Mocking in Unit Tests: Mockito vs. EasyMock vs. JMockit
This article compares three popular Java mocking frameworks: Mockito, EasyMock, and JMockit, focusing on their syntax, features, ease of use, and long-term suitability. We'll address each question in turn.
Understanding the Key Differences in Syntax and Usage
Mockito, EasyMock, and JMockit all allow you to create mock objects that simulate the behavior of real objects during unit testing. However, they differ significantly in their approach and syntax.
-
Mockito: Employs a more natural and intuitive syntax. It uses a "natural" style where you stub methods directly on the mock object using methods like
when()
andthenReturn()
. It supports various types of verification, including verification of method calls withverify()
. Mockito is generally considered easier to learn and use, especially for beginners. Example:
List<String> mockList = Mockito.mock(List.class); Mockito.when(mockList.get(0)).thenReturn("Hello"); String result = mockList.get(0); // result will be "Hello" Mockito.verify(mockList).get(0); // Verifies that get(0) was called
-
EasyMock: Uses a record-and-replay style. You first define the expected interactions (method calls and return values) using methods like
expect()
andandReturn()
. Then, you replay the mock object, and finally, verify that all expected interactions occurred usingverify()
. This approach can be more verbose and less intuitive than Mockito, especially for complex scenarios. Example:
List<String> mockList = EasyMock.createMock(List.class); EasyMock.expect(mockList.get(0)).andReturn("Hello"); EasyMock.replay(mockList); String result = mockList.get(0); // result will be "Hello" EasyMock.verify(mockList);
-
JMockit: Offers a powerful and flexible approach using bytecode manipulation. It allows for mocking classes, interfaces, and even final classes and methods without the need for explicit mock creation in many cases. JMockit uses annotations like
@Mocked
to specify which objects should be mocked. This can lead to cleaner test code but has a steeper learning curve. Example:
@Test public void testSomething(@Mocked List<String> mockList) { mockList.add("Hello"); // This automatically mocks the add method assertEquals(1, mockList.size()); }
Best Balance of Ease of Use and Powerful Features for Complex Scenarios
For complex mocking scenarios, Mockito generally offers the best balance of ease of use and powerful features. While JMockit provides incredibly powerful capabilities, its bytecode manipulation can make debugging and understanding complex interactions more challenging. Mockito's clear syntax and comprehensive features (e.g., argument matchers, spy objects) make it well-suited for managing intricate mocking requirements without sacrificing readability. EasyMock's record-and-replay style becomes increasingly cumbersome as the complexity grows.
Recommended Framework for Long-Term Projects: Maintainability and Community Support
For long-term projects, Mockito is generally recommended due to its larger and more active community, extensive documentation, and readily available support resources. A larger community translates to easier troubleshooting, quicker resolution of issues, and a greater abundance of readily available examples and tutorials. While JMockit is powerful, its smaller community might lead to challenges in finding solutions to specific problems. EasyMock, while mature, has seen a decline in popularity compared to Mockito, resulting in less readily available support. The cleaner syntax and easier-to-understand approach of Mockito also contribute to better maintainability over the long term.
The above is the detailed content of Mocking in Unit Tests: Mockito vs. EasyMock vs. JMockit. For more information, please follow other related articles on the PHP Chinese website!
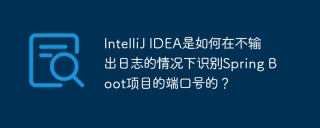
Start Spring using IntelliJIDEAUltimate version...
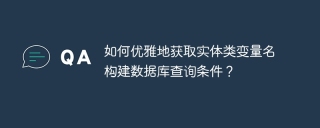
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
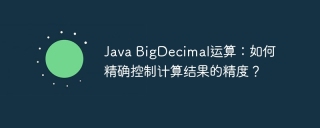
Java...
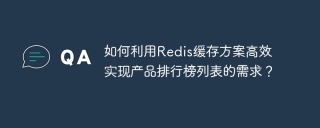
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
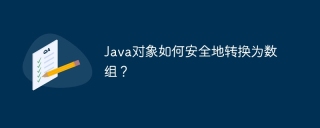
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
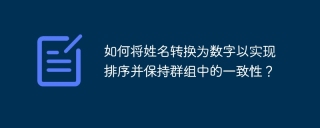
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
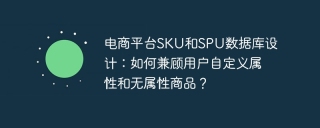
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
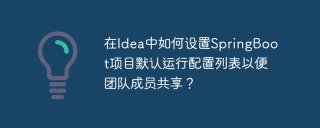
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
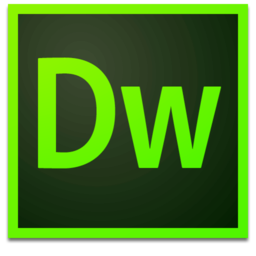
Dreamweaver Mac version
Visual web development tools
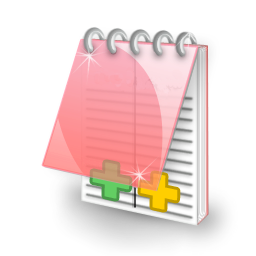
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Atom editor mac version download
The most popular open source editor