatedly generate data or execute expensive operations. This reduces memory pressure by avoiding redundant calculations and data loading.
- Asynchronous Operations: For long-running tasks, consider using asynchronous programming techniques (e.g., using message queues) to avoid blocking the main thread and consuming excessive memory while waiting for results.
- Efficient Database Queries: Optimize database queries to retrieve only the necessary data. Avoid fetching entire tables when only a subset is required. Use appropriate indexing to speed up queries and reduce database interaction overhead.
- Compression: For large datasets, consider compressing data before storing it in memory or on disk. This reduces the memory footprint.
- Data Serialization: Efficiently serialize and deserialize data using formats like JSON or MessagePack, which are more compact than traditional PHP serialization.
- Code Optimization: Refactor your code to eliminate redundant calculations, unnecessary object creations, and inefficient algorithms. Profile your code to identify bottlenecks and optimize accordingly.
- Load Balancing: Distribute the workload across multiple servers to reduce the memory burden on any single machine.
By implementing these best practices and techniques, you can significantly enhance the performance and stability of your PHP 8 applications by effectively managing memory usage. Remember that regular profiling and monitoring are crucial for identifying and addressing memory-related issues proactively.
The above is the detailed content of What are Best Practices for Memory Management in PHP 8?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
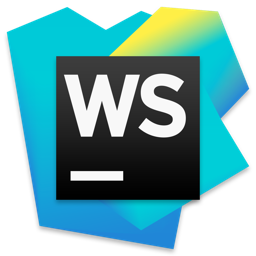
WebStorm Mac version
Useful JavaScript development tools
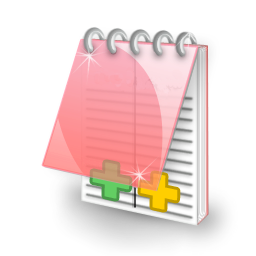
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!