Checking Logs in a Spock Test Example
Spock offers several ways to check logs within your unit tests. The most straightforward approach involves using a mocking framework like Mockito to mock your logging implementation (e.g., org.slf4j.Logger
). This allows you to verify that specific logging methods (like debug()
, info()
, warn()
, error()
) were called with the expected messages and parameters.
Here's an example using Mockito:
import spock.lang.* import static org.mockito.Mockito.* class MyService { private final Logger logger = LoggerFactory.getLogger(MyService.class) void myMethod(String input) { if (input == null) { logger.error("Input is null!") } else { logger.info("Processing input: {}", input) } } } class MyServiceTest extends Specification { def "test log messages"() { given: Logger mockLogger = mock(Logger.class) MyService service = new MyService(mockLogger) // Inject mock logger when: service.myMethod(null) service.myMethod("hello") then: verify(mockLogger).error("Input is null!") verify(mockLogger).info("Processing input: hello") } }
This example demonstrates how to mock the logger and then verify the calls to its methods using Mockito's verify()
method. This approach keeps your tests focused on the behavior of your service and isolates them from the complexities of the logging framework. Remember to include necessary dependencies for Mockito and SLF4j in your build.gradle
or pom.xml
.
How Can I Effectively Assert Log Messages Within My Spock Unit Tests?
Effectively asserting log messages within Spock unit tests requires careful consideration of your logging strategy. While mocking the logger as shown above is often the preferred approach for unit tests, it can be less suitable for integration tests where you want to see the actual log output.
For more direct assertion, you can use a logging appender that captures log messages into a collection (e.g., a list). This allows you to directly assert the contents of the captured messages. Libraries like Logback offer this functionality. You can configure a custom appender to write to an in-memory list, then assert against that list after your test execution. This approach is more suitable for integration testing, where you want to check the actual logging behavior in a closer-to-production environment.
What Are the Best Practices for Handling and Verifying Log Output in Spock Integration Tests?
Best practices for handling and verifying log output in Spock integration tests include:
- Using a dedicated logging appender: As mentioned earlier, employing a custom appender that collects log messages in memory avoids the need to parse log files. This significantly speeds up your tests.
-
Filtering log levels: Configure your appender to capture only the log levels relevant to your tests (e.g., only
ERROR
orWARN
levels for specific test cases). This reduces the amount of data you need to process and improves test readability. - Using clear and concise log messages: Well-structured log messages with meaningful information greatly simplify the process of verifying log output in your tests.
- Avoiding excessive logging in tests: Overly verbose logging can make your tests harder to read and maintain. Focus on logging the essential information relevant to your test assertions.
- Separate logging for tests: Consider using a separate logging configuration for your tests to avoid interfering with your application's production logging. This could involve setting a different logging level or using a different appender for test execution.
Is There a Way to Integrate a Logging Framework with My Spock Tests for Easier Log Analysis?
Yes, you can easily integrate a logging framework like Logback or Log4j with your Spock tests. The key is proper configuration. You can achieve this by:
- Configuring the logging framework programmatically: You can write code to configure the logging framework (e.g., setting the root logger level, adding appenders) within your Spock test setup.
- Using a separate logback.xml (or log4j.properties) file for tests: This allows you to maintain a separate logging configuration specifically for your tests without affecting your application's production logging. You can point your test environment to this configuration file.
-
Using a testing-specific logging level: You might want to set a less restrictive logging level (e.g.,
DEBUG
) for your tests to capture more detailed information during test execution.
By combining these approaches, you can seamlessly integrate your preferred logging framework with your Spock tests, enabling effective log analysis and ensuring that your tests provide comprehensive coverage of your application's logging behavior. Remember to choose the method that best suits your testing environment and complexity.
The above is the detailed content of Check Logs In A Spock Test Example. For more information, please follow other related articles on the PHP Chinese website!
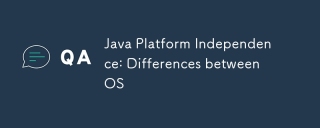
There are subtle differences in Java's performance on different operating systems. 1) The JVM implementations are different, such as HotSpot and OpenJDK, which affect performance and garbage collection. 2) The file system structure and path separator are different, so it needs to be processed using the Java standard library. 3) Differential implementation of network protocols affects network performance. 4) The appearance and behavior of GUI components vary on different systems. By using standard libraries and virtual machine testing, the impact of these differences can be reduced and Java programs can be ensured to run smoothly.
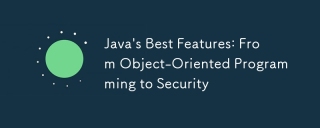
Javaoffersrobustobject-orientedprogramming(OOP)andtop-notchsecurityfeatures.1)OOPinJavaincludesclasses,objects,inheritance,polymorphism,andencapsulation,enablingflexibleandmaintainablesystems.2)SecurityfeaturesincludetheJavaVirtualMachine(JVM)forsand
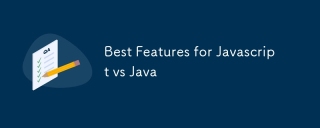
JavaScriptandJavahavedistinctstrengths:JavaScriptexcelsindynamictypingandasynchronousprogramming,whileJavaisrobustwithstrongOOPandtyping.1)JavaScript'sdynamicnatureallowsforrapiddevelopmentandprototyping,withasync/awaitfornon-blockingI/O.2)Java'sOOPf
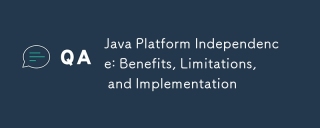
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM)andbytecode.1)TheJVMinterpretsbytecode,allowingthesamecodetorunonanyplatformwithaJVM.2)BytecodeiscompiledfromJavasourcecodeandisplatform-independent.However,limitationsincludepotentialp
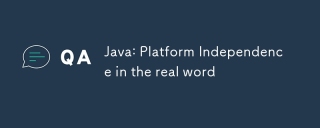
Java'splatformindependencemeansapplicationscanrunonanyplatformwithaJVM,enabling"WriteOnce,RunAnywhere."However,challengesincludeJVMinconsistencies,libraryportability,andperformancevariations.Toaddressthese:1)Usecross-platformtestingtools,2)
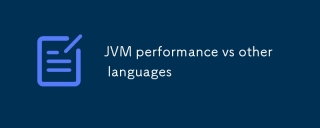
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
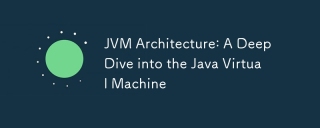
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
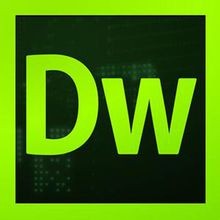
Dreamweaver CS6
Visual web development tools
