


How do I benchmark and compare different algorithm implementations in Go?
Benchmarking and comparing different algorithm implementations in Go involves using the built-in testing
package's benchmarking capabilities. This allows you to measure the execution time of your algorithms under various conditions and compare their performance. The core process involves writing benchmark functions that are annotated with the Benchmark
prefix. These functions take a benchmarking object (*testing.B
) as an argument, which provides methods for controlling the benchmark's execution, such as running the algorithm multiple times and measuring the total execution time. You then use the go test -bench=.
command to run the benchmarks.
For example, let's say you have two sorting algorithms, bubbleSort
and quickSort
:
package main import ( "fmt" "math/rand" "sort" "testing" ) func bubbleSort(data []int) { n := len(data) for i := 0; i < n-1; i++ { for j := 0; j < n-i-1; j++ { if data[j] > data[j+1] { data[j], data[j+1] = data[j+1], data[j] } } } } func quickSort(data []int) { sort.Ints(data) // Using Go's built-in quick sort for comparison } func BenchmarkBubbleSort(b *testing.B) { data := generateRandomData(1000) for i := 0; i < b.N; i++ { bubbleSort(append([]int(nil), data...)) // Create a copy to avoid modifying the original data } } func BenchmarkQuickSort(b *testing.B) { data := generateRandomData(1000) for i := 0; i < b.N; i++ { quickSort(append([]int(nil), data...)) // Create a copy to avoid modifying the original data } } func generateRandomData(size int) []int { data := make([]int, size) for i := 0; i < size; i++ { data[i] = rand.Intn(size) } return data } func main() {}
Running go test -bench=.
will output the benchmark results, showing the execution time for each algorithm. You can then directly compare these times to assess the relative performance. Remember to run benchmarks multiple times and on different datasets to get a reliable comparison.
What are the best practices for benchmarking algorithm performance in Go?
Several best practices ensure accurate and reliable benchmarking results:
- Use representative data: The data used in your benchmarks should accurately reflect the data your algorithm will encounter in real-world scenarios. Avoid using overly simplistic or contrived data sets.
-
Run multiple times: Execute each benchmark multiple times (controlled by
b.N
) to reduce the impact of random fluctuations and get a more stable average. -
Warm-up: Before measuring the execution time, run the algorithm a few times to allow the JIT compiler to optimize the code. This prevents the first few runs from being artificially slow.
b.ResetTimer()
can be used after a warm-up phase. - Measure only the relevant code: Focus on measuring the execution time of the core algorithm, excluding any extraneous operations like data generation or output.
- Use consistent hardware and software: Run your benchmarks on the same hardware and software configuration to ensure consistent results.
-
Consider memory usage: Besides execution time, also consider the memory usage of your algorithms, particularly for large datasets. Tools like
pprof
can help with this. - Account for data structure choice: The choice of data structure can significantly impact algorithm performance. Ensure fair comparisons by using consistent data structures for all algorithms being compared.
- Test with different input sizes: Benchmark your algorithms with a range of input sizes to understand how their performance scales.
- Properly handle data copying: Avoid unnecessary data copying within the benchmark loop. This can significantly skew results. Use techniques like slicing to create copies efficiently.
How can I effectively visualize the results of my Go algorithm benchmarks for clear comparison?
While the go test -bench=.
command provides numerical results, visualizing these results can greatly improve understanding and comparison. Several approaches can achieve this:
- Spreadsheet software: Export the benchmark results (usually to the console) and import them into a spreadsheet program like Excel or Google Sheets. You can then create charts (bar charts, line graphs) to visually compare the performance of different algorithms across various input sizes.
-
Plotting libraries: Use Go plotting libraries like
gonum/plot
to generate charts directly from your benchmark data within your Go code. This provides more automation and integration with your benchmarking process. -
Benchmarking tools: Some specialized benchmarking tools provide built-in visualization capabilities. Explore tools beyond the standard
go test
command to see if they offer this functionality.
The choice of visualization method depends on your needs and preferences. For simple comparisons, a spreadsheet might suffice. For more complex analyses or automated reporting, a Go plotting library offers greater flexibility.
What tools and libraries are most helpful for benchmarking and comparing algorithms written in Go?
Beyond the standard testing
package, several tools and libraries can enhance your benchmarking workflow:
-
testing
package (Go's built-in): This is the foundation for benchmarking in Go. It provides the necessary functions for defining and running benchmarks. -
go test
command: This command-line tool executes Go tests and benchmarks. Flags like-bench=.
,-benchmem
, and-benchtime
allow fine-grained control over the benchmarking process. -
pprof
(profiling tool): While not directly for benchmarking,pprof
is invaluable for analyzing the performance bottlenecks of your algorithms. It can identify areas where your code spends the most time, allowing for targeted optimization. -
gonum/plot
(plotting library): This library facilitates the creation of charts and graphs to visualize your benchmark results effectively. - Other profiling tools: Consider other profiling tools that might offer more advanced features or better integration with your development environment.
By combining these tools and following best practices, you can effectively benchmark, compare, and visualize the performance of your Go algorithms, leading to more informed decisions about algorithm selection and optimization.
The above is the detailed content of How do I benchmark and compare different algorithm implementations in Go?. For more information, please follow other related articles on the PHP Chinese website!
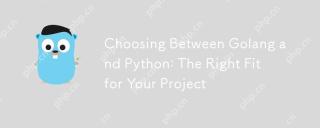
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
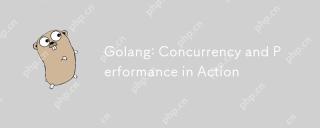
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
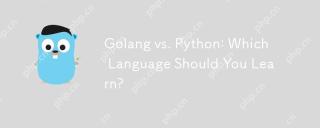
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
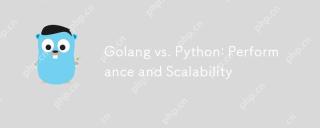
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
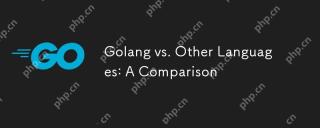
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
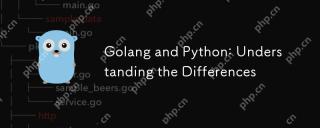
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
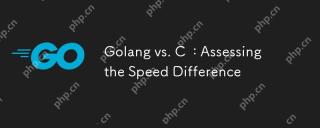
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
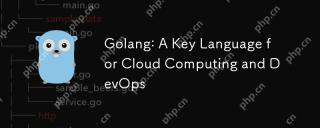
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
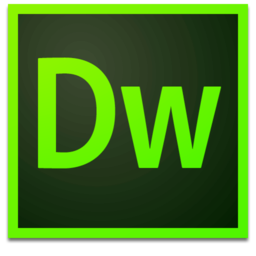
Dreamweaver Mac version
Visual web development tools
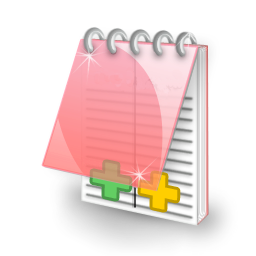
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Atom editor mac version download
The most popular open source editor