Hibernate Envers – Extending Revision Info with Custom Fields
This question explores how to augment the standard revision information tracked by Hibernate Envers with custom fields. Envers, by default, provides information like revision number, timestamp, and the user responsible for the change. However, often you need to store additional context-specific data alongside these standard fields. This is achieved by creating a custom revision entity that extends the default Envers revision entity. This allows you to add your own attributes, providing richer audit trails. We'll explore how to implement this effectively in the following sections.
How can I add custom data to my Hibernate Envers revision information?
To add custom data, you need to create a custom revision entity that extends the default Envers revision entity provided by Hibernate. Let's assume your default revision entity is org.hibernate.envers.DefaultRevisionEntity
. You would create a new entity, for example, CustomRevisionEntity
, which extends DefaultRevisionEntity
(or its equivalent, depending on your Envers version) and adds your custom fields.
@Entity @Audited @Table(name = "REVINFO") public class CustomRevisionEntity extends DefaultRevisionEntity { @Column(name = "application_name") private String applicationName; @Column(name = "client_ip") private String clientIp; // Add other custom fields as needed... // Getters and setters for all fields // ... }
This code defines a CustomRevisionEntity
with two additional fields: applicationName
and clientIp
. Remember to add the necessary getters and setters. The @Entity
, @Audited
, and @Table
annotations are crucial for Hibernate to recognize and manage this entity as an Envers revision entity. The @Table(name = "REVINFO")
annotation assumes your revision information is stored in a table named REVINFO
. Adjust this if your table name differs.
Crucially, you need to configure Hibernate Envers to use your custom revision entity. This is typically done by setting the revisionEntityClass
property in your Hibernate configuration (e.g., hibernate.cfg.xml
or a programmatic configuration).
<property name="org.hibernate.envers.revision_entity_class" value="com.yourpackage.CustomRevisionEntity" />
Replace com.yourpackage.CustomRevisionEntity
with the fully qualified name of your custom revision entity. After this configuration, Envers will use your custom entity to store revision information, including your added custom fields.
What are the best practices for extending Hibernate Envers revision metadata with my own attributes?
Several best practices should be followed when extending Envers revision metadata:
- Keep it relevant: Only add fields that provide genuinely valuable auditing information. Avoid adding unnecessary data to keep the revision table concise and efficient.
- Use appropriate data types: Choose data types that accurately represent the data being stored. Consider using enums for controlled vocabularies and appropriate numeric types for quantities.
- Consider indexing: For frequently queried custom fields, add database indexes to improve query performance. This is especially important for large audit tables.
- Data validation: Implement data validation for your custom fields to maintain data integrity. This could involve using Hibernate validators or custom validation logic.
- Maintainability: Design your custom fields with future extensibility in mind. Avoid hardcoding values or tightly coupling them to specific application logic.
- Naming conventions: Use clear and consistent naming conventions for your custom fields, following your project's style guide. This improves readability and maintainability.
- Performance considerations: Large or complex custom fields might negatively impact performance. Consider storing large objects separately and referencing them in your revision entity using a foreign key relationship.
Is it possible to use custom data types when extending revision info in Hibernate Envers?
Yes, it's entirely possible to use custom data types when extending revision information in Hibernate Envers. Your custom fields in the CustomRevisionEntity
can be of any type supported by Hibernate, including your own custom classes. However, remember that Hibernate needs to be able to persist these types. This means your custom types need to be properly mapped as Hibernate entities or value objects.
For example, if you have a custom Address
class:
@Entity @Audited @Table(name = "REVINFO") public class CustomRevisionEntity extends DefaultRevisionEntity { @Column(name = "application_name") private String applicationName; @Column(name = "client_ip") private String clientIp; // Add other custom fields as needed... // Getters and setters for all fields // ... }
You can include an Address
field in your CustomRevisionEntity
:
<property name="org.hibernate.envers.revision_entity_class" value="com.yourpackage.CustomRevisionEntity" />
Remember that you'll need to ensure that the Address
class is correctly mapped as an embeddable entity using @Embeddable
annotation. This allows Hibernate to persist the address data within the CustomRevisionEntity
. For more complex types, consider using a separate table and a foreign key relationship for optimal database performance. This approach is particularly beneficial for larger or more complex custom data types.
The above is the detailed content of Hibernate Envers – Extending Revision Info with Custom Fields. For more information, please follow other related articles on the PHP Chinese website!
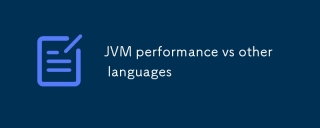
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
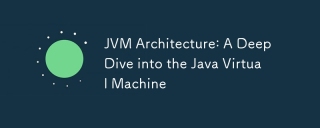
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
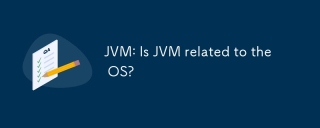
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
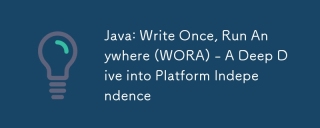
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
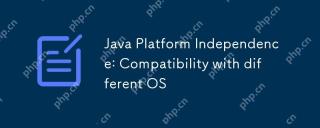
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
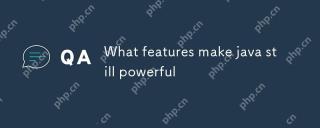
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
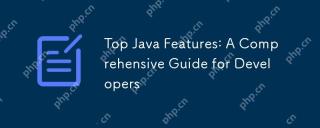
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
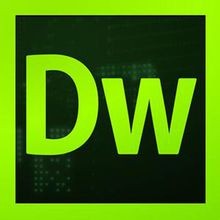
Dreamweaver CS6
Visual web development tools
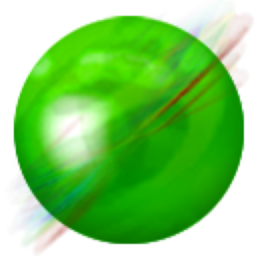
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
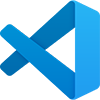
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
