Preventing XSS, CSRF, and SQL Injection in JavaScript Applications
This article addresses common web vulnerabilities and how to mitigate them in JavaScript applications. We'll cover Cross-Site Scripting (XSS), Cross-Site Request Forgery (CSRF), and SQL Injection. Effective security requires a layered approach, encompassing both client-side (JavaScript) and server-side measures. While JavaScript can play a role in defense, it's crucial to remember that it's not the sole line of defense; server-side validation is paramount.
How to Effectively Sanitize User Inputs to Prevent XSS Vulnerabilities
XSS attacks occur when malicious scripts are injected into a website and executed in the user's browser. Effective sanitization is crucial to prevent this. Never trust user input. Always validate and sanitize data on both the client-side (JavaScript) and, more importantly, the server-side. Here's a breakdown of techniques:
-
Output Encoding: This is the most effective method. Before displaying user-supplied data on a webpage, encode it according to the context where it will be displayed. For HTML contexts, use
DOMPurify
library or similar robust solutions. This library will escape special characters like, <code>>
,"
,'
, and&
, preventing them from being interpreted as HTML tags or script code. For attributes, use appropriate attribute escaping. For example, if you are embedding user input in asrc
attribute, you'll need to escape special characters differently than if you are embedding it within the text content of an element. - Input Validation: Validate user input on the server-side to ensure it conforms to expected formats and data types. Client-side validation using JavaScript can provide immediate feedback to the user, but it should never be the sole security measure. Server-side validation is essential to prevent malicious users from bypassing client-side checks. Regular expressions can be used to enforce specific patterns.
- Content Security Policy (CSP): Implement a CSP header on your server. This header controls the resources the browser is allowed to load, reducing the risk of XSS attacks by restricting the sources of scripts and other resources. A well-configured CSP can significantly mitigate the impact of successful XSS attacks.
- Use a Templating Engine: Employ a templating engine (e.g., Handlebars, Mustache, etc.) that automatically escapes user input, preventing accidental injection of malicious scripts.
-
Avoid
eval()
andinnerHTML
: These functions are dangerous and should be avoided whenever possible. They can easily lead to XSS vulnerabilities if used with unsanitized user input. Prefer safer methods for manipulating the DOM.
Best Practices for Protecting Against CSRF Attacks When Building JavaScript Applications
CSRF (Cross-Site Request Forgery) attacks trick users into performing unwanted actions on a website they're already authenticated to. These attacks typically involve embedding malicious links or forms on a different website. Effective protection relies on server-side measures primarily, but client-side considerations can enhance security.
- Synchronizer Token Pattern: This is the most common and effective method. The server generates a unique, unpredictable token and includes it in a hidden form field or cookie. The server-side then validates this token with each request. If the token is missing or invalid, the request is rejected. JavaScript can be used to handle the token's inclusion in forms.
-
HTTP Referer Header Check (Weak): While the
Referer
header can provide some indication of the origin of a request, it's unreliable and easily spoofed. It should not be solely relied upon for CSRF protection. -
SameSite Cookies: Setting the
SameSite
attribute on your cookies toStrict
orLax
can prevent cookies from being sent in cross-site requests, making CSRF attacks more difficult. This is a crucial server-side configuration. - HTTPS: Always use HTTPS to encrypt communication between the client and the server. This prevents attackers from intercepting and manipulating requests.
Techniques to Prevent SQL Injection Vulnerabilities in My JavaScript Application's Database Interactions
SQL injection allows attackers to inject malicious SQL code into database queries, potentially compromising data or gaining unauthorized access. Again, the primary defense is on the server-side. JavaScript should never directly construct SQL queries.
- Parameterized Queries (Prepared Statements): This is the gold standard for preventing SQL injection. Instead of directly embedding user input into SQL queries, use parameterized queries. The database driver treats parameters as data, not executable code, preventing injection. Your backend framework should handle this. This is a server-side solution.
- Object-Relational Mappers (ORMs): ORMs provide an abstraction layer between your application code and the database. They often automatically handle parameterized queries, making it easier to avoid SQL injection vulnerabilities.
- Input Validation (Server-Side): Even with parameterized queries, validating user input on the server-side is crucial to ensure data integrity and prevent unexpected behavior.
- Avoid Dynamic SQL: Never construct SQL queries dynamically based on unsanitized user input. Always use parameterized queries or ORMs.
- Least Privilege: Ensure your database user has only the necessary permissions to perform its tasks, minimizing the impact of a successful SQL injection attack. This is a database configuration issue.
Remember that client-side JavaScript security measures are supplementary and should always be combined with robust server-side validation and security practices. Relying solely on client-side protection is extremely risky.
The above is the detailed content of Preventing XSS, CSRF, and SQL Injection in JavaScript Applications. For more information, please follow other related articles on the PHP Chinese website!
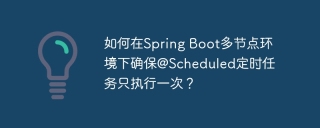
How to avoid repeated execution of timed tasks in SpringBoot multi-node environment? In Spring...
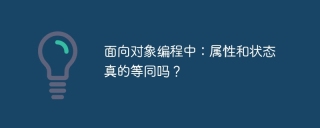
Deeply discussing properties and states in object-oriented programming. In object-oriented programming, the concepts of properties and state are often confused, and there is a subtle between them...
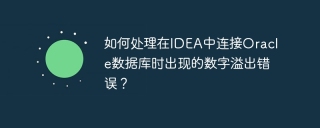
How to deal with digital overflow errors when connecting to Oracle database in IDEA When we are using IntelliJ...
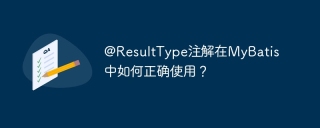
When studying the MyBatis framework, developers often encounter various problems about annotations. One of the common questions is how to use the @ResultType annotation correctly...
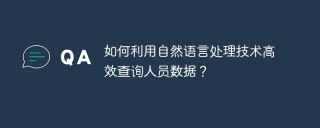
Methods of using natural language processing technology to query personnel data In modern enterprises, the management and query of personnel data is a common requirement. Suppose we...
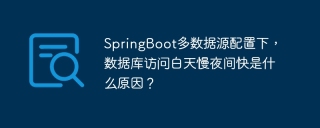
Database access performance problem in Springboot project multi-data source configuration This article aims at using Atomikos for multi-data source configuration in a Springboot project...
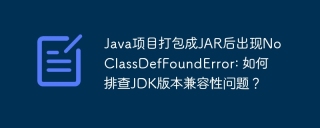
When packaging a Java project into an executable JAR file, it encounters the problem of NoClassDefFoundError. Many Java developers may...
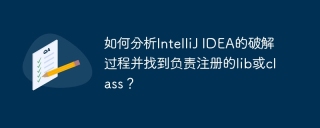
Regarding the analysis method of IntelliJIDEA cracking in the programming world, IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
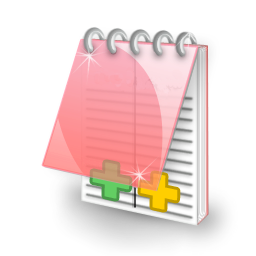
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool