Sorting Custom Data Types with Go's sort
Package
This article addresses common questions regarding the use of Go's sort
package for custom data types. We'll cover sorting custom structs, implementing the Less
function, and best practices for complex data structures.
How can I use Go's sort package for custom data types?
Go's sort
package provides efficient sorting algorithms for slices. However, to sort custom data types, you need to implement the sort.Interface
interface. This interface requires three methods: Len()
, Less(i, j int) bool
, and Swap(i, j int)
. Let's illustrate with an example:
package main import ( "fmt" "sort" ) // Person struct represents a person with a name and age. type Person struct { Name string Age int } // ByAge implements sort.Interface for []Person based on the Age field. type ByAge []Person func (a ByAge) Len() int { return len(a) } func (a ByAge) Swap(i, j int) { a[i], a[j] = a[j], a[i] } func (a ByAge) Less(i, j int) bool { return a[i].Age < a[j].Age } func main() { people := []Person{ {"Alice", 30}, {"Bob", 25}, {"Charlie", 35}, } sort.Sort(ByAge(people)) // Sort the slice of Person structs by age. fmt.Println(people) // Output: [{Bob 25} {Alice 30} {Charlie 35}] }
In this example, ByAge
implements sort.Interface
for a slice of Person
structs. The Less
function compares the ages of two people, defining the sorting order. The sort.Sort
function then uses this interface to sort the slice efficiently. This pattern can be applied to any custom data type. You create a new type that's a slice of your custom type, implement the sort.Interface
methods for that new type, and then use sort.Sort
to sort your slice.
Can I sort structs in Go using the sort package?
Yes, absolutely. As demonstrated in the previous example, you can sort structs using the sort
package. The key is to create a type that satisfies the sort.Interface
and define the Less
function to specify how the structs should be compared (e.g., by a specific field or a combination of fields). The struct fields can be of any comparable type (e.g., int
, string
, float64
). If you need to compare complex fields or use custom comparison logic, you'll need to incorporate that logic within the Less
function.
How do I implement the Less function for custom types in Go's sort package?
The Less(i, j int) bool
function is crucial for defining the sorting order. It takes two indices i
and j
as input, representing elements in the slice. It should return true
if the element at index i
should come before the element at index j
in the sorted order, and false
otherwise. The implementation depends entirely on your sorting criteria.
For instance, if you're sorting Person
structs by age, as shown before: return a[i].Age . If you need a more complex comparison (e.g., sorting by name then by age), you would implement it like this:
func (a ByNameThenAge []Person) Less(i, j int) bool { if a[i].Name != a[j].Name { return a[i].Name < a[j].Name } return a[i].Age < a[j].Age }
This prioritizes name sorting; only if names are equal does it compare ages. Remember, the Less
function must be consistent and reflexive (a.Less(b) && b.Less(c) implies a.Less(c)) to ensure a correctly sorted result.
What are the best practices for using Go's sort package with complex data structures?
When dealing with complex data structures, consider these best practices:
-
Separate Sorting Logic: Keep the sorting logic separate from the data structure itself. Create a custom type that implements
sort.Interface
instead of embedding the sorting methods directly into your main struct. This improves code organization and maintainability. -
Efficient Comparisons: Avoid expensive operations within the
Less
function. Pre-compute values if possible to speed up comparisons. For example, if you're sorting by a calculated field, calculate it once and store it as a separate field. -
Handle Edge Cases: Carefully consider edge cases, such as
nil
values or values that might cause panics during comparison (e.g., comparing strings that might benil
). Add appropriate error handling or checks. -
Testability: Write unit tests to verify the correctness of your
Less
function and the overall sorting behavior. This helps prevent subtle bugs that might be difficult to detect otherwise. -
Consider Alternatives: If your sorting needs are highly specialized or performance-critical, consider using alternative sorting algorithms or libraries that might be more suitable than the standard
sort
package. For very large datasets, consider using techniques like external sorting.
By following these best practices, you can effectively and efficiently utilize Go's sort
package for sorting even the most complex data structures. Remember to always prioritize clear, well-documented code for maintainability and readability.
The above is the detailed content of How can I use Go's sort package for custom data types?. For more information, please follow other related articles on the PHP Chinese website!
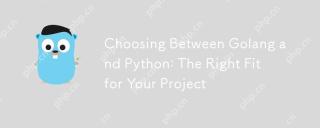
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
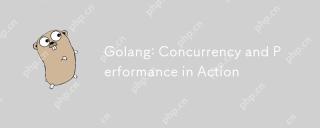
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
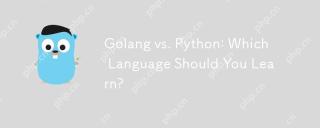
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
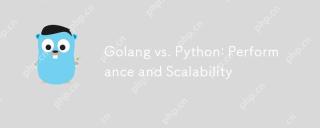
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
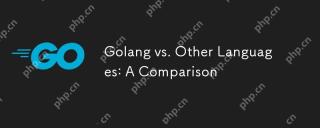
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
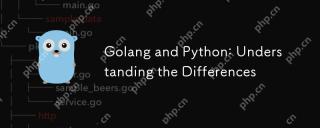
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
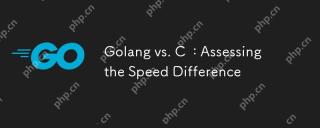
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
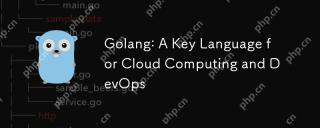
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
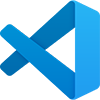
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
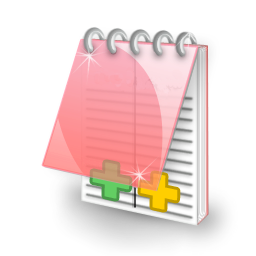
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
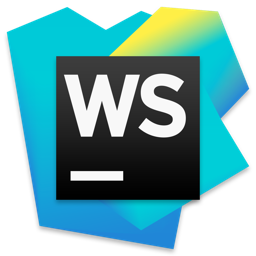
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.